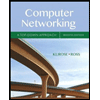
Using C
Write a program that reads a text file, and calculates the frequency distribution of individual letters in the file.
Upper and lower case letters are considered the same. Numbers and punctuation are ignored.
The frequency value should be the percentage that particular letter occurs in relation to all letters. Thus, the output will have two columns, each row will show the letter, and it's frequency (neatly formatted).
Your program should prompt for the file name, and handle the case where the file isn't found.
Use at least one user defined function (even if it's trivial) that's defined in a separate file, and use a header file that contains the declaration.

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 2 images

- The Clay Hill Golf Club has a tournament every weekend. the club president ask you to write two programs: 1. a program that will read each player's name and golf score as keyboard input, then save those records in a file named golf.txt. (each record will have a field for the player name and five rounds of golf scores for each golfer.) allow for any number of players to be input. 2. a program that reads the records from the golf.txt file and averages the scores, calculates the golfer's handicaparrow_forwardWrite a program to read the date and average from the temps.txt file. Use a string data type for the date and a doub e average. The program should then convert the average (Fahrenheit) to Celsius. The conversion formula is subtract 32 from the average (Fahrenheit) and then multiply by five and divide by nine, e. 32) * 5/9. Output the date, the average temperature (Fahrenheit) and the Celsius temperature to a file named celsi Use the manipulators you have learned about in this chapter to format the output as shown in the sample be low and print each number value to one decimal space. 1. Identify the following parameters to complete the specification above. Input parameters? Output parameters? Constant parameters? 2. Attach the input and output of a successful execution of your program below (Hint: Use the snipping tool to take screenshots):arrow_forwardProgram Input The input file consists of blocks of lines, each of which is a test case. Each block except the last describes one train and possibly more requirements for its reorganization. In the first line of the block there is the integer , which is the number of coaches in the train. In each of the next lines of the block there is a permutation of 1, 2, ..., N . For example, if N is 5, and the permutation could be 5, 3, 2, 1, 4. Your program will take this permutation as input and determine whether you can marshal the coaches from track A an incoming order 1, 2, 3, 4, 5 to track B with an outgoing order 5, 3, 2, 1, 4 using the station, which can be treated as a stack.The last line of the block contains just 0.If a block starts with a zero, the program will terminate. You should use the input file named lab1in.txt (download from Canvas) to test your program; an output file named lab1out.txt (with correct output) is also provided for you to verify your program.Input Sample 5…arrow_forward
- The GTUC Company wants to produce a product orders report from its product orders file. Each record on the file contains the product number of the item ordered, the product description, the number of units ordered, the retail price per unit, the freight charges per unit, and the packaging costs per unit. Your algorithm is to read the product orders file, calculate the total amount due for each product ordered and print these details on the product orders report.The amount due for each product is calculated as the product of the number of units ordered and the retail price of the unit. A discount of 10% is allowed on the amount due for all orders over 100.00. The freight charges and packaging costs per unit must be added to this resulting value to determine the total amount due.arrow_forwardYou are tasked with writing a program to process students’ end of semester marks. For each student you must enter into your program the student’s id number (an integer), followed by their mark (an integer out of 100) in 4 courses. A student number of 0 indicates the end of the data. A student moves on to the next semester if the average of their 4 courses is at least 60 (greater than or equal to 60). Write a program to read the data and print, for each student: The student number, the marks obtained in each course, final mark of the student (average of all the marks), their final grade (A,B,C,D or F) and whether or not they move on to the next semester. In addition, after all data entry is completed, print The number of students who move on to the next semester and the number who do not. The student with the highest final mark (ignore the possibility of a tie). Grades are calculated as follows: A = 70 and over, B = 60 (inclusive) -70, C = 50(inclusive)-60 D =…arrow_forwardTask. Your task is to develop a python program that reads input from text file and finds if the alphanumeric sequence in each line of the provided input file is valid for Omani car license plate. The rules for valid sequences for car plates in Oman are as follows: Each sequence is composed of 1 to 5 digits followed by one or two letters. Digits cannot start with 0, for instance, 00, 011, or 09 are not valid digit sequences. The following list are the only valid letter combinations: ['A','AA','AB','AD','AR','AM','AW','AY', 'B','BA','BB','BD','BR','BM','BW','BY', 'D',DA','DD','DR','DW','DY', "R',RA','RR','RM','RW','RY', 'S','SS', 'M', 'MA','MB','MM','MW','MY', W',WA','WB','ww, Y,YA','YB','YD','YR','YW','YY'] Program Input/Output. Your program should read input from a file named plates.txt and write lines with valid sequences to a file named valid.txt. Any line from the input file containing invalid sequence should be written to a file named invalid.txt. Each line from the input file must…arrow_forward
