Program Specifications Write a function called rCopy that copies the entire contents of one file to another, but copies them over in reverse order. This function should take the names of the input and output files as strings. If either file does not open for any reason, the function should output an error message stating the name of the file that didn't open and then return the value false. Otherwise, if both files open correctly, the function should return true once the reverse copy has been completed. For example, if a file named in.dat contains the following: A c d 87 arc 6587 9 9 then after the function call: rCopy("in.dat", "out.dat") the file out.dat should contain the following: 9 9 7856 cra 78 d c A You must solve this recursively!! To implement this recursively you will need to write a helper function that passes in an input and an output file stream. This helper function will be the recursive function. Recall that the expression cin.get(ch) will read in a single character from cin into a char variable named ch. Again, this must use recursion to get any points. You may NOT use any kind of loop. You may NOT use global or static variables. You will be docked at least 20 points if you use a loop, a global variable, or a static variable.
Program Specifications Write a function called rCopy that copies the entire contents of one file to another, but copies them over in reverse order. This function should take the names of the input and output files as strings. If either file does not open for any reason, the function should output an error message stating the name of the file that didn't open and then return the value false. Otherwise, if both files open correctly, the function should return true once the reverse copy has been completed. For example, if a file named in.dat contains the following: A c d 87 arc 6587 9 9 then after the function call: rCopy("in.dat", "out.dat") the file out.dat should contain the following: 9 9 7856 cra 78 d c A You must solve this recursively!! To implement this recursively you will need to write a helper function that passes in an input and an output file stream. This helper function will be the recursive function. Recall that the expression cin.get(ch) will read in a single character from cin into a char variable named ch. Again, this must use recursion to get any points. You may NOT use any kind of loop. You may NOT use global or static variables. You will be docked at least 20 points if you use a loop, a global variable, or a static variable.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
The coding language is c++
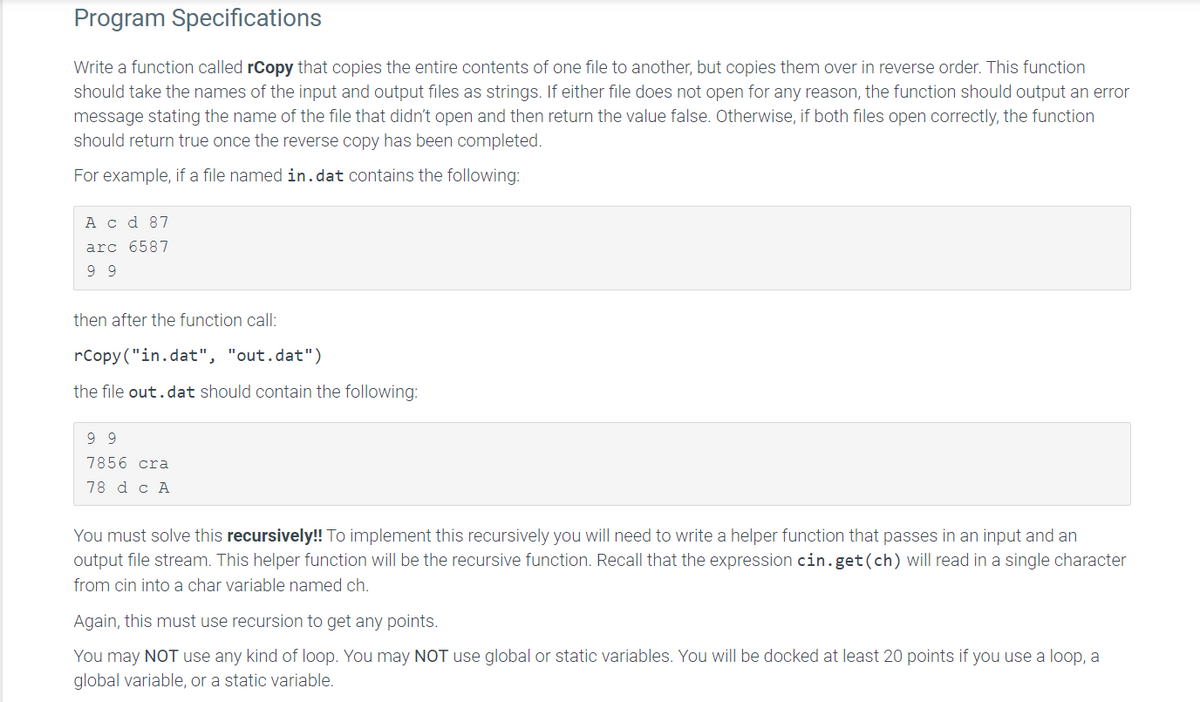
Transcribed Image Text:Program Specifications
Write a function called rCopy that copies the entire contents of one file to another, but copies them over in reverse order. This function
should take the names of the input and output files as strings. If either file does not open for any reason, the function should output an error
message stating the name of the file that didn't open and then return the value false. Otherwise, if both files open correctly, the function
should return true once the reverse copy has been completed.
For example, if a file named in.dat contains the following:
A c d 87
arc 6587
9 9
then after the function call:
rCopy("in.dat", "out.dat")
the file out.dat should contain the following:
9 9
7856 cra
78 d c A
You must solve this recursively!! To implement this recursively you will need to write a helper function that passes in an input and an
output file stream. This helper function will be the recursive function. Recall that the expression cin.get(ch) will read in a single character
from cin into a char variable named ch.
Again, this must use recursion to get any points.
You may NOT use any kind of loop. You may NOT use global or static variables. You will be docked at least 20 points if you use a loop, a
global variable, or a static variable.
![#include <iostream>
#include <fstream>
using namespace std;
void rCopy(istream &, ostream &);
bool rCopy(const string &, const string &);
int main(int argc, char *argv[]) {
if (argc != 3) {
cout << "USAGE:
<« argv[0] <
input-file output-file" << endl;
return 1;
}
if (rCopy(argv[1], argv[2])) {
cout <« "Copy completed" <« endl;
} else {
cout <« "Copy not completed" <« endl;
}
return 0;
}
// Implement these functions
void rCopy(istream &in, ostream &out) {
}
bool rCopy(const string &in, const string &out) {
return true;
}](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F75456869-7480-46ae-9c35-9bbc61c69e85%2F242d126f-d2c0-4fd8-b33a-adee00fc3d9c%2F1069b2b_processed.png&w=3840&q=75)
Transcribed Image Text:#include <iostream>
#include <fstream>
using namespace std;
void rCopy(istream &, ostream &);
bool rCopy(const string &, const string &);
int main(int argc, char *argv[]) {
if (argc != 3) {
cout << "USAGE:
<« argv[0] <
input-file output-file" << endl;
return 1;
}
if (rCopy(argv[1], argv[2])) {
cout <« "Copy completed" <« endl;
} else {
cout <« "Copy not completed" <« endl;
}
return 0;
}
// Implement these functions
void rCopy(istream &in, ostream &out) {
}
bool rCopy(const string &in, const string &out) {
return true;
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Recommended textbooks for you
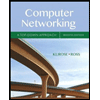
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
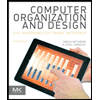
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
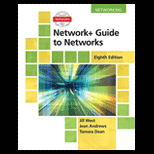
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
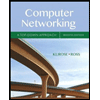
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
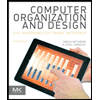
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
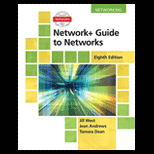
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
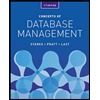
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
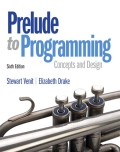
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
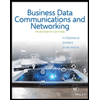
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY