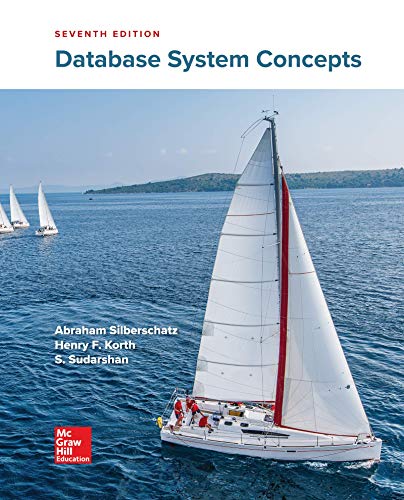
Concept explainers
Population
Compile and run CreateCityDB.java which will create a Java DB database named CityDB. The CityDB database will have a table named City, with the following columns: CityName & Population. Their Data Types: CHAR(50) Primary Key & DOUBLE. The CityName column stores the name of a city, and the Population column stores the population of that city. After you run the CreateCityDB.java
- Sort the list of cities by population, in ascending order.
- Sort the list of cities by population, in descending order.
- Sort the list of cities by name.
- Get the total population of all the cities.
- Get the average population of all the cities.
- Get the highest population.
- Get the lowest population.
CreateCityDB.java
import java.sql.*;
/**
This program creates the CityDB database. *
*/
public class CreateCityDB
{
public static void main(String[] args) throws Exception
{
String sql;
final String DB_URL = "jdbc:derby:CityDB;create=true";
// Create a connection to the database.
Connection conn = DriverManager.getConnection(DB_URL);
// Create a Statement object.
Statement stmt = conn.createStatement();
try
{
// Create the City table.
System.out.println("Creating the City table...");
stmt.execute("CREATE TABLE City (" +
"CityName CHAR(25) NOT NULL PRIMARY KEY, " +
"Population DOUBLE)");
// Add some rows to the new table.
sql = "INSERT INTO City VALUES" +
"('Beijing', 12500000)";
stmt.executeUpdate(sql);
sql = "INSERT INTO City VALUES" +
"('Buenos Aires', 13170000)";
stmt.executeUpdate(sql);
sql = "INSERT INTO City VALUES" +
"('Cairo', 14450000)";
stmt.executeUpdate(sql);
sql = "INSERT INTO City VALUES" +
"('Calcutta', 15100000)";
stmt.executeUpdate(sql);
sql = "INSERT INTO City VALUES" +
"('Delhi', 18680000)";
stmt.executeUpdate(sql);
sql = "INSERT INTO City VALUES" +
"('Jakarta', 18900000)";
stmt.executeUpdate(sql);
sql = "INSERT INTO City VALUES" +
"('Karachi', 11800000)";
stmt.executeUpdate(sql);
sql = "INSERT INTO City VALUES" +
"('Lagos', 13488000)";
stmt.executeUpdate(sql);
sql = "INSERT INTO City VALUES" +
"('London', 12875000)";
stmt.executeUpdate(sql);
sql = "INSERT INTO City VALUES" +
"('Los Angeles', 15250000)";
stmt.executeUpdate(sql);
sql = "INSERT INTO City VALUES" +
"('Manila', 16300000)";
stmt.executeUpdate(sql);
sql = "INSERT INTO City VALUES" +
"('Mexico City', 20450000)";
stmt.executeUpdate(sql);
sql = "INSERT INTO City VALUES" +
"('Moscow', 15000000)";
stmt.executeUpdate(sql);
sql = "INSERT INTO City VALUES" +
"('Mumbai', 19200000)";
stmt.executeUpdate(sql);
sql = "INSERT INTO City VALUES" +
"('New York City', 19750000)";
stmt.executeUpdate(sql);
sql = "INSERT INTO City VALUES" +
"('Osaka', 17350000)";
stmt.executeUpdate(sql);
sql = "INSERT INTO City VALUES" +
"('Sao Paulo', 18850000)";
stmt.executeUpdate(sql);
sql = "INSERT INTO City VALUES" +
"('Seoul', 20550000)";
stmt.executeUpdate(sql);
sql = "INSERT INTO City VALUES" +
"('Shanghai', 16650000)";
stmt.executeUpdate(sql);
sql = "INSERT INTO City VALUES" +
"('Tokyo', 32450000)";
stmt.executeUpdate(sql);
}
catch(Exception ex)
{
System.out.println("ERROR: " + ex.getMessage());
}
finally
{
// Close the resources.
