Programming Fundamentals II JAVA Lab #7-Inheritance NAME Problem: Develop the 'Shape' application such that: 'Rectangle', 'Ellipse', and 'Triangle' classes inherit from the 'Shape' class Develop the 'Square' and 'Circle' class where 'Square' inherits from 'Rectangle' and Circle' inherits from Ellipse'. 'Triangle' has no derived class. For each class, implement the overridden methods 'draw', 'move', and 'erase'. Each method should only have an output statement such as "Rectangle draw method" that will be displayed when the method is invoked Implement the default constructors for each class with a corresponding message to be displayed when invoked. No initializations are required; that is, the output message will be the only executable statement in the constructors Do not implement any other methods for these classes ( i.e., 'toString', 'equals', getters and setters ) Implement a 'ShapeTest' class which will instantiate an object of each class . Exercise each of the 'draw', 'move', and 'erase' methods of each class Remember to make sure that there is only a single class per file.
Develop the ‘Shape’ application such that:
‘Rectangle’, ‘Ellipse’, and ‘Triangle’ classes inherit from the ‘Shape’ class.
Develop the ‘Square’ and ‘Circle’ class where ‘Square’ inherits from ‘Rectangle’ and
‘Circle’ inherits from ‘Ellipse’. ‘Triangle’ has no derived class.
For each class, implement the overridden methods ‘draw’, ‘move’, and ‘erase’. Each
method should only have an output statement such as “Rectangle – draw method”
that will be displayed when the method is invoked.
Implement the default constructors for each class with a corresponding message to
be displayed when invoked. No initializations are required; that is, the output
message will be the only executable statement in the constructors.
Do not implement any other methods for these classes ( i.e., ‘toString’, ‘equals’,
getters and setters ).
Implement a ‘ShapeTest’ class which will instantiate an object of each class.
Exercise each of the ‘draw’, ‘move’, and ‘erase’ methods of each class
Remember to make sure that there is only a single class per file.
-----------------------------------------------------------------------------------
- 4 classes ( one of them is a default constructor)
-no initialization
-no instance data
-no tostring
-no return
-no arguments
only one output
-----------------------------------------------------------------------------------


Trending now
This is a popular solution!
Step by step
Solved in 10 steps with 8 images

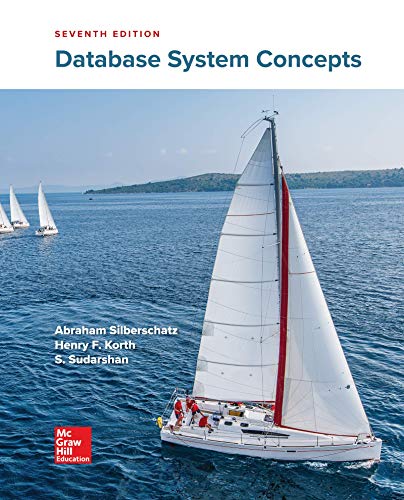
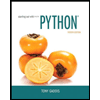
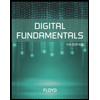
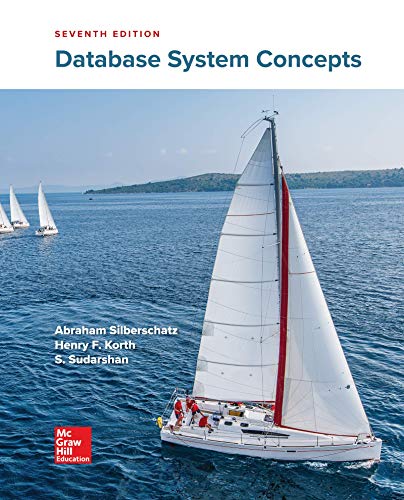
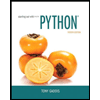
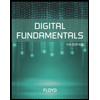
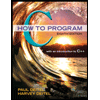
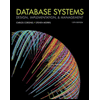
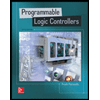