Please read the question to the very end. Below is an example of a code that we need to reprogram in C++ #include const char* filename = "Humans.dat"; struct Human { char name[20]; int phone; }; void my_scanf(Human *s, unsigned short int n) { printf("Enter name %hu >", n+1); scanf("%25s", s->name); printf("Enter phone >"); scanf("%i", &s->phone); } void my_printf(Human *s, unsigned short int n) { for (unsigned short int i = 0; i < n; i++) printf("%i. Name: %s, phone: %i\n", i+1, s[i].name, s[i].phone); } void read_from_user_and_save_to_file() { unsigned short int m; printf("Enter count of Humans >"); scanf("%hu", &m); Human* AllHumans = new Human[m]; for (unsigned short int i = 0; i < m; i++) my_scanf(&AllHumans[i], i); my_printf(AllHumans, m); FILE * fp = fopen(filename, "wb"); fwrite(AllHumans, sizeof(Human), m, fp); fclose(fp); } void read_from_file_and_show_to_user() { FILE * fp = fopen(filename, "rb"); fseek(fp, 0, SEEK_END); unsigned int fsize = ftell(fp); unsigned short int m = fsize / sizeof(Human); Human* AllHumans = new Human[m]; fseek(fp, 0, SEEK_SET); fread(AllHumans, sizeof(Human), m, fp); fclose(fp); my_printf(AllHumans, m); } int main() { read_from_user_and_save_to_file(); read_from_file_and_show_to_user(); } Q. Do you agree, that sequence read_from_user_and_save_to_file(); read_from_file_and_show_to_user(); is good only for initial entering of data? Please check, is code ready to run in other sequence: read_from_file(); show_to_user(); edit_data(); // add, change, delete elements in array save_to_file(); What needs to be changed here? Please, remake the code
Please read the question to the very end.
Below is an example of a code that we need to reprogram in C++
#include <iostream>
const char* filename = "Humans.dat";
struct Human {
char name[20];
int phone;
};
void my_scanf(Human *s, unsigned short int n) {
printf("Enter name %hu >", n+1); scanf("%25s", s->name);
printf("Enter phone >"); scanf("%i", &s->phone);
}
void my_printf(Human *s, unsigned short int n) {
for (unsigned short int i = 0; i < n; i++)
printf("%i. Name: %s, phone: %i\n", i+1, s[i].name, s[i].phone);
}
void read_from_user_and_save_to_file() {
unsigned short int m; printf("Enter count of Humans >"); scanf("%hu", &m);
Human* AllHumans = new Human[m];
for (unsigned short int i = 0; i < m; i++) my_scanf(&AllHumans[i], i);
my_printf(AllHumans, m);
FILE * fp = fopen(filename, "wb");
fwrite(AllHumans, sizeof(Human), m, fp); fclose(fp);
}
void read_from_file_and_show_to_user() {
FILE * fp = fopen(filename, "rb");
fseek(fp, 0, SEEK_END);
unsigned int fsize = ftell(fp);
unsigned short int m = fsize / sizeof(Human);
Human* AllHumans = new Human[m];
fseek(fp, 0, SEEK_SET);
fread(AllHumans, sizeof(Human), m, fp); fclose(fp);
my_printf(AllHumans, m);
}
int main() {
read_from_user_and_save_to_file();
read_from_file_and_show_to_user();
}
Q. Do you agree, that sequence
read_from_user_and_save_to_file();
read_from_file_and_show_to_user();
is good only for initial entering of data?
Please check, is code ready to run in other sequence:
read_from_file();
show_to_user();
edit_data(); // add, change, delete elements in array
save_to_file();
What needs to be changed here? Please, remake the code.

Step by step
Solved in 2 steps

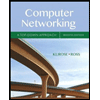
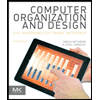
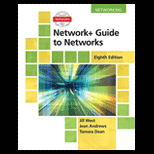
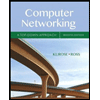
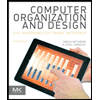
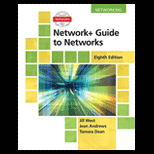
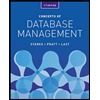
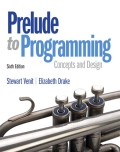
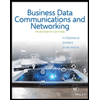