- Can you help me write a C++ Program that does the following: Create a program that reads a file containing a list of songs andprints the songs to the screen one at a time. After each song is printed,except for the last song, the program asks the user to press enter for more.After the last song, the program should say that this was the last song andquit. If there were no songs in the file to begin with, the program shouldsay that there are no songs to show and quit.The program should begin by asking the user for the name of the input file.Each song consists of a title, artist and year.In the file, each song is given on three consecutive lines.Create this program using the latest version of the class Song you created. The latest version of the class song I created is below: #include <iostream>#include <string>using namespace std;class Song {private: string Title; string Artist; int Year;public: Song() : Title("invalid"), Artist("invalid"), Year(-1) {} Song(const string…arrow_forwardThe objective of this problem is to show that you can write a program that reads a text file and uses string methods to manipulate the text. File cart.txt contains shopping cart type data from golfsmith.com representing the gifts you have purchased for your dad for this past Fathers Day. Good for you. You have been very generous! The first item in each row of the file is the part number, the second the quantity, the third the price and the fourth the description. Your program will read the file line by line and compute the total cost of all of the items in the list. You will then display a message that looks like this, assuming the cost of each item adds up to 168.42: Here's what your output should look like: The total price for the awesome Fathers Day gifts you bought is $168.42. The shopping cart can be found here: cart.txt. Right click on the link and save the file to the folder where you have your Python files.arrow_forwardPYTHON: I need to get the avg statement out of the loop so that the output prints correctly. Any suggestions. Write a program that reads the student information from a tab separated values (tsv) file. The program then creates a text file that records the course grades of the students. Each row of the tsv file contains the Last Name, First Name, Midterm1 score, Midterm2 score, and the Final score of a student. A sample of the student information is provided in StudentInfo.tsv. Assume the number of students is at least 1 and at most 20. The program performs the following tasks: Read the file name of the tsv file from the user. Open the tsv file and read the student information. Compute the average exam score of each student. Assign a letter grade to each student based on the average exam score in the following scale: A: 90 =< x B: 80 =< x < 90 C: 70 =< x < 80 D: 60 =< x < 70 F: x < 60 Compute the average of each exam. Output the last names, first names, exam…arrow_forward
- Java Your program must read a file called personin.txt. Each line of the file will be a person's name, the time they arrived at the professor's office, and the amount of time they want to meet with the professor. These entries will be sorted by the time the person arrived. Your program must then print out a schedule for the day, printing each person's arrival, and printing when each person goes in to meet with the professor. You need to print the events in order of the time they happen. In other words, your output will be sorted by the arrival times and the times the person goes into the professor's office. In your output you need to print out a schedule. In the schedule, new students go to the end of the line. Whenever the professor is free, the professor will either meet with the first person in line, or meet with the first person in line if nobody is waiting. Assume no two people arrive at the same time. You should solve this problem using a stack and a queue. You can only…arrow_forwardIn Java Write a program that prints the 128-character ASCII table. It should print the tablein eight tab-separated columns. The first column contains a "Dec" heading (fordecimal number) with the numbers 0 through 31 below it. The second columncontains the ASCII characters associated with the numbers 0 through 31. The nextcolumns contain the subsequent numbers and associated ASCII characters. See thebelow sample output for details. Be aware that that output was produced by aprogram running in a console window in a Windows environment. If you runyour program in a different environment, the first 32 characters and the lastcharacter will probably be different from what’s shown below.Note that some characters display in a non-standard manner. For example, thenumber 7 corresponds to a bell sound. You can’t see a sound, but you can see thevacant spot for 7’s character in the below table. The number 8 corresponds to thebackspace character. You can’t see a backspace, but you can see how the…arrow_forwardPlease help me with this question to built it in python. Your company keeps a list of employee information for each pay period in a text file. The format of each line of the file is following: <name>, <rate>, <hours worked>. Write a program that inputs a file from the user and prints to the terminal a report of the wages paid to the employees for the given period. The report should be in tabular format with the appropriate header. Each line should contain employee's name, the hours worked, and the wages paid for that period.arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
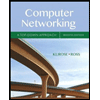
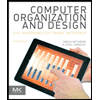
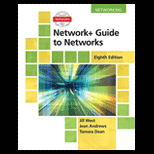
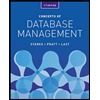
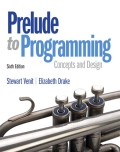
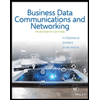