Write the code in c++ without using global variables Purpose: Demonstrate the use of classes Program definition: Design a class bookType that defines a book as a class. a. Each object of the class bookType will hold the following information about a book: title number of authors up to four authors publisher year published ISBN 13 (with dashes) price number of copies in stock (may be 0) b. Include the member functions to perform the various operations on objects of type bookType: Include individual get and set functions for all member variables. c. Add a member function to update the number of copies in stock. d. Add the appropriate constructors and a destructor (if one is needed). Ensure a default constructor is coded and the constructor initializes all data members. Remember, an array of classes uses a default constructor only. e. ALL member variables must be private and accessed through member functions. The main client program CANNOT access the variables directly. f. Ensure the class declaration and class implementation files are in separate header and code (.cpp) files. This assignment will require the creation of a project. g. Write a client program that uses the class bookType and tests various operations on the objects of the class bookType. Declare an array of 50 components of type bookType in the client program. h. Using a function, open the attached datafile (bookData.txt). Request the file name from the user. If the file does not open, display a proper message and exit the program. The file open should be in main and test a return value of success or failure. i.Use a function and the attached datafile (bookData.txt) to initially load the data. The data file is of the form: Book Title ISBN Publisher Year Published Cost Number In Stock Number of Authors List of Authors j.Read until end of file. Do not hardcode and use the actual number of books as the maximum (limiting factor) for the array of books. Keep a count of actual rows read and pass into and out of any functions and processes. k. Have the program provide the following capabilities (by user request): Through a menu system provide the capability to: 1) Search for a book by ISBN, Author or Book title. If the store sells the book, return an appropriate message. If the store does not sell the book, return an appropriate message. If the store sells the book, print the Title, authors, ISBN, publisher, price and the number in stock for the book. 2) Print a list of ALL the books in stock. This list will include all the information available for a book. A book is in stock when the number in stock is greater than 0. 3) Update the number of copies in stock. The user may search for the book using one of the capabilities indicated in (1) (by ISBN, Author or Title) and add to the number of copies by the number entered. This will simulate the process of restocking. 4) Print a list of ALL the books the store sells, even if not in stock at the time. The list will include all the information available for a book. 5) The user may exit the program by keying in an exit command or choice determined by you. l.Place all function prototypes and const variables for the client program in a different header file than the class header file. Include the header file in the client program. m. Include error checking (invalid menu choices, file open errors, etc.) n. Once your class is declared and defined and your client program written, test each of the menu options to ensure proper processing and program behavior. All classes must have a class definition header file (*.h) and a class implementation file (*.cpp). You must have a main program that exercises these classes (*.cpp). Please show the output to
Write the code in c++ without using global variables Purpose: Demonstrate the use of classes Program definition: Design a class bookType that defines a book as a class. a. Each object of the class bookType will hold the following information about a book: title number of authors up to four authors publisher year published ISBN 13 (with dashes) price number of copies in stock (may be 0) b. Include the member functions to perform the various operations on objects of type bookType: Include individual get and set functions for all member variables. c. Add a member function to update the number of copies in stock. d. Add the appropriate constructors and a destructor (if one is needed). Ensure a default constructor is coded and the constructor initializes all data members. Remember, an array of classes uses a default constructor only. e. ALL member variables must be private and accessed through member functions. The main client program CANNOT access the variables directly. f. Ensure the class declaration and class implementation files are in separate header and code (.cpp) files. This assignment will require the creation of a project. g. Write a client program that uses the class bookType and tests various operations on the objects of the class bookType. Declare an array of 50 components of type bookType in the client program. h. Using a function, open the attached datafile (bookData.txt). Request the file name from the user. If the file does not open, display a proper message and exit the program. The file open should be in main and test a return value of success or failure. i.Use a function and the attached datafile (bookData.txt) to initially load the data. The data file is of the form: Book Title ISBN Publisher Year Published Cost Number In Stock Number of Authors List of Authors j.Read until end of file. Do not hardcode and use the actual number of books as the maximum (limiting factor) for the array of books. Keep a count of actual rows read and pass into and out of any functions and processes. k. Have the program provide the following capabilities (by user request): Through a menu system provide the capability to: 1) Search for a book by ISBN, Author or Book title. If the store sells the book, return an appropriate message. If the store does not sell the book, return an appropriate message. If the store sells the book, print the Title, authors, ISBN, publisher, price and the number in stock for the book. 2) Print a list of ALL the books in stock. This list will include all the information available for a book. A book is in stock when the number in stock is greater than 0. 3) Update the number of copies in stock. The user may search for the book using one of the capabilities indicated in (1) (by ISBN, Author or Title) and add to the number of copies by the number entered. This will simulate the process of restocking. 4) Print a list of ALL the books the store sells, even if not in stock at the time. The list will include all the information available for a book. 5) The user may exit the program by keying in an exit command or choice determined by you. l.Place all function prototypes and const variables for the client program in a different header file than the class header file. Include the header file in the client program. m. Include error checking (invalid menu choices, file open errors, etc.) n. Once your class is declared and defined and your client program written, test each of the menu options to ensure proper processing and program behavior. All classes must have a class definition header file (*.h) and a class implementation file (*.cpp). You must have a main program that exercises these classes (*.cpp). Please show the output to
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Write the code in c++ without using global variables
Purpose:
Demonstrate the use of classes
Program definition:
Design a class bookType that defines a book as a class.
