Please Use Netbeans Create four pages: Three Classes: Employee, Department, and Company A program MyCompany which supplies employee name and salary information and prints out the total salary of all the employees. Pass in all four .java files. 1) Create a class called "Employee" with the following properties: name (String) age (int) salary (double) In the Employee class, create a constructor that accepts and sets an employee’s name, age, and salary. Create getters for name, age, and salary. 2) Create a class called "Department" with the following properties: i (int) name (String) Employee[] employees=new Employee[10]; In the Department class, create a constructor that accepts and sets the department name. Also set i=0 (to initialize the value). In the Department class create a method called "addEmployee" that accepts an Employee object and adds it to the array of employees. (Hint, include i++ to increment the value of i.) In the Department class, create a method called "getTotalSalary" as follows: public double getTotalSalary() { double total = 0; for (int z=0;z
Please Use Netbeans
Create four pages:
Three Classes: Employee, Department, and Company
A
salary of all the employees.
Pass in all four .java files.
1) Create a class called "Employee" with the following properties:
name (String)
age (int)
salary (double)
In the Employee class, create a constructor that accepts and sets an employee’s name, age, and salary.
Create getters for name, age, and salary.
2) Create a class called "Department" with the following properties:
i (int)
name (String)
Employee[] employees=new Employee[10];
In the Department class, create a constructor that accepts and sets the department name. Also set i=0
(to initialize the value).
In the Department class create a method called "addEmployee" that accepts an Employee object and
adds it to the array of employees. (Hint, include i++ to increment the value of i.)
In the Department class, create a method called "getTotalSalary" as follows:
public double getTotalSalary() {
double total = 0;
for (int z=0;z<i;z++) {
total += employees[z].getSalary();
}
return total;
}
3) Create a class called "Company" with the following properties:
j (int)
name (String)
Department[] departments=new Department[10];
In the Company class, create a constructor that accepts a name and sets the company name. Also,
initialize j=0.
In the Company class, create a method called "addDepartment" that accepts a Department object and
adds it to the array of departments. (Hint, include j++ to increment the value of j.)
In the Company class, create a method called "getTotalSalary" as follows:
public double getTotalSalary() {
double total = 0;
for (int z=0;z<j;z++) {
total += departments[z].getTotalSalary();
}
return total;
}
4) Create a program MyCompany as given below:
public class MyCompany {
public static void main(String[] args) {
Employee e1 = new Employee("John", 25, 5000);
Employee e2 = new Employee("Jane", 30, 6000);
Employee e3 = new Employee("Jim", 35, 7000);
Employee e4 = new Employee("Jack", 40, 8000);
Employee e5 = new Employee("Jill", 45, 9000);
Department d1 = new Department("Sales");
d1.addEmployee(e1);
d1.addEmployee(e2);
Department d2 = new Department("Marketing");
d2.addEmployee(e3);
d2.addEmployee(e4);
Department d3 = new Department("IT");
d3.addEmployee(e5);
Company c = new Company("ACME Inc.");
c.addDepartment(d1);
c.addDepartment(d2);
c.addDepartment(d3);
System.out.println("Total salary of all employees: " + c.getTotalSalary());
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

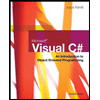
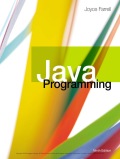
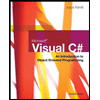
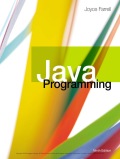