PLEASE RETURN THE ENTIRE CODE, INCLUDING WHAT IS GIVEN (PYTHON) A simple software system for a library models a library as a collection of books and patrons, as per the requirements below: - A patron can have at most three books out on loan at any given time. - Each book has a title, an author, a patron to whom it has been checked out, and a list of patrons waiting for that book to be returned. - When a patron wants to borrow a book, that patron is automatically added to the book’s wait list if the book is already checked out. - When a patron returns a book, it is automatically loaned to the first patron on its wait list who can check out a book. - Each patron has a name and the number of books that patron has currently checked out. Develop the classes Book and Patron to model these objects. Think first of the interface or set of methods to be used with each class, and then choose appropriate data structures for the state of the objects. Here is the code so far, please write the rest of the program based on the above guidelines: class Patron(object): """This class represents a patron with a name and a number of books checked out.""" MAX_BOOKS_OUT = 3 def __init__(self, name): self._name = name self._numBooksOut = 0 def __str__(self): result = self._name + ', ' + str(self._numBooksOut) + \ " books out" return result def getNumBooksOut(self): """Returns the number of books out.""" def inc(self): """Increments the number of books out.""" def dec(self): """Decrements the number of books out.""" class Book(object): """This class represents a book with a title, author, a patron to whom the book is check out, and a wait list of patrons for it.""" def __init__(self, title, author): """Creates a new book with the given title and author.""" self._title = title self._author = author self._patron = None self._waitList = [] def __str__(self): result = 'Title: ' + self._title + '\n' result += 'Author: ' + self._author + '\n' if self._patron: result += "Checked out to: " + str(self._patron) + '\n' else: result += "Not checked out\n" result += "Wait list:\n" for patron in self._waitList: result += str(patron) + '\n' return result def borrowMe(self, patron): """Attempts to loan book to patron.""" def returnMe(self): """Current patron returns book, attempts to loan it to a qualified waiting patron.""" def main(): """Tests the Patron and Book classes.""" p1 = Patron("Ken") p2 = Patron("Martin") b1 = Book("Atonement", "McEwan") b2 = Book("The March", "Doctorow") b3 = Book("Beach Music", "Conroy") b4 = Book("Thirteen Moons", "Frazier") print(b1.borrowMe(p1)) print(b2.borrowMe(p1)) print(b3.borrowMe(p1)) print(b1.borrowMe(p2)) print(b4.borrowMe(p1)) print(p1) print(b1) print(b4) print(b1.returnMe()) print(b2.returnMe()) print(b1) print(b2) main()
PLEASE RETURN THE ENTIRE CODE, INCLUDING WHAT IS GIVEN (PYTHON)
A simple software system for a library models a library as a collection of books and patrons, as per the requirements below:
- A patron can have at most three books out on loan at any given time.
- Each book has a title, an author, a patron to whom it has been checked out, and a list of patrons waiting for that book to be returned.
- When a patron wants to borrow a book, that patron is automatically added to the book’s wait list if the book is already checked out.
- When a patron returns a book, it is automatically loaned to the first patron on its wait list who can check out a book.
- Each patron has a name and the number of books that patron has currently checked out.
Develop the classes Book and Patron to model these objects. Think first of the interface or set of methods to be used with each class, and then choose appropriate data structures for the state of the objects.
Here is the code so far, please write the rest of the program based on the above guidelines:
class Patron(object):
"""This class represents a patron
with a name and a number of books checked out."""
MAX_BOOKS_OUT = 3
def __init__(self, name):
self._name = name
self._numBooksOut = 0
def __str__(self):
result = self._name + ', ' + str(self._numBooksOut) + \
" books out"
return result
def getNumBooksOut(self):
"""Returns the number of books out."""
def inc(self):
"""Increments the number of books out."""
def dec(self):
"""Decrements the number of books out."""
class Book(object):
"""This class represents a book with a title, author,
a patron to whom the book is check out, and a wait list
of patrons for it."""
def __init__(self, title, author):
"""Creates a new book with the given title and author."""
self._title = title
self._author = author
self._patron = None
self._waitList = []
def __str__(self):
result = 'Title: ' + self._title + '\n'
result += 'Author: ' + self._author + '\n'
if self._patron:
result += "Checked out to: " + str(self._patron) + '\n'
else:
result += "Not checked out\n"
result += "Wait list:\n"
for patron in self._waitList:
result += str(patron) + '\n'
return result
def borrowMe(self, patron):
"""Attempts to loan book to patron."""
def returnMe(self):
"""Current patron returns book, attempts to loan it
to a qualified waiting patron."""
def main():
"""Tests the Patron and Book classes."""
p1 = Patron("Ken")
p2 = Patron("Martin")
b1 = Book("Atonement", "McEwan")
b2 = Book("The March", "Doctorow")
b3 = Book("Beach Music", "Conroy")
b4 = Book("Thirteen Moons", "Frazier")
print(b1.borrowMe(p1))
print(b2.borrowMe(p1))
print(b3.borrowMe(p1))
print(b1.borrowMe(p2))
print(b4.borrowMe(p1))
print(p1)
print(b1)
print(b4)
print(b1.returnMe())
print(b2.returnMe())
print(b1)
print(b2)
main()

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

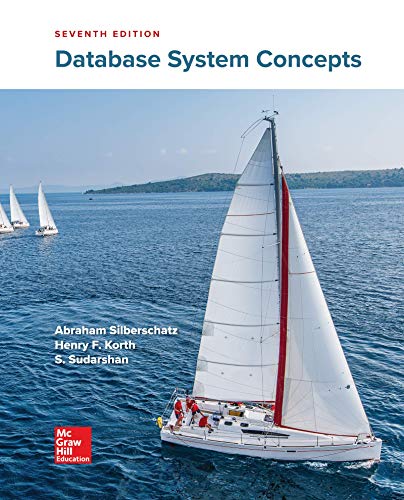
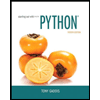
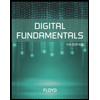
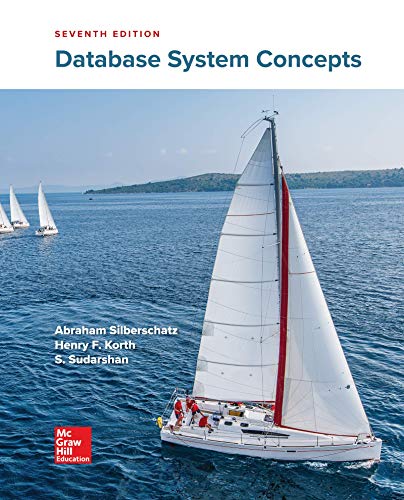
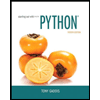
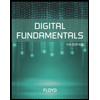
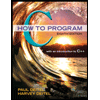
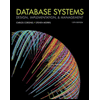
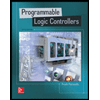