stmt.close();
conn.close();
System.out.println("Done");
}
}
}
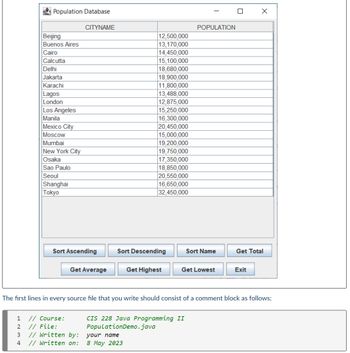

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

- MySql Workbench CREATE TABLE students ( id INT PRIMARY KEY, first_name VARCHAR(50), last_name VARCHAR(50), age INT, major VARCHAR(50), faculty VARCHAR(50)); CREATE TABLE location ( id INT PRIMARY KEY, name VARCHAR(50), rooms INT); CREATE TABLE faculty ( id INT PRIMARY KEY, name VARCHAR(50), department_id INT); 1. List last name of all students whose first name is longer than 4 letters in ascending order accordingto the last name. Duplicated rows should be removed from the output.2. Count the total number of rooms in Location.3. Find the number of students in each major.4. Find the number of employees in each department who get no commission or have salary less than5000.5. Find the maximum salary of employees in each department that the employee was hired 15 yearsbefore now. *hint: user TIMESTAMPDIFF(<unit type>,<Date_value 1>,<Date_value 2>), the unitcan be YEAR, MONTH, DAY, HOUR, etc...arrow_forwardSQL CODE FOR For the players who show up in Batting, Bowling, and Fielding tables, create a list that shows their names, runs they have scored, wickets they have taken, and catches they have taken? table is in picture (bowling table is same as batting and fielding )arrow_forward1-Write the syntax to insert into a relational table called students the address column references an object table called addresses that was created using an address_type. Other columns exist in the students table, but you are only inserting into the ones below. Aliases used should be the first letter of the table name, eg students would be s, addresses would be a Relational Table Name students attribute student_id attribute surname attribute addressarrow_forward
- Assume that a database has a table named Stock, with the following columns:Column Name TypeTrading_Symbol nchar(10)Company_Name nchar(25)Num_Shares intPurchase_Price moneySelling_Price moneyWrite a Select statement that returns the Trading_Symbol column only from the rows where Purchase_Price is greater than $25.00.arrow_forwardAccess Assignment Problem: JMS TechWizards is a local company that provides technical services to several small businesses in the area. The company currently keeps its technicians and clients’ records on papers. The manager requests you to create a database to store the technician and clients’ information. The following table contains the clients’ information. Client Number Client Name Street City State Postal Code Telephone Number Billed Paid Technician Number AM53 Ashton-Mills 216 Rivard Anderson TX 78077 512-555-4070 $315.50 $255.00 22 AR76 The Artshop 722 Fisher Liberty Corner TX 78080 254-555-0200 $535.00 $565.00 23 BE29 Bert's Supply 5752 Maumee Liberty Corner TX 78080 254-555-2024 $229.50 $0.00 23 DE76 D & E Grocery 464 Linnell Anderson TX 78077 512-555-6050 $485.70…arrow_forwardThe Save Transaction button depicted in the screen attached is used to save relevant data to the sales table and the salesdetails tables from the depicted schema. When this button is clicked it calls the saveTransaction() function that is within the PosDAO class, it passes to this function an ArrayList of salesdetails object, this list contains the data entered into the jTable which is the products and qty being sold.Write the saveTransaction function. You are to loop through the items and get the total sales, next you are to insert the current date and the total sales into the sales table. Reminder that the sales table SalesNumber field is set to AUTO-INCREMENT, hence the reason for only entering the total sales and current date in sales table.arrow_forward
- Below is a database with 2 tables - Painter, Painting: Painting table: primary key = Painting Num, foreign key = Painter_Num 11 Painting Num Painting_title Painter_Num 1336 Tired peasants 123 1337 Dawn Thunder 111 1338 Plastic Paradise 111 Hasty Exit Vanilla Chocolate 123 1339 134 1340 1341 X-files 123 Painter table: primary key Painter Num, no foreign key Painter Num Painter_lastname Painter_Firstname Painter_Initial Nationality 111 Ross Smithson P British 123 Angela Itero X Italian The above database contains an error in the data. Identify and describe this error.arrow_forwardQuery 3: Write a parameter query to display the names of all prospects each member tried to recruit based on the member’s first name and the member’s last name you input. List the member’s First Name, member’s Last Name, prospect’s First Name, and prospect’s Last Name (in this order in the query grid). Display the member’s First Name Heading as Member First Name, member’s Last Name Heading as Member Last Name, prospect’s first name heading as Prospect First Name, and prospect’s last name heading as Prospect Last Name. Sort the list by Member Last Name, Member First Name, Prospect Last Name, and Prospect First Name, all ascending order. (WE ARE USING ACCESS SO I AM JUST TRYING TO UNDERSTAND WHAT TO PUT AND PLUG IN ETC. USE MY PICTURES AS REFERRENCE!)arrow_forwardThe MongoDB shell is an interactive ______interface to MongoDB. You can use the mongo shell to query and update data as well as perform administrative operations. A. NodeJS B. JavaScript C. C D. SQLarrow_forward
- Database coursearrow_forwardin C# in Visual Studios using Windows Form App(.Net Framework) Thank youarrow_forwardBelow is “Book Order,” the only table in library management system’s database. The design of “Book Order” as you may tell is in the zero normal formal form, you as the database designer want to convert the design into the third normal form. Order ID Special order date Customer ID Customer last name Customer First name Customer birth date Book ISBN1 Book Title 1 Book Author 1 Book publication year 1 Book ISBN2 Book Title 2 Book Author 2 Book publication year 2 Store ID Store name Store location Special order status Book Order(Order ID, Special order date, Customer ID, Customer last name, Customer First name, Customer birth date, Book ISBN1, Book Title 1, Book Author 1, Book publication year 1, Book ISBN2, Book Title 2, Book Author 2, Book publication year 2, Store ID, Store name, Store location, Special order status)arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
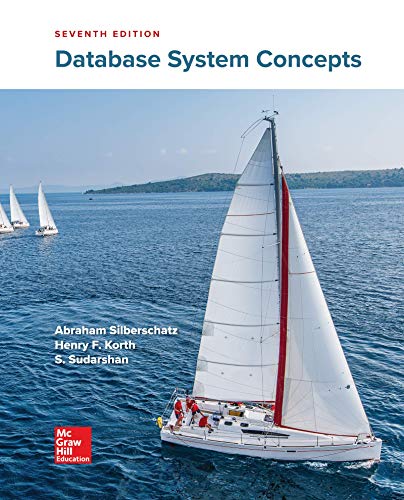
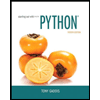
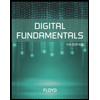
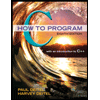
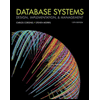
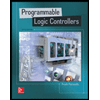