Please see and run attached code: test.py. You need to convert test.py into new code using: function, OOP and exception handling. The code should as the user to key-in the size of playing grid (e.g., 3x3, 6x6, ..etc). For example current grid and final should be printed using function. In addition, invali input should be handled. You need to develop three main classes: Board Player, GameState. Each class must be implemented and tested. It is ex pected that your program can accept various grid sizes, so all input should b set automatically based on the gird size. You must use the same names fo classes (Board, Player, GameState) and functions. The main steps are: 1. Once you run the program, the user will be prompted with a menu. 2. The user will need to enter their choice. 3. The choice will be to either begin the game, or quit. 4. If the user enters an invalid choice, the user should be prompted appro priately letting them choose once more. 5. The program should not end unless the user chooses otherwise.
Test.py
## test
# 3 * 3 grid : board
grid = [' *','* ','*','*','*','*','*','*','*']
# we have two players denomted as "X" and "O"
play=True
count=0
while play:
# add first player
add_x=True
# loop to add player
while add_x:
# at which postion you would like to add the player
player_postion_x=int(input ("Enter postion for player X: "))
## add X player into the board
if grid[player_postion_x-1]!="X" and grid[player_postion_x-1]!="O":
grid[player_postion_x-1]="X"
add_x=False
#to count the number of added players
count=count+1
else:
print ("The postion has been taken")
if grid[0]=="X" and grid[1]=="X" and grid[2]=="X":
print ("Player X win")
print(grid[0:3])
print(grid[3:6])
print(grid[6:9])
break
elif grid[3]=="X" and grid[4]=="X" and grid[5]=="X":
print ("Player X win")
print(grid[0:3])
print(grid[3:6])
print(grid[6:9])
break
elif grid[6]=="X" and grid[7]=="X" and grid[7]=="X":
print ("Player X win")
print(grid[0:3])
print(grid[3:6])
print(grid[6:9])
break
elif grid[0]=="X" and grid[3]=="X" and grid[6]=="X":
print ("Player X win")
print(grid[0:3])
print(grid[3:6])
print(grid[6:9])
break
elif grid[1]=="X" and grid[4]=="X" and grid[7]=="X":
print ("Player X win")
print(grid[0:3])
print(grid[3:6])
print(grid[6:9])
break
elif grid[2]=="X" and grid[5]=="X" and grid[8]=="X":
print ("Player X win")
print(grid[0:3])
print(grid[3:6])
print(grid[6:9])
break
elif grid[0]=="X" and grid[4]=="X" and grid[8]=="X":
print ("Player X win")
print(grid[0:3])
print(grid[3:6])
print(grid[6:9])
break
elif grid[2]=="X" and grid[4]=="X" and grid[6]=="X":
print ("Player X win")
print(grid[0:3])
print(grid[3:6])
print(grid[6:9])
break
print(grid[0:3])
print(grid[3:6])
print(grid[6:9])
add_o=True
## add O player
player_postion_O=int(input ("Enter postion for player O: "))
while add_o:
# at which postion you would like to add the player
player_postion_O=int(input ("Enter postion for player O: "))
## add X player into the board
if grid[player_postion_x-1]!="X" and grid[player_postion_x-1]!="O":
grid[player_postion_x-1]="O"
add_o=False
#to count the number of added players
count=count+1
else:
print ("The postion has been taken")
if grid[0]=="O" and grid[1]=="O" and grid[2]=="O":
print ("Player O win")
print(grid[0:3])
print(grid[3:6])
print(grid[6:9])
break
elif grid[3]=="O" and grid[4]=="O" and grid[5]=="O":
print ("Player O win")
print(grid[0:3])
print(grid[3:6])
print(grid[6:9])
break
elif grid[6]=="O" and grid[7]=="O" and grid[7]=="O":
print ("Player O win")
print(grid[0:3])
print(grid[3:6])
print(grid[6:9])
break
elif grid[0]=="O" and grid[3]=="O" and grid[6]=="O":
print ("Player O win")
print(grid[0:3])
print(grid[3:6])
print(grid[6:9])
break
elif grid[1]=="O" and grid[4]=="O" and grid[7]=="O":
print ("Player O win")
print(grid[0:3])
print(grid[3:6])
print(grid[6:9])
break
elif grid[2]=="O" and grid[5]=="O" and grid[8]=="O":
print ("Player O win")
print(grid[0:3])
print(grid[3:6])
print(grid[6:9])
break
elif grid[0]=="O" and grid[4]=="O" and grid[8]=="O":
print ("Player O win")
print(grid[0:3])
print(grid[3:6])
print(grid[6:9])
break
elif grid[2]=="O" and grid[4]=="O" and grid[6]=="O":
print ("Player O win")
print(grid[0:3])
print(grid[3:6])
print(grid[6:9])
break
print(grid[0:3])
print(grid[3:6])
print(grid[6:9])
if count==9:
print ("Game Over")
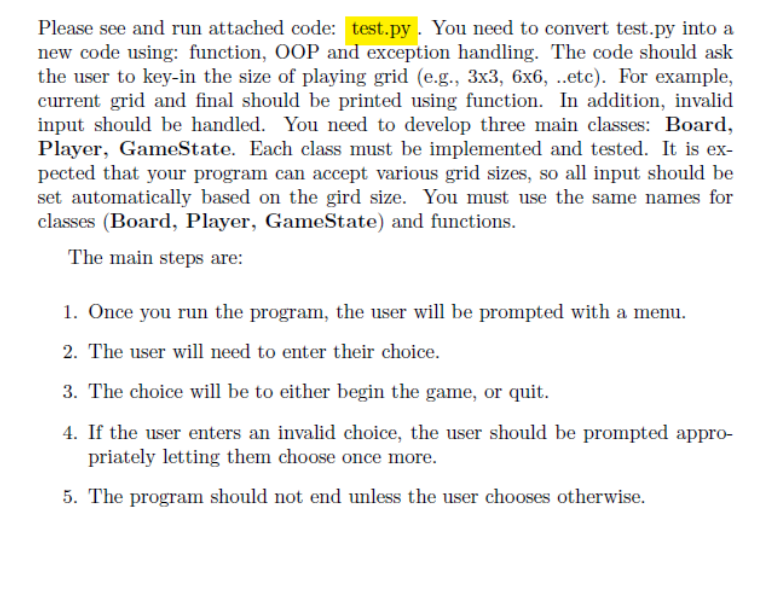


Step by step
Solved in 2 steps

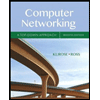
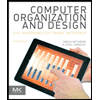
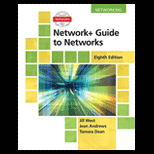
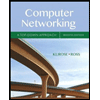
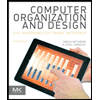
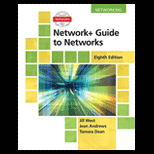
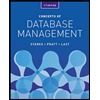
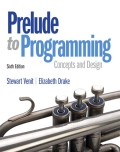
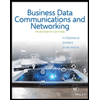