a. Each object of the class bookType will hold the following information about a book:
title
number of authors
up to four authors
publisher
year published
ISBN 13 (with dashes)
price
number of copies in stock (may be 0)
b. Include the member functions to perform the various operations on objects of type bookType: Include individual get and set functions for all member variables.
c. Add a member function to update the number of copies in stock.
d. Add the appropriate constructors and a destructor (if one is needed). Ensure a default constructor is coded and the constructor initializes all data members. Remember, an array of classes uses a default constructor only.
e. ALL member variables must be private and accessed through member functions. The main client program CANNOT access the variables directly.
f. Ensure the class declaration and class implementation files are in separate header and code (.cpp) files. This assignment will require the creation of a project.
g. Write a client program that uses the class bookType and tests various operations on the objects of the class bookType. Declare an array of 50 components of type bookType in the client program.
h. Using a function, open the attached datafile (bookData.txt). Request the file name from the user. If the file does not open, display a proper message and exit the program. The file open should be in main and test a return value of success or failure.
i.Use a function and the attached datafile (bookData.txt) to initially load the data. The data file is of the form:
Book Title
ISBN
Publisher
Year Published
Cost
Number In Stock
Number of Authors
List of Authors
j.Read until end of file. Do not hardcode and use the actual number of books as the maximum (limiting factor) for the array of books. Keep a count of actual rows read and pass into and out of any functions and processes.
k. Have the program provide the following capabilities (by user request):
Through a menu system provide the capability to:
1) Search for a book by ISBN, Author or Book title. If the store sells the book, return an appropriate message. If the store does not sell the book, return an appropriate message. If the store sells the book, print the Title, authors, ISBN, publisher, price and the number in stock for the book.
2) Print a list of ALL the books in stock. This list will include all the information available for a book. A book is in stock when the number in stock is greater than 0.
3) Update the number of copies in stock. The user may search for the book using one of the capabilities indicated in (1) (by ISBN, Author or Title) and add to the number of copies by the number entered. This will simulate the process of restocking.
