program in C ++ which enables the addition of elements in a vector dynamically and the calculation of the sum of the elements of the vector using a function. Use classes, create objects, call functions and validate from the main function

Program Approach-
- Include the header file.
- Declare the integer type vector named myvector.
- Declare the class named useofVector with class variable number, data2 and class functions insertElementinVector(), addVectorElement().
- Define insertElementinVector(int n) function to prompt the user to enter the elements for myvector. Use for loop to insert the elements in the vector.
- Define the addVectorElement() function to calculate the sum of the elements of myvector and print the result.
- Define the main function.
- Create the object of the class useofVector named v1 and prompt the user to enter the number of elements that he wants to insert in the vector.
- Call the insertElementinVector() and addVectorElement() functions to provide the required result.
Code ( in C ++) with comments :–
//include header file
#include <bits/stdc++.h>
//use namespace
using namespace std;
//declare the integer type vector named myvector
vector<int> myvector;
//declare the global var
int intElement;
//declare the class named useofVector
class useofVector
{
//class variables
private:
int number;
int data2;
//class functions
public:
//function to insert the elements in myvector dynaically
void insertElementinVector(int n)
{
number =n;
cout << "\nEnter elements for Vector: ";
//for loop
for(int i = 0 ; i< number ; i++)
{
cin >> intElement;
//insert the elements in myvector
myvector.push_back(intElement);
}
}
//function to calculate the sum of the elements of myvector
void addVectorElement()
{
cout << "\nSum = " << accumulate(myvector.begin(), myvector.end(), 0);
}
};
//main function
int main()
{
int n;
//create class object
useofVector v1;
//prompt the user to enter the number
cout<<"Enter how many number you want to insert in the vector"<<endl;
cin>>n;
//call the functions
v1.insertElementinVector(n);
v1.addVectorElement();
return 0;
}
Step by step
Solved in 4 steps with 3 images

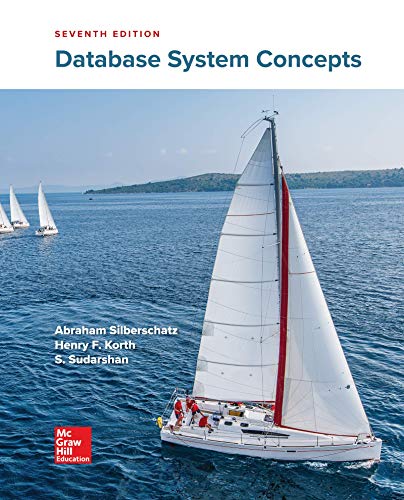
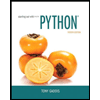
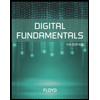
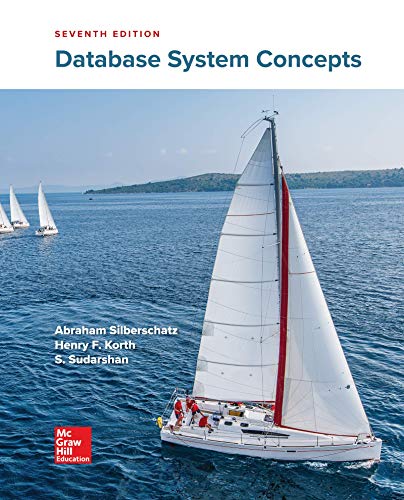
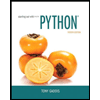
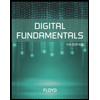
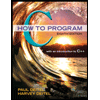
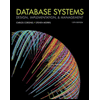
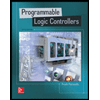