#pragma once #include "person.h" using namespace std; class Contact :public Person { public: Contact(); }; void setContactId(int id) { } int getContactId() const { } setId(id); private: return getId(); // Todo overloaded operators for !=, >, <, >=₁ <=, etc bool operator==(const Contact&) const; bool operator !=(const Contact& others) const; bool operator> (const Contact& otherS) const; bool operator<(const Contact& otherS) const; bool operator>=(const Contact& otherS) const; bool operator<=(const Contact& otherS) const; // todo
#pragma once #include "person.h" using namespace std; class Contact :public Person { public: Contact(); }; void setContactId(int id) { } int getContactId() const { } setId(id); private: return getId(); // Todo overloaded operators for !=, >, <, >=₁ <=, etc bool operator==(const Contact&) const; bool operator !=(const Contact& others) const; bool operator> (const Contact& otherS) const; bool operator<(const Contact& otherS) const; bool operator>=(const Contact& otherS) const; bool operator<=(const Contact& otherS) const; // todo
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter10: Classes And Data Abstraction
Section: Chapter Questions
Problem 17PE
Related questions
Concept explainers
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Question
Implement a new class Contact :public Person
I provided person.h you don't have to touch person.h just need help on contact.h class public person and private person please help me
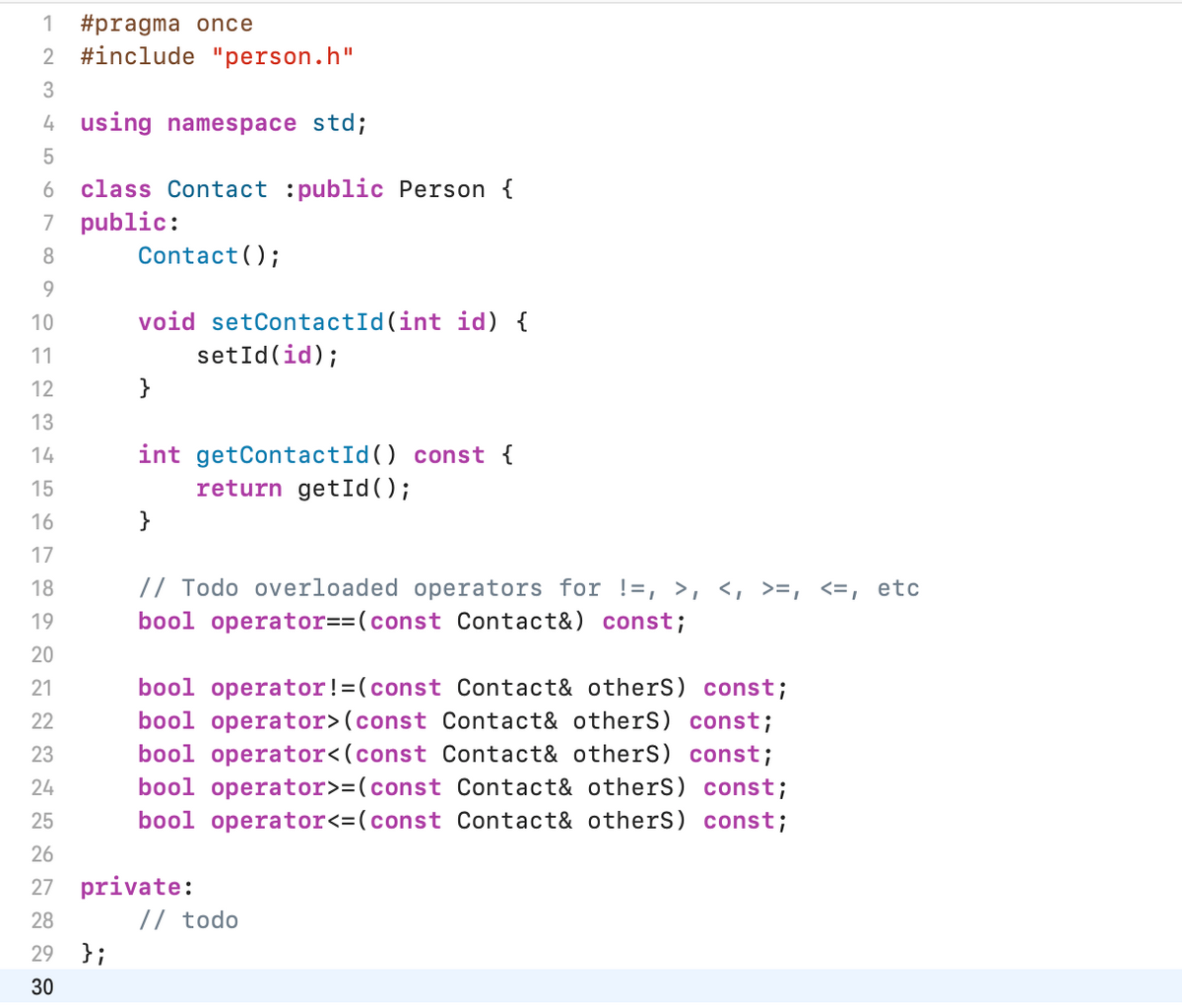
Transcribed Image Text:1 #pragma once
2 #include "person.h"
3
4
5
using namespace std;
6 class Contact :public Person {
7 public:
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29 };
30
Contact();
void setContactId(int id) {
}
int getContactId() const {
}
setId(id);
return getId();
// Todo overloaded operators for !=, >, <, >=, <=, etc
bool operator==(const Contact&) const;
private:
bool operator!=(const Contact& others) const;
bool operator> (const Contact& otherS) const;
bool operator<(const Contact& otherS) const;
bool operator>=(const Contact& others) const;
bool operator<=(const Contact& others) const;
// todo
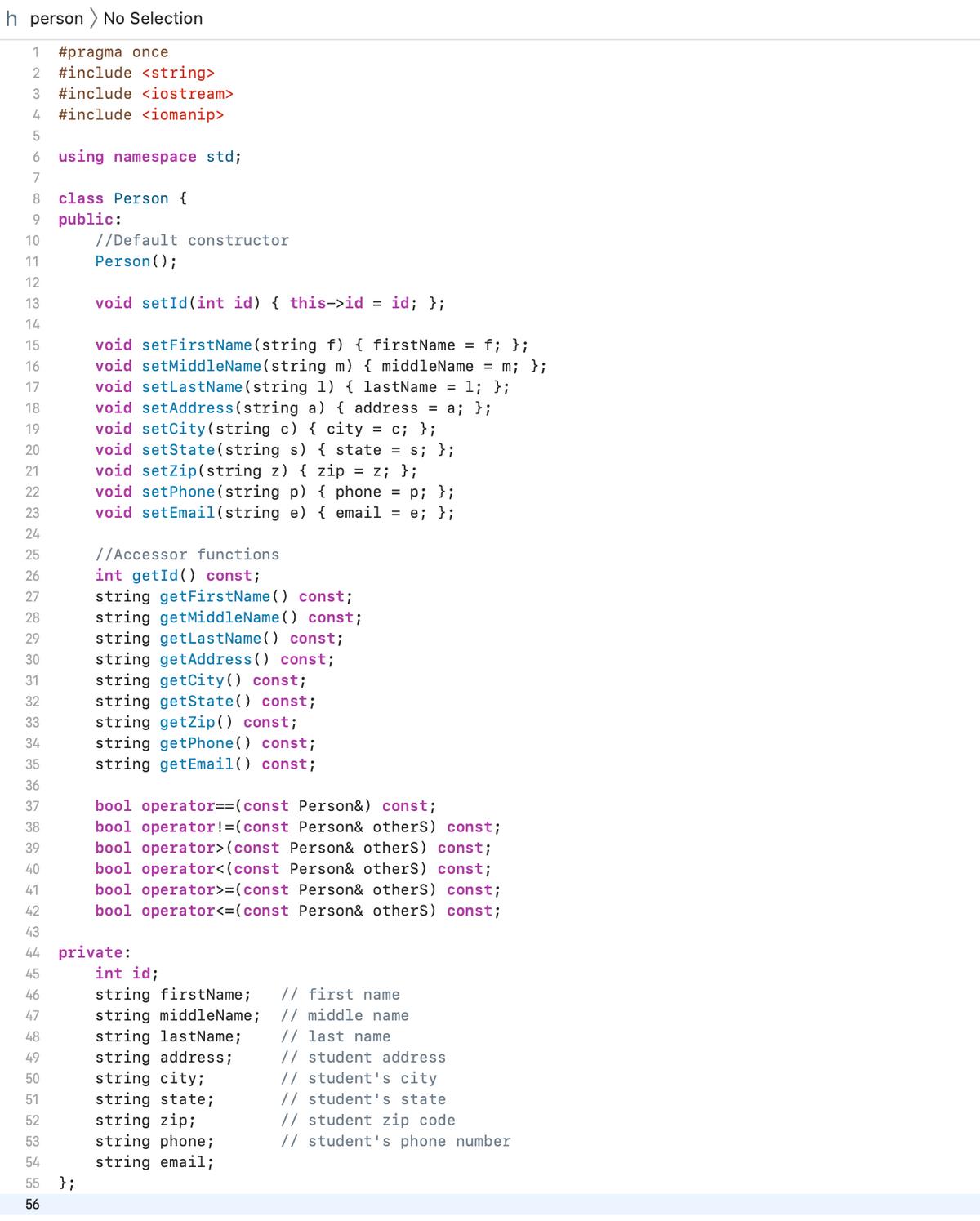
Transcribed Image Text:person > No Selection
1 #pragma once
2 #include <string>
3 #include <iostream>
4 #include <iomanip>
5
6 using namespace std;
7
8 class Person {
9 public:
10
11
12
13
14
DEFOO¦~~~~~~~¦¦¦¦¦¦¦¦¦¦¦¦÷FIF FF FF¶¶¦¦✿*¦¦
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
45
46
47
44 private:
48
49
50
51
52
53
54
55 };
//Default constructor
Person();
56
void setId(int id) { this->id = id; };
void setFirstName (string f) { firstName = f;
void setMiddleName(string m) { middleName = m; };
void setLastName (string 1) { lastName = 1; };
void setAddress (string a) { address = a; };
void setCity (string c) { city = c; };
setState(string s) { state = s; };
setZip(string z) { zip = z; };
void
void
void setPhone (string p) { phone = p; };
void setEmail(string e) { email = e; };
//Accessor functions
int getId() const;
string getFirstName() const;
string getMiddleName() const;
string getLastName() const;
string getAddress () const;
string getCity() const;
string getState() const;
string getZip() const;
string getPhone () const;
string getEmail() const;
bool operator==(const Person&) const;
bool operator !=(const Person& others) const;
bool operator> (const Person& otherS) const;
bool operator<(const Person& others) const;
bool operator>=(const Person& otherS) const;
bool operator<=(const Person& otherS) const;
int id;
string firstName; // first name
string middleName;
// middle name
string lastName; // last name
string address;
string city;
string state;
string zip;
string phone;
string email;
// student address
// student's city
// student's state
// student zip code
// student's phone number
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
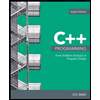
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
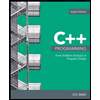
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning