In the file Calculator.java, write a class called Calculator that emulates basic functions of a calculator: add, subtract, multiply, divide, and clear. The class has one private member field called value for the calculator's current value. Implement the following Constructor and instance methods as listed below: public Calculator() - Constructor method to set the member field to 0.0 public void add(double val) - add the parameter to the member field public void subtract(double val) - subtract the parameter from the member field public void multiply(double val) - multiply the member field by the parameter public void divide(double val) - divide the member field by the parameter public void clear( ) - set the member field to 0.0 public double getValue( ) - return the member field
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Calculator Class
In the file Calculator.java, write a class called Calculator that emulates basic functions of a calculator: add, subtract, multiply, divide, and clear. The class has one private member field called value for the calculator's current value. Implement the following Constructor and instance methods as listed below:
- public Calculator() - Constructor method to set the member field to 0.0
- public void add(double val) - add the parameter to the member field
- public void subtract(double val) - subtract the parameter from the member field
- public void multiply(double val) - multiply the member field by the parameter
- public void divide(double val) - divide the member field by the parameter
- public void clear( ) - set the member field to 0.0
- public double getValue( ) - return the member field
Given two double input values num1 and num2, the program outputs the following values:
- The initial value of the instance field, value
- The value after adding num1
- The value after multiplying by 3
- The value after subtracting num2
- The value after dividing by 2
- The value after calling the clear() method
Ex: If the input is:
10.0 5.0the output is:
0.0import java.util.Scanner;
public class LabProgram {
public static void main(String[] args) {
Calculator calc = new Calculator();
Scanner keyboard = new Scanner(System.in);
double num1 = keyboard.nextDouble();
double num2 = keyboard.nextDouble();
// 1. The initial value
System.out.println(calc.getValue());
// 2. The value after adding num1
calc.add(num1);
System.out.println(calc.getValue());
// 3. The value after multiplying by 3
calc.multiply(3);
System.out.println(calc.getValue());
// 4. The value after subtracting num2
calc.subtract(num2);
System.out.println(calc.getValue());
// 5. The value after dividing by 2
calc.divide(2);
System.out.println(calc.getValue());
// 6. The value after calling the clear() method
calc.clear();
System.out.println(calc.getValue());
}
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

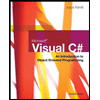
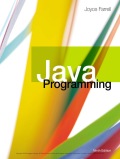
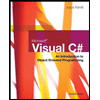
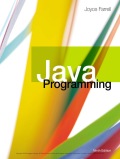