Please only do part b which is below part A. It is only creating a class for starship. I put part A down incase if it's confusing. In python. Part A: In this assignment, we’ll be creating several different classes to represent the crew of a spaceship as they encounter another hostile ship. We’ll start with a Crew class to represent a basic member of the spaceship crew - we’ll later use inheritance on this class to represent a variety of potential specializations that a crew member could have. The Crew class should have the following methods to start: Constructor: The Crew class constructor should have the following signature: __init__(self, name) Where name (the name of the crew member) is a string. The constructor should create an instance variable to store the value of the argument (self.name), along with two more instance variables: self.status, a string representing whether the crew member is currently Active or Injured. This should be initialized to 'Active'. self.location, a string representing where the crew member is currently located on the spaceship. This should be initialized to 'Sleep Pods'. __repr__(self) You must override the repr() method (similar to the str() method, except that it also changes what is output when you pass the object directly to the interpreter) so that it returns a string of the format below. : , at Be sure to match the spacing and format of the above string exactly or you will fail test cases and lose points (note that the angled brackets are not part of the string itself, but indicate where you should substitute in the actual title, name, status, and location of your the crew member). Note that changing __repr__ automatically changes the behavior of str() as well. See the sample output below for an example. Examples: Copy the following if __name__ == "__main__" block into your hw12.py file, and comment out tests for parts of the class you haven’t implemented yet. The lines that have output include the expected value next to them as a comment. if __name__ == '__main__': crew0 = Crew('Ava') print(crew0.name) #Ava print(crew0.status) #Active print(crew0.location) #Sleep Pods print(crew0) #Ava : Active, at Sleep Pods Problem B. Starship Class(please do this part) Before we start looking at some of the more interesting methods for Crew and its subclasses, we need a class to represent the spaceship that the crew members are interacting with. For now, we’ll just set up the constructor for the Starship class. Constructor: The Starship class constructor should have the following signature: __init__(self, name, crew_list) Where name (the name of the ship) is a string, and crew_list is a list of the Crew objects (or its subclasses) that make up the crew of the ship. The constructor should create instance variables to store the value of the arguments (self.name and self.crew_list respectively), along with one more instance variable: self.damaged, a dictionary with string keys and boolean values representing whether each area of the ship is currently damaged. This should be initialized to: {'Bridge':False, 'Medbay':False, 'Engine':False, 'Lasers':False, 'Sleep Pods':False} Examples: Copy the following if __name__ == "__main__" block into your hw12.py file, and comment out tests for parts of the class you haven’t implemented yet. The lines that have output include the expected value next to them as a comment: if __name__ == '__main__': crew1 = Crew('Sakshi') crew2 = Crew('Jina') crew3 = Crew('Daniel') space_boat = Starship('Ebon Hawk', [crew1, crew2, crew3]) print(space_boat.name) #Ebon Hawk print(space_boat.crew_list) #[Sakshi : Active, at Sleep Pods, Jina : Active, at Sleep Pods, Daniel : Active, at Sleep Pods] print(space_boat.damaged) #{'Bridge': False, 'Medbay': False, 'Engine': False, 'Lasers': False, 'Sleep Pods': False}
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Please only do part b which is below part A. It is only creating a class for starship. I put part A down incase if it's confusing. In python.
Part A: In this assignment, we’ll be creating several different classes to represent the crew of a spaceship as they encounter another hostile ship.
We’ll start with a Crew class to represent a basic member of the spaceship crew - we’ll later use inheritance on this class to represent a variety of potential specializations that a crew member could have.
The Crew class should have the following methods to start:
- Constructor: The Crew class constructor should have the following signature:
__init__(self, name)
Where name (the name of the crew member) is a string. The constructor should create an instance variable to store the value of the argument (self.name), along with two more instance variables:
- self.status, a string representing whether the crew member is currently Active or Injured. This should be initialized to 'Active'.
- self.location, a string representing where the crew member is currently located on the spaceship. This should be initialized to 'Sleep Pods'.
- __repr__(self)
You must override the repr() method (similar to the str() method, except that it also changes what is output when you pass the object directly to the interpreter) so that it returns a string of the format below.
<Name> : <Status>, at <Location>
Be sure to match the spacing and format of the above string exactly or you will fail test cases and lose points (note that the angled brackets are not part of the string itself, but indicate where you should substitute in the actual title, name, status, and location of your the crew member). Note that changing __repr__ automatically changes the behavior of str() as well. See the sample output below for an example.
Examples:
Copy the following if __name__ == "__main__" block into your hw12.py file, and comment out tests for parts of the class you haven’t implemented yet. The lines that have output include the expected value next to them as a comment.
if __name__ == '__main__':
crew0 = Crew('Ava')
print(crew0.name) #Ava
print(crew0.status) #Active
print(crew0.location) #Sleep Pods
print(crew0) #Ava : Active, at Sleep Pods
Problem B. Starship Class(please do this part)
Before we start looking at some of the more interesting methods for Crew and its subclasses, we need a class to represent the spaceship that the crew members are interacting with. For now, we’ll just set up the constructor for the Starship class.
- Constructor: The Starship class constructor should have the following signature:
__init__(self, name, crew_list)
Where name (the name of the ship) is a string, and crew_list is a list of the Crew objects (or its subclasses) that make up the crew of the ship. The constructor should create instance variables to store the value of the arguments (self.name and self.crew_list respectively), along with one more instance variable:
- self.damaged, a dictionary with string keys and boolean values representing whether each area of the ship is currently damaged. This should be initialized to:
{'Bridge':False, 'Medbay':False, 'Engine':False,
'Lasers':False, 'Sleep Pods':False}
Examples:
Copy the following if __name__ == "__main__" block into your hw12.py file, and comment out tests for parts of the class you haven’t implemented yet. The lines that have output include the expected value next to them as a comment:
if __name__ == '__main__':
crew1 = Crew('Sakshi')
crew2 = Crew('Jina')
crew3 = Crew('Daniel')
space_boat = Starship('Ebon Hawk', [crew1, crew2, crew3])
print(space_boat.name) #Ebon Hawk
print(space_boat.crew_list) #[Sakshi : Active, at Sleep Pods, Jina : Active, at Sleep Pods, Daniel : Active, at Sleep Pods]
print(space_boat.damaged) #{'Bridge': False, 'Medbay': False, 'Engine': False, 'Lasers': False, 'Sleep Pods': False}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

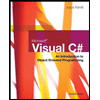
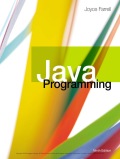
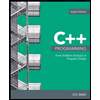
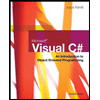
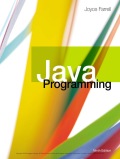
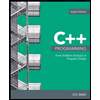