Please read the question very carefully. Before you start to code please draw and analyze the scenarios. Complete the method updateList(head, number) where a non-dummy headed singly linked list and an integer value can be taken as parameters. You have to write a python/JAVA program that will find out the result of mod operation between the given integer and number of nodes of the linked list and then if the result is an odd number then remove the node from that index otherwise insert a new node on that index containing the given integer as value. Note that you are not allowed to use/create a separate linked list. You cannot use any additional methods other than countNode() and nodeAt(). Return head at the end. Return head at the end. The Node class is given below. [Note: If a valid index is found from the result of mod operation for the given linked list, only then insertion/removal can be done on that particular index.]
Please read the question very carefully. Before you start to code please draw and analyze the scenarios. Complete the method updateList(head, number) where a non-dummy headed singly linked list and an integer value can be taken as parameters. You have to write a python/JAVA program that will find out the result of mod operation between the given integer and number of nodes of the linked list and then if the result is an odd number then remove the node from that index otherwise insert a new node on that index containing the given integer as value. Note that you are not allowed to use/create a separate linked list. You cannot use any additional methods other than countNode() and nodeAt(). Return head at the end. Return head at the end. The Node class is given below. [Note: If a valid index is found from the result of mod operation for the given linked list, only then insertion/removal can be done on that particular index.]
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
100%
use python language to solve this question
![Question 2
Please read the question very carefully. Before you start to code please draw and analyze the
scenarios.
Complete the method updateList(head, number) where a non-dummy headed singly linked list
and an integer value can be taken as parameters. You have to write a python/JAVA program that
will find out the result of mod operation between the given integer and number of nodes of the
linked list and then if the result is an odd number then remove the node from that index otherwise
insert a new node on that index containing the given integer as value. Note that you are not allowed
to use/create a separate linked list. You cannot use any additional methods other than countNode()
and nodeAt(). Return head at the end. Return head at the end. The Node class is given below.
[Note: If a valid index is found from the result of mod operation for the given linked list, only then
insertion/removal can be done on that particular index.]
Sample Input
Sample Output Explanation
11 5 4 13 2
Here, the number of nodes of the linked list is 6 and the given
integer is 133. The result of 133 % 6 is 1 which is an odd number
and represents a valid index for insertion/removal. Therefore, the
node at index 1 has been removed.
1195 4 13 2;
133
13 59 17 8; 87 13 5 87 9 17 8 Here, the number of nodes of the linked list is 5 and the given
integer is 87. The result of 87 % 5 is 2 which is an even number and
represents a valid index for insertion/removal. Therefore, a new
node containing 87 as value has been inserted at index 2.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fafc370c2-0278-4830-8365-c4b2fd589557%2F49386ba0-f622-4edd-b0b0-a557fc5e45da%2Fppnjjo7_processed.png&w=3840&q=75)
Transcribed Image Text:Question 2
Please read the question very carefully. Before you start to code please draw and analyze the
scenarios.
Complete the method updateList(head, number) where a non-dummy headed singly linked list
and an integer value can be taken as parameters. You have to write a python/JAVA program that
will find out the result of mod operation between the given integer and number of nodes of the
linked list and then if the result is an odd number then remove the node from that index otherwise
insert a new node on that index containing the given integer as value. Note that you are not allowed
to use/create a separate linked list. You cannot use any additional methods other than countNode()
and nodeAt(). Return head at the end. Return head at the end. The Node class is given below.
[Note: If a valid index is found from the result of mod operation for the given linked list, only then
insertion/removal can be done on that particular index.]
Sample Input
Sample Output Explanation
11 5 4 13 2
Here, the number of nodes of the linked list is 6 and the given
integer is 133. The result of 133 % 6 is 1 which is an odd number
and represents a valid index for insertion/removal. Therefore, the
node at index 1 has been removed.
1195 4 13 2;
133
13 59 17 8; 87 13 5 87 9 17 8 Here, the number of nodes of the linked list is 5 and the given
integer is 87. The result of 87 % 5 is 2 which is an even number and
represents a valid index for insertion/removal. Therefore, a new
node containing 87 as value has been inserted at index 2.
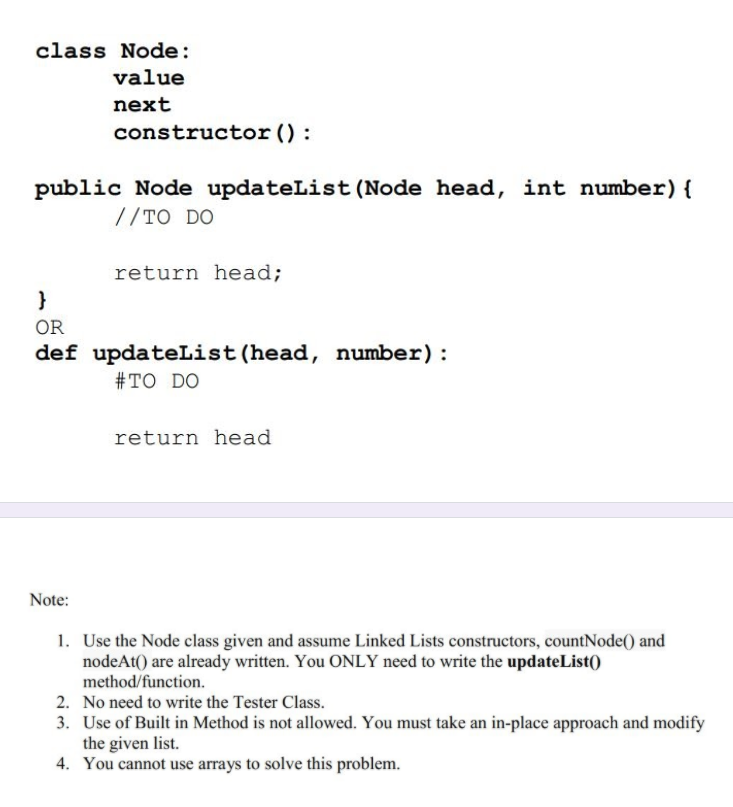
Transcribed Image Text:class Node:
value
next
constructor () :
public Node updateList (Node head, int number){
//TO DO
return head;
}
OR
def updateList (head, number) :
#TO DO
return head
Note:
1. Use the Node class given and assume Linked Lists constructors, countNode() and
nodeAt() are already written. You ONLY need to write the updateList()
method/function.
2. No need to write the Tester Class.
3. Use of Built in Method is not allowed. You must take an in-place approach and modify
the given list.
4. You cannot use arrays to solve this problem.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
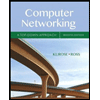
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
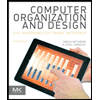
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
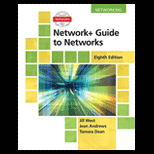
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
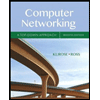
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
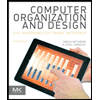
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
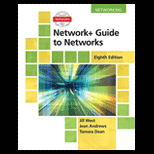
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
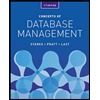
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
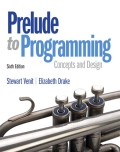
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
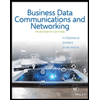
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY