Problem For this project, you will use the Fraction class I have given you (Fraction.h and Fraction.cpp) to create and use Fractions in your driver. Your main program will use the Fraction data type to create a calculator. This calculator should give the user the ability to specify operations and input operands (Fractions). Your program should make use of all methods and friend functions of the Fraction class. Do not change the Fraction class in any way.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Fraction.h:
#ifndef _FRACTION_H
#define _FRACTION_H
#include <iostream>
using namespace std;
class Fraction{
int num;
int den;
public:
Fraction();
Fraction(int n);
Fraction(int n, int d);
void reduce();
int GetNum()const;
int GetDen()const;
void SetNum(int n);
void SetDen(int d);
Fraction operator+(const Fraction&)const;
Fraction operator-(const Fraction&)const;
Fraction operator*(const Fraction&)const;
Fraction operator/(const Fraction&)const;
Fraction operator++();
Fraction operator++(int);
friend Fraction operator--(Fraction&);
friend Fraction operator--(Fraction&, int);
friend ostream& operator<<(ostream&, const Fraction&);
friend istream& operator>>(istream&, Fraction&);
friend bool isExact(const Fraction&, const Fraction&); //checks exact
values
friend bool operator == (const Fraction&, const Fraction&);//checks
equivalency
friend bool operator < (const Fraction&, const Fraction&);
friend bool operator > (const Fraction&, const Fraction&);
friend bool operator <= (const Fraction&, const Fraction&);
friend bool operator >= (const Fraction&, const Fraction&);
friend bool operator != (const Fraction&, const Fraction&);
};
#endif
Fraction.cpp:
#include "Fraction.h"
// Author: Dr. Janet T. Jenkins
// File: Fraction.cpp
// For CS 255 Project 1
// Fraction class implementation
// Do not alter for project
//*******************************************************
Fraction:: Fraction():num(0), den(1){}
//*******************************************************
Fraction:: Fraction(int n):num(n), den(1){}
//*******************************************************
Fraction::Fraction(int n, int d):num(n){
if (d == 0)
d = 1;
den = d;
}
//*******************************************************
int gcd(int one, int two){
while( one != 0 ){
int temp = one;
one = two % one;
two = temp;
}
return two;
}
//*******************************************************
void Fraction::reduce(){
int divisor = gcd(num,den);
num/=divisor;
den/=divisor;
}
//*******************************************************
int Fraction::GetNum()const{
return num;
}
//*******************************************************
int Fraction::GetDen()const{
return den;
}
//*******************************************************
void Fraction::SetNum(int n){
num = n;
}
//*******************************************************
void Fraction::SetDen(int d){
if (d==0)
d = 1;
//*******************************************************
Fraction Fraction::operator+(const Fraction& f)const{
Fraction temp;
temp.num = num*f.den + den * f.num;
temp.den = den * f.den;
return temp;
}
//*******************************************************
Fraction Fraction::operator-(const Fraction& f)const{
Fraction temp;
temp.num = num*f.den - den * f.num;
temp.den = den * f.den;
return temp;
}
//*******************************************************
Fraction Fraction::operator*(const Fraction& f)const{
Fraction temp;
temp.num = num * f.num;
temp.den = den * f.den;
return temp;
}
//*******************************************************
Fraction Fraction::operator/(const Fraction& f)const{
Fraction temp;
temp.num = num * f.den;
temp.den = den * f.num;
return temp;
}
//*******************************************************
ostream& operator<<(ostream& os, const Fraction& f){
os << f.num << "/" << f.den ;
return os;
}
//*******************************************************
istream& operator>>(istream& is, Fraction& f){
char dummy;
is >> f.num >> dummy >> f.den;
return is;
}
//*******************************************************
bool isExact(const Fraction& one , const Fraction& two){
return one.num == two.num && one.den == two.den;
}
//*******************************************************
bool operator == (const Fraction& one, const Fraction& two){
int left = one.num * two.den;
int right = two.num * one. den;
return left == right;
}
bool operator < (const Fraction& one, const Fraction& two){
int left = one.num * two.den;
int right = two.num * one. den;
return left < right;
}
//*******************************************************
bool operator > (const Fraction& one, const Fraction& two){
return !(one == two || one < two);
}
//*******************************************************
bool operator <= (const Fraction& one, const Fraction& two){
return (one == two || one < two);
}
bool operator >= (const Fraction& one, const Fraction& two){
return (one == two || one > two);
}
bool operator != (const Fraction& one, const Fraction& two){
return !(one==two);
}
//*******************************************************
Fraction Fraction::operator++(){ // prefix
num+=den;
return *this;
}
//*******************************************************
Fraction Fraction::operator++(int){
Fraction temp;
temp = *this;
num +=den;
return temp;
}
//*******************************************************
Fraction operator--(Fraction& f){
f.num-=f.den;
return f;
}
//*******************************************************
Fraction operator--(Fraction& f, int){
Fraction temp = f;
f.num -= f.den;
return temp;


Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 3 images

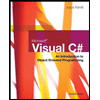
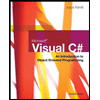