Private Member Functions The member functions declared private, isLeap, daysPerMonth, name, number, are helper functions - member functions that will never be needed by a user of the class, and so do not belong to the public interface (which is why they are "private"). They are, however, needed by the interface functions (public member functions), which use them to test the validity of arguments and construct valid dates. For example, the constructor that passes in the month as a string will call the number function to assign a value to the unsigned member variable month. isLeap: The rule for whether a year is a leap year is: (year % 4 == 0) implies leap year except (year % 100 == 0) implies NOT leap year except (year % 400 == 0) implies leap year So, for instance, year 2000 is a leap year, but 1900 is NOT a leap year. Years 2004, 2008, 2012, 2016, etc. are all leap years. Years 2005, 2006, 2007, 2009, 2010, etc. are NOT leap years. Output Specifications Read the specifications for the print function carefully. The only cout statements within your Date member functions should be: the "Invalid Date" warnings in the constructors in your two print functions Required Main Function You must use the provided main function and global function getDate as they are here. You may not change these functions at all to pass the tests in submit mode.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Coding Language C++
Note: Do not make use of coding techniques that are too advanced. Techniques such as linked lists, recursive functions are not permitted.
Must be done using classes
Private Member Functions
The member functions declared private, isLeap, daysPerMonth, name, number, are helper functions - member functions that will never be needed by a user of the class, and so do not belong to the public interface (which is why they are "private"). They are, however, needed by the interface functions (public member functions), which use them to test the validity of arguments and construct valid dates. For example, the constructor that passes in the month as a string will call the number function to assign a value to the unsigned member variable month.
isLeap: The rule for whether a year is a leap year is:
- (year % 4 == 0) implies leap year
- except (year % 100 == 0) implies NOT leap year
- except (year % 400 == 0) implies leap year
So, for instance, year 2000 is a leap year, but 1900 is NOT a leap year. Years 2004, 2008, 2012, 2016, etc. are all leap years. Years 2005, 2006, 2007, 2009, 2010, etc. are NOT leap years.
Output Specifications
Read the specifications for the print function carefully. The only cout statements within your Date member functions should be:
- the "Invalid Date" warnings in the constructors
- in your two print functions
Required Main Function
You must use the provided main function and global function getDate as they are here. You may not change these functions at all to pass the tests in submit mode.
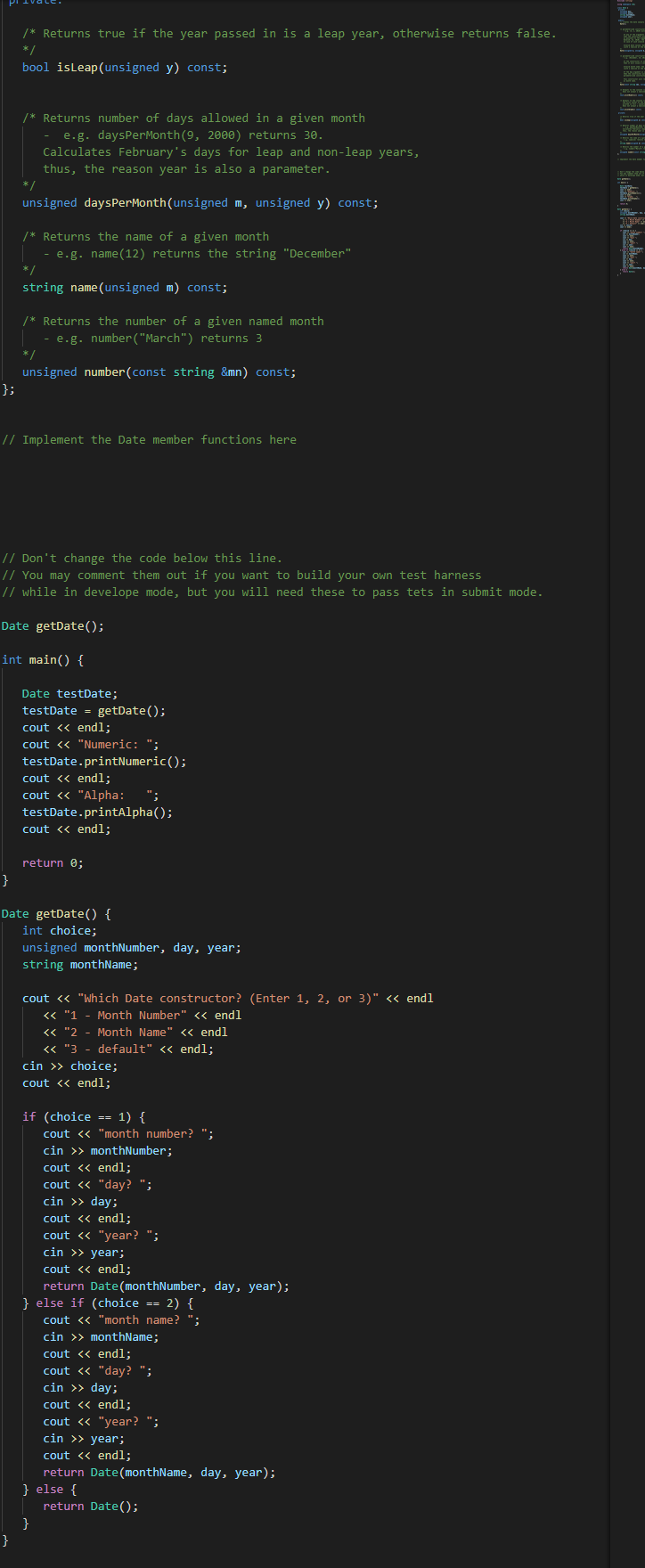
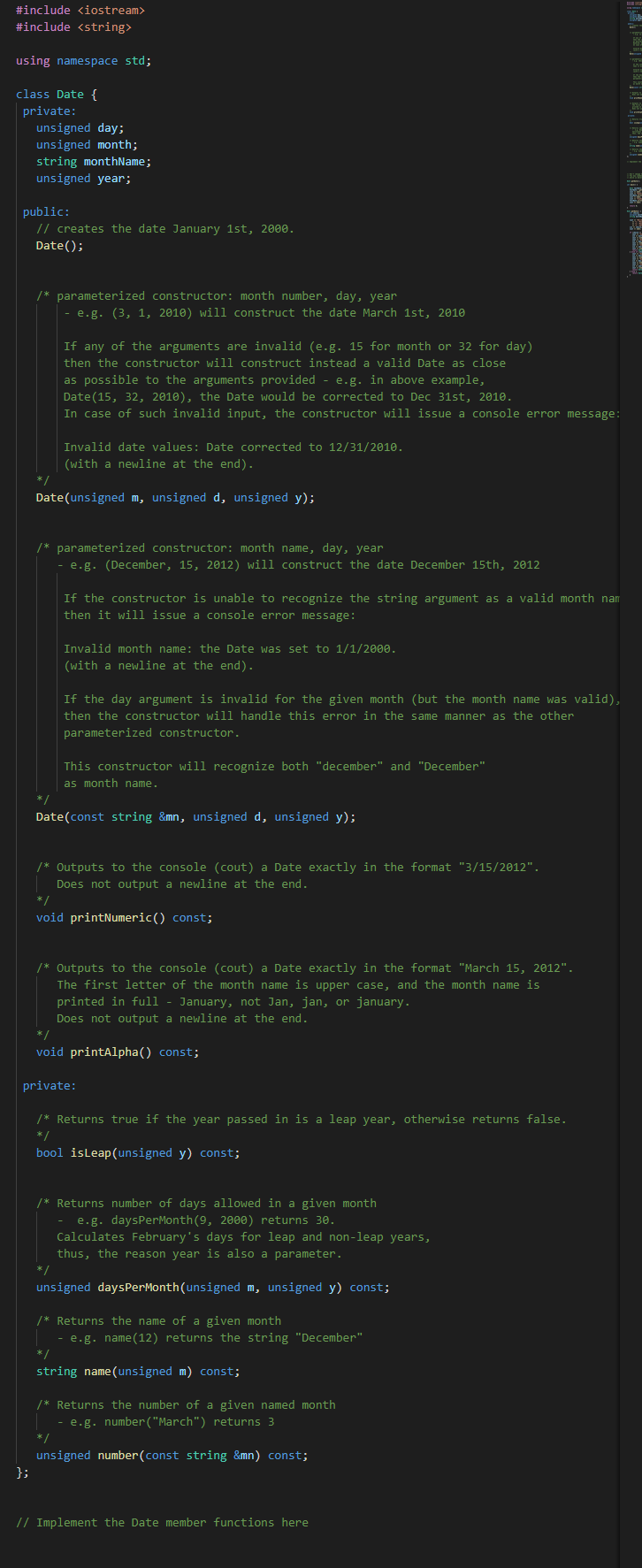

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

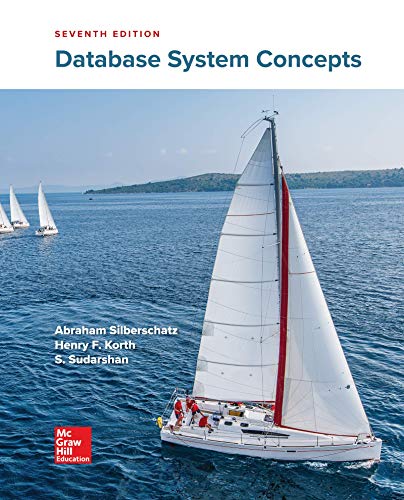
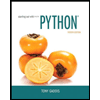
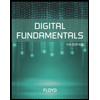
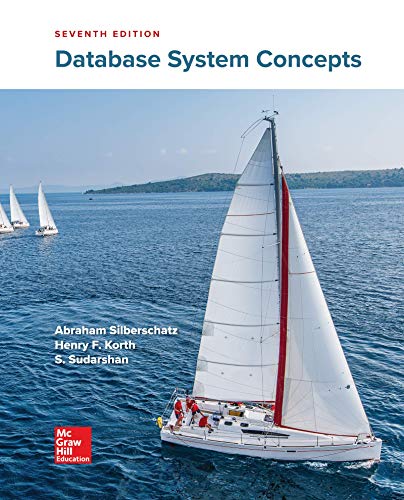
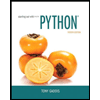
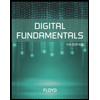
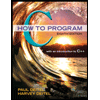
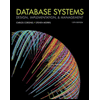
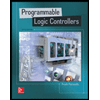