Prompt We have learned how to use accessor and mutator methods to access private class member data. It is possible to instead make the class members public, which would allow other programs to directly retrieve and modify the class member data without needing to write the accessor and mutator methods. This is usually considered bad practice. Why do you think that is? What do you think the benefits are to writing accessor and mutator methods instead of just leaving the variables public? Below is the definition for a class called Counter. Define a new method for this class called "findDifference". This method should take another Counter object as an argument and return the difference in the counts between the counter being called and the one passed as an argument. The difference should be given as an absolute value (not returned as a negative). See below the class definition for examples of this method being used. public class Counter { private int count; public Counter() { count = 0; } public int countUp() { count++; return count; } public int getCount() { return count; } } Examples of findDifference: Counter "a" has count 5. Counter "b" has count 10. Counter "myCount" has count 200. Counter "yourCount" has count 0. a.findDifference(b); // This returns 5 b.findDifference(a); // This also returns 5 myCount.findDifference(yourCount); // This returns 200 yourCount.findDifference(b); // This returns 10 b.findDifference(myCount); // This returns 190
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Prompt
- We have learned how to use accessor and mutator methods to access private class member data. It is possible to instead make the class members public, which would allow other
programs to directly retrieve and modify the class member data without needing to write the accessor and mutator methods. This is usually considered bad practice. Why do you think that is? What do you think the benefits are to writing accessor and mutator methods instead of just leaving the variables public? - Below is the definition for a class called Counter. Define a new method for this class called "findDifference". This method should take another Counter object as an argument and return the difference in the counts between the counter being called and the one passed as an argument. The difference should be given as an absolute value (not returned as a negative). See below the class definition for examples of this method being used.
public class Counter {
private int count;
public Counter() {
count = 0;
}
public int countUp() {
count++;
return count;
}
public int getCount() {
return count;
}
}
Examples of findDifference:
Counter "a" has count 5. Counter "b" has count 10. Counter "myCount" has count 200. Counter "yourCount" has count 0.
a.findDifference(b); // This returns 5
b.findDifference(a); // This also returns 5
myCount.findDifference(yourCount); // This returns 200
yourCount.findDifference(b); // This returns 10
b.findDifference(myCount); // This returns 190

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

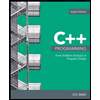
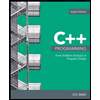