4) Print a list of ALL the books the store sells, even if not in stock at the time. The list will include all the information available for a book.
5) The user may exit the program by keying in an exit command or choice determined by you.
l.Place all function prototypes and const variables for the client program in a different header file than the class header file. Include the header file in the client program.
m. Include error checking (invalid menu choices, file open errors, etc.)
n. Once your class is declared and defined and your client program written, test each of the menu options to ensure proper processing and program behavior.
All classes must have a class definition header file (*.h) and a class implementation file (*.cpp). You must have a main program that exercises these classes (*.cpp).
Please show the output too
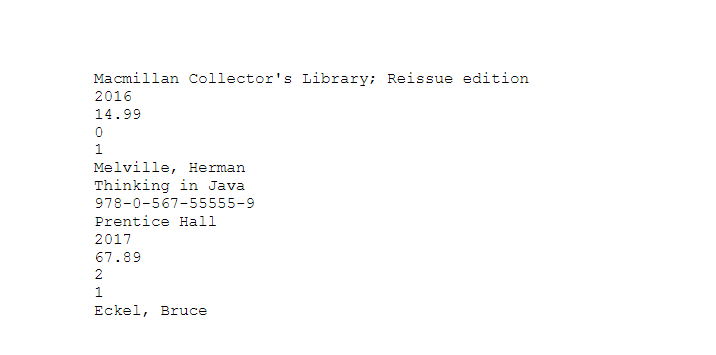
Transcribed Image Text:Macmillan Collector's Library; Reissue edition
2016
14.99
1
Melville, Herman
Thinking in Java
978-0-567-55555-9
Prentice Hall
2017
67.89
2
1
Eckel, Bruce
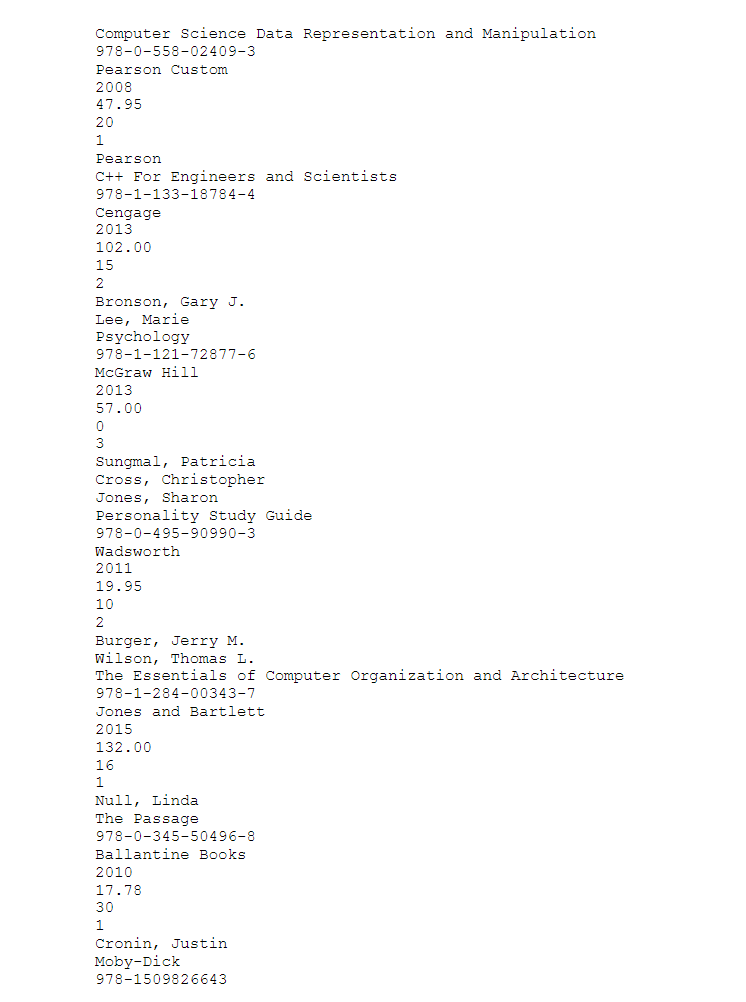
Transcribed Image Text:Computer Science Data Representation and Manipulation
978-0-558-02409-3
Pearson Custom
2008
47.95
20
Pearson
C++ For Engineers and Scientists
978-1-133-18784-4
Cengage
2013
102.00
15
2
Bronson, Gary J.
Lee, Marie
Psychology
978-1-121-72877-6
McGraw Hill
2013
57.00
Sungmal, Patricia
Cross, Christopher
Jones, Sharon
Personality Study Guide
978-0-495-90990-3
Wadsworth
2011
19.95
10
2
Burger, Jerry M.
Wilson, Thomas L.
The Essentials of Computer Organization and Architecture
978-1-284-00343-7
Jones and Bartlett
2015
132.00
16
Null, Linda
The Passage
978-0-345-50496-8
Ballantine Books
2010
17.78
30
Cronin, Justin
Moby-Dick
978-1509826643
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 9 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
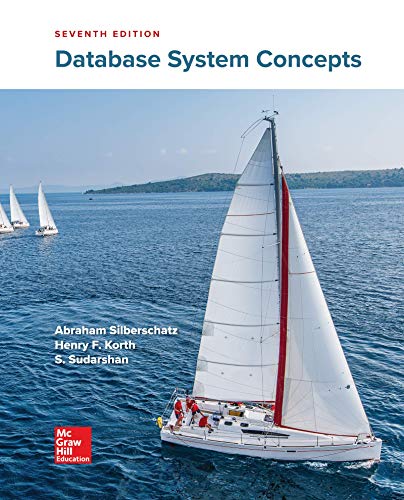
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
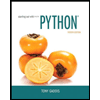
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
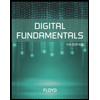
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
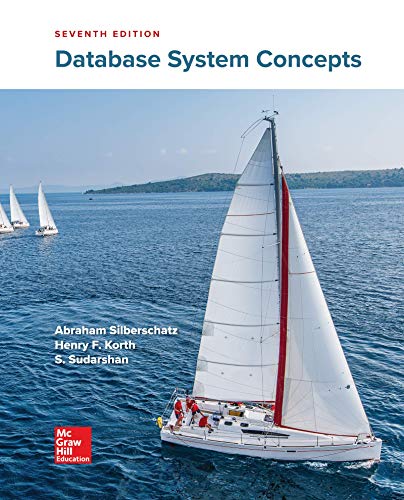
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
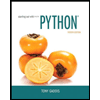
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
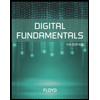
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
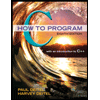
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
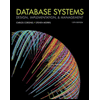
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
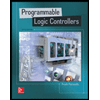
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education