Please see the image attached but I am having compiling issues (instructions on what the objective is is below): Introduction to Programming (C++): Create a program with three vectors (be sure to use: #include ) : One vector for the last name One vector for the first name One vector for the age of the person (whole number) **You determine what sentinel value to use to stop input** So input different kinds of people (first name, last name, and age) until you get to the sentinel value and then output the list of people Calculate the average age of the people and then calculate the Standard Deviation of the ages. Expect to see the means, a function to display the list of names and ages, a function to get the average, and a function to calculate the Standard Deviation **Please use at least 10 people** --------------------------------------------------------------------------------- #include #include #include // Defines various mathematical functions using namespace std; // Functions void func_display(vector& v1, vector& v2, vector& v3); void func_average(vector& v3); void func_std(vector& v3, float avg_ages); void func_display(vector& v1, vector& v2, vector& v3) { // Since all of the vectors are of the same size, the size of any vector can be used cout << "\n PERSON DETAILS"; for (int i = 0; i < v1.size(); i++) { cout << "\nName: " << v1[i] << " " << v2[i]; cout << "\nAge: " << v3[i]; } } void func_average(vector& v3) { int sumAges = 0; cout << "\n COMPUTE AVERAGE AND STANDARD DEVIATION OF AGES"; for (int i = 0; i < v3.size(); i++) sumAges += v3[i]; float avg = (float)sumAges / v3.size(); // Display average cout << "\nAverage: " << avg; // Call the method to show the Standard Deviation func_std(v3, avg); } void func_std(vector& v3, float avg_ages) { float var_ages, st_deviation; for (int i = 0; i < v3.size(); i++) var_ages += pow(v3[i] - avg_ages, 2); var_ages = var_ages / v3.size(); st_deviation = sqrt(var_ages); cout << "\nStandard Deviation:" << st_deviation; } int main() { vectorlast_name; vectorfirst_name; vectorage; int answer, p_age; string l_name, f_name; // Input values until the sentinel value(0) is obtained while (true) { // Last name below cout << "Enter the last name of the individual: "; cin >> l_name; last_name.push_back(l_name); // First name below cout << "Enter the first name of the individual: "; cin >> f_name; first_name.push_back(f_name); // Person's age below cout << "Enter the age of the individual: "; cin >> p_age; age.push_back(p_age); // Require more values? cout << "\nRequire more values? (press 0 to exit OR 1 to continue):"; cin >> answer; if (answer == 0) break; } func_display(first_name, last_name, age); func_average(age); return 0; }
Please see the image attached but I am having compiling issues (instructions on what the objective is is below):
Introduction to Programming (C++):
Create a program with three
One vector for the last name
One vector for the first name
One vector for the age of the person (whole number)
**You determine what sentinel value to use to stop input**
So input different kinds of people (first name, last name, and age) until you get to the sentinel value and then output the list of people
Calculate the average age of the people and then calculate the Standard Deviation of the ages.
Expect to see the means, a function to display the list of names and ages, a function to get the average, and a function to calculate the Standard Deviation
**Please use at least 10 people**
---------------------------------------------------------------------------------
#include <iostream>
#include <vector>
#include <math.h> // Defines various mathematical functions
using namespace std;
// Functions
void func_display(vector<string>& v1, vector<string>& v2, vector<int>& v3);
void func_average(vector<int>& v3);
void func_std(vector<int>& v3, float avg_ages);
void func_display(vector<string>& v1, vector<string>& v2, vector<int>& v3)
{
// Since all of the vectors are of the same size, the size of any vector can be used
cout << "\n PERSON DETAILS";
for (int i = 0; i < v1.size(); i++)
{
cout << "\nName: " << v1[i] << " " << v2[i];
cout << "\nAge: " << v3[i];
}
}
void func_average(vector<int>& v3)
{
int sumAges = 0;
cout << "\n COMPUTE AVERAGE AND STANDARD DEVIATION OF AGES";
for (int i = 0; i < v3.size(); i++)
sumAges += v3[i];
float avg = (float)sumAges / v3.size();
// Display average
cout << "\nAverage: " << avg;
// Call the method to show the Standard Deviation
func_std(v3, avg);
}
void func_std(vector<int>& v3, float avg_ages)
{
float var_ages, st_deviation;
for (int i = 0; i < v3.size(); i++)
var_ages += pow(v3[i] - avg_ages, 2);
var_ages = var_ages / v3.size();
st_deviation = sqrt(var_ages);
cout << "\nStandard Deviation:" << st_deviation;
}
int main()
{
vector<string>last_name;
vector<string>first_name;
vector<int>age;
int answer, p_age;
string l_name, f_name;
// Input values until the sentinel value(0) is obtained
while (true)
{
// Last name below
cout << "Enter the last name of the individual: ";
cin >> l_name;
last_name.push_back(l_name);
// First name below
cout << "Enter the first name of the individual: ";
cin >> f_name;
first_name.push_back(f_name);
// Person's age below
cout << "Enter the age of the individual: ";
cin >> p_age;
age.push_back(p_age);
// Require more values?
cout << "\nRequire more values? (press 0 to exit OR 1 to continue):";
cin >> answer;
if (answer == 0)
break;
}
func_display(first_name, last_name, age);
func_average(age);
return 0;
}


Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

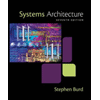
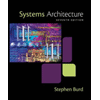