Problem Definition Task. Your task is to develop a python program that reads input from text file and finds if the alphanumeric sequence in each line of the provided input file is valid for Omani car license plate. The rules for valid sequences for car plates in Oman are as follows: Each sequence is composed of 1 to 5 digits followed by one or two letters. Digits cannot start with 0, for instance, 00, 011, or 09 are not valid digit sequences. The following list are the only valid letter combinations: ['A','AA','AB','AD','AR','AM','AW",'AY', 'B','BA','BB','BD','BR','BM','BW','BY', 'D','DA','DD','DR','DW','DY', 'R', RA','RR','RM', RW','RY', 'S','SS', 'M",'MA','MB','MM', MW','MY', "W',WA', WB','WW', Y,YA','YB',YD', YR',YW',YY] Program Input/Output. Your program should read input from a file named plates.txt and write lines with valid sequences to a file named valid.txt. Any line from the input file containing invalid sequence should be written to a file named invalid.txt. Each line from the input file must be written to a separate line to the respective output files. Each line in the input file will be composed of exactly two sequences separated by any number of white spaces. Valid lines will be composed of 1 to 5 digits followed by one or more spaces followed by one or two letters without any spaces between digits or letters. Any line that does not follow this requirement may be considered invalid and included as output to file invalid.txt. after shown appropriate message as described below in the exception handling section. Table A below shows example sequences and their validity for car plates. Example Sequence Validity 12 A Valid 123 BB Valid 10 BZ Invalid (BZ is not a valid letter combination) 00 R Invalid (cannot start with zero digit) Invalid (missing digits) Invalid (more than 5 digits) MM 123456 B 1110 Y Valid 9 AAA Invalid (AAA is not a valid letter combination) Table A Example valid/invalid sequences. Table B below shows example input file plates.txt and the corresponding expected content in the output files. plates.txt 11 A valid.txt invalid.txt 11 A OOB OOB 12 YY 222 12 YY 87652 M 500111 FA D 999 I0)&0123456 87652 M 34091 D 222 34091 D 500111 FA D 999 ,10)&0123456 Table B Example input file and expected output files. Exception Handling. Your program must catch and handle exceptions where appropriate, for instance, when reading and writing files in addition to any other python runtime errors such as ValueError and IOError, including any other potential errors that may emerge from your code.
Problem Definition Task. Your task is to develop a python program that reads input from text file and finds if the alphanumeric sequence in each line of the provided input file is valid for Omani car license plate. The rules for valid sequences for car plates in Oman are as follows: Each sequence is composed of 1 to 5 digits followed by one or two letters. Digits cannot start with 0, for instance, 00, 011, or 09 are not valid digit sequences. The following list are the only valid letter combinations: ['A','AA','AB','AD','AR','AM','AW",'AY', 'B','BA','BB','BD','BR','BM','BW','BY', 'D','DA','DD','DR','DW','DY', 'R', RA','RR','RM', RW','RY', 'S','SS', 'M",'MA','MB','MM', MW','MY', "W',WA', WB','WW', Y,YA','YB',YD', YR',YW',YY] Program Input/Output. Your program should read input from a file named plates.txt and write lines with valid sequences to a file named valid.txt. Any line from the input file containing invalid sequence should be written to a file named invalid.txt. Each line from the input file must be written to a separate line to the respective output files. Each line in the input file will be composed of exactly two sequences separated by any number of white spaces. Valid lines will be composed of 1 to 5 digits followed by one or more spaces followed by one or two letters without any spaces between digits or letters. Any line that does not follow this requirement may be considered invalid and included as output to file invalid.txt. after shown appropriate message as described below in the exception handling section. Table A below shows example sequences and their validity for car plates. Example Sequence Validity 12 A Valid 123 BB Valid 10 BZ Invalid (BZ is not a valid letter combination) 00 R Invalid (cannot start with zero digit) Invalid (missing digits) Invalid (more than 5 digits) MM 123456 B 1110 Y Valid 9 AAA Invalid (AAA is not a valid letter combination) Table A Example valid/invalid sequences. Table B below shows example input file plates.txt and the corresponding expected content in the output files. plates.txt 11 A valid.txt invalid.txt 11 A OOB OOB 12 YY 222 12 YY 87652 M 500111 FA D 999 I0)&0123456 87652 M 34091 D 222 34091 D 500111 FA D 999 ,10)&0123456 Table B Example input file and expected output files. Exception Handling. Your program must catch and handle exceptions where appropriate, for instance, when reading and writing files in addition to any other python runtime errors such as ValueError and IOError, including any other potential errors that may emerge from your code.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
![Problem Definition
Task. Your task is to develop a python program that reads input from text file and finds if the
alphanumeric sequence in each line of the provided input file is valid for Omani car license plate.
The rules for valid sequences for car plates in Oman are as follows:
Each sequence is composed of 1 to 5 digits followed by one or two letters.
Digits cannot start with 0, for instance, 00, 011, or 09 are not valid digit sequences.
The following list are the only valid letter combinations:
['A','AA','AB','AD','AR','AM','AW",'AY"',
'B', BA','BB','BD',"BR','BM',"BW',"BY',
'D','DA','DD','DR','DW','DY',
'R','RA','RR','RM','RW','RY',
'S','SS',
'M','MA','MB','MM','MW','MY",
"W',"WA',WB',"wW',
Y,YA','YB','YD','YR','YW',YY]
Program Input/Output. Your program should read input from a file named plates.txt and write
lines with valid sequences to a file named valid.txt. Any line from the input file containing invalid
sequence should be written to a file named invalid.txt. Each line from the input file must be
written to a separate line to the respective output files.
Each line in the input file will be composed of exactly two sequences separated by any number
of white spaces. Valid lines will be composed of 1 to 5 digits followed by one or more spaces
followed by one or two letters without any spaces between digits or letters. Any line that does
not follow this requirement may be considered invalid and included as output to file invalid.txt.
after shown appropriate message as described below in the exception handling section. Table
A below shows example sequences and their validity for car plates.
Example Sequence
Validity
12 A
Valid
123 BB
Valid
10 BZ
Invalid (BZ is not a valid letter combination)
00 R
Invalid (cannot start with zero digit)
Invalid (missing digits)
Invalid (more than 5 digits)
MM
123456 B
1110 Y
Valid
9 AAA
Invalid (AAA is not a valid letter combination)
Table A Example valid/invalid sequences.
Table B below shows example input file plates.txt and the corresponding expected content in
the output files.
plates.txt
11 A
valid.txt
invalid.txt
11 A
OOB
OOB
12 YY
222
12 YY
87652 M
500111 FA
D 999
„IO)&0123456
87652 M
34091 D
222
34091 D
500111
FA
D 999
„10)&0123456
Table B Example input file and expected output files.
Exception Handling. Your program must catch and handle exceptions where appropriate, for
instance, when reading and writing files in addition to any other python runtime errors such as
ValueError and IOError, including any other potential errors that may emerge from your code.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F1efb34e5-a6de-4cde-b5f0-a9035a4538f5%2Fdd2c954c-1d98-4182-ba6c-238bf721fb03%2Fjs4itee_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Problem Definition
Task. Your task is to develop a python program that reads input from text file and finds if the
alphanumeric sequence in each line of the provided input file is valid for Omani car license plate.
The rules for valid sequences for car plates in Oman are as follows:
Each sequence is composed of 1 to 5 digits followed by one or two letters.
Digits cannot start with 0, for instance, 00, 011, or 09 are not valid digit sequences.
The following list are the only valid letter combinations:
['A','AA','AB','AD','AR','AM','AW",'AY"',
'B', BA','BB','BD',"BR','BM',"BW',"BY',
'D','DA','DD','DR','DW','DY',
'R','RA','RR','RM','RW','RY',
'S','SS',
'M','MA','MB','MM','MW','MY",
"W',"WA',WB',"wW',
Y,YA','YB','YD','YR','YW',YY]
Program Input/Output. Your program should read input from a file named plates.txt and write
lines with valid sequences to a file named valid.txt. Any line from the input file containing invalid
sequence should be written to a file named invalid.txt. Each line from the input file must be
written to a separate line to the respective output files.
Each line in the input file will be composed of exactly two sequences separated by any number
of white spaces. Valid lines will be composed of 1 to 5 digits followed by one or more spaces
followed by one or two letters without any spaces between digits or letters. Any line that does
not follow this requirement may be considered invalid and included as output to file invalid.txt.
after shown appropriate message as described below in the exception handling section. Table
A below shows example sequences and their validity for car plates.
Example Sequence
Validity
12 A
Valid
123 BB
Valid
10 BZ
Invalid (BZ is not a valid letter combination)
00 R
Invalid (cannot start with zero digit)
Invalid (missing digits)
Invalid (more than 5 digits)
MM
123456 B
1110 Y
Valid
9 AAA
Invalid (AAA is not a valid letter combination)
Table A Example valid/invalid sequences.
Table B below shows example input file plates.txt and the corresponding expected content in
the output files.
plates.txt
11 A
valid.txt
invalid.txt
11 A
OOB
OOB
12 YY
222
12 YY
87652 M
500111 FA
D 999
„IO)&0123456
87652 M
34091 D
222
34091 D
500111
FA
D 999
„10)&0123456
Table B Example input file and expected output files.
Exception Handling. Your program must catch and handle exceptions where appropriate, for
instance, when reading and writing files in addition to any other python runtime errors such as
ValueError and IOError, including any other potential errors that may emerge from your code.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 5 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
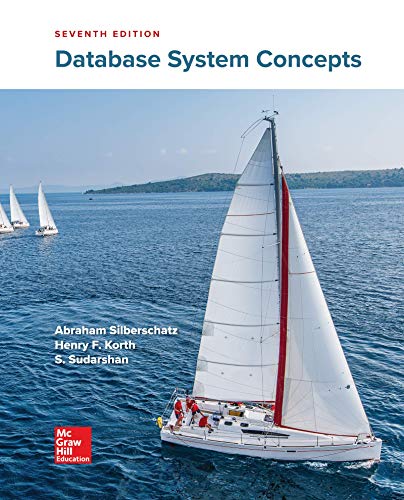
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
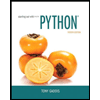
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
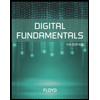
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
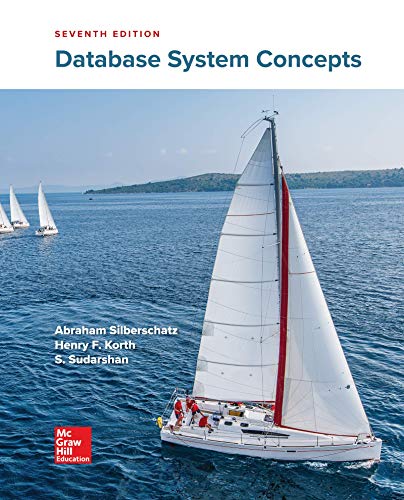
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
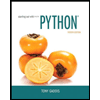
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
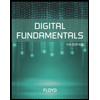
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
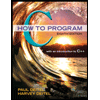
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
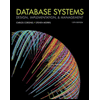
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
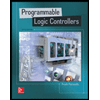
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education