PROGRAMMING LANGUAGE: C++ Note: Kindly refer to the attached screenshot for the sample program. 1. Identify the format of the function used: no return type & no parameter, with return type & no parameter, no return type & with parameter and with return type & with parameter. 2. Identify the format and actual parameters. 3. Identify the function signature 4. Identify parameter if value parameter, reference parameter or constant reference parameter is used. 5. Identify the the scope of variable used. 6. Identify the type of C++ function is used.
PROGRAMMING LANGUAGE: C++ Note: Kindly refer to the attached screenshot for the sample program. 1. Identify the format of the function used: no return type & no parameter, with return type & no parameter, no return type & with parameter and with return type & with parameter. 2. Identify the format and actual parameters. 3. Identify the function signature 4. Identify parameter if value parameter, reference parameter or constant reference parameter is used. 5. Identify the the scope of variable used. 6. Identify the type of C++ function is used.
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter13: Structures
Section: Chapter Questions
Problem 3PP
Related questions
Question
100%
Note: Kindly refer to the attached screenshot for the sample program.
1. Identify the format of the function used: no return type & no parameter, with return type & no parameter, no return type & with parameter and with return type & with parameter.
2. Identify the format and actual parameters.
3. Identify the function signature
4. Identify parameter if value parameter, reference parameter or constant reference parameter is used.
5. Identify the the scope of variable used.
6. Identify the type of C++ function is used.
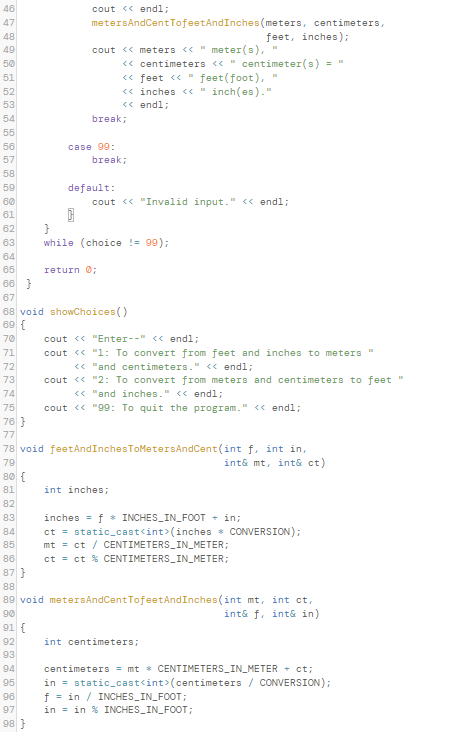
Transcribed Image Text:46
cout <« endl;
47
metersAndCentTofeetAndInches (meters, centimeters,
48
feet, inches);
49
cout << meters <«
meter(s),
<« centimeters « " centimeter(s) = "
« feet <« " feet(foot),
<« inches <« " inch(es)."
<« endl;
50
51
52
53
54
break;
55
56
case 99:
57
break;
58
59
default:
60
cout <« "Invalid input." <« endl;
61
}
while (choice != 99);
62
63
64
65
return 0;
66 }
67
68 void showChoices ()
69 {
70
71
cout <« "Enter--" << endl;
cout <« "1: To convert from feet and inches to meters "
<« "and centimeters." << endl;
cout <« "2: To convert from meters and centimeters to feet "
<« "and inches." << endl;
cout <« "99: To quit the program." << endl;
72
73
74
75
76 }
77
78 void feetAndInchesToMetersAndCent (int f, int in,
79
80 {
int& mt, int& ct)
81
int inches;
82
inches = f * INCHES_IN_FOOT - in;
ct = static_cast<int>(inches * CONVERSION);
mt = ct / CENTIMETERS_IN_METER;
ct = ct % CENTIMETERS_IN_METER;
83
84
85
86
87 }
88
89 void metersAndCentTofeetAndInches (int mt, int ct,
90
91 {
int& f, int& in)
92
int centimeters;
93
centimeters = mt * CENTIMETERS_IN_METER + ct;
in = static_cast<int>(centimeters / CONVERSION);
f = in / INCHES_IN_FOOT;
in = in % INCHES_IN_FOOT;
94
95
96
97
98 }
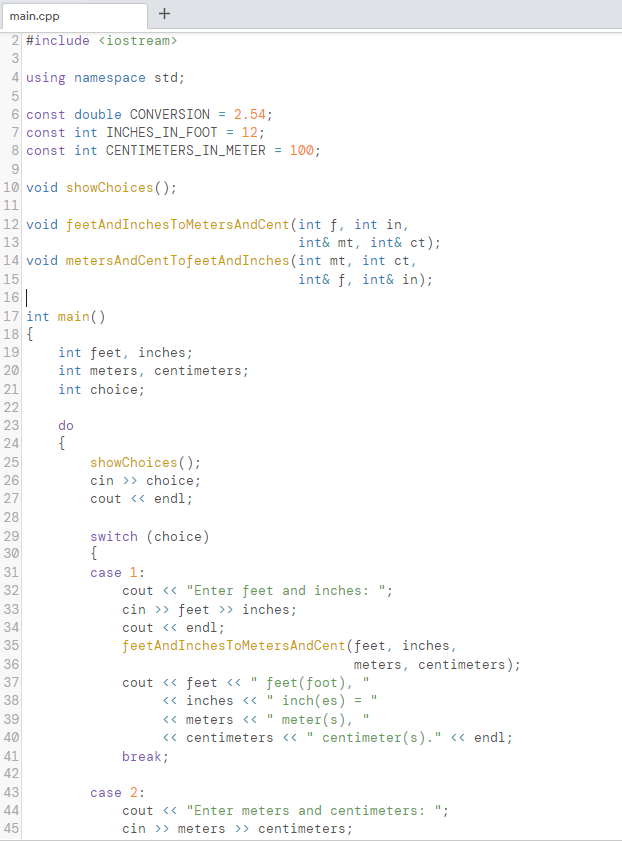
Transcribed Image Text:main.cpp
2 #include <iostream>
3
4 using namespace std;
5
6 const double CONVERSION = 2.54;
7 const int INCHES_IN_FOOT = 12;
8 const int CENTIMETERS_IN_METER = 100;
10 void showChoices ();
11
12 void feetAndInchesToMetersAndCent (int f, int in,
int& mt, int& ct);
14 void metersAndCentTofeetAndInches (int mt, int ct,
int& f, int& in);
13
15
16||
17 int main()
18 {
19
int feet, inches;
int meters, centimeters;
20
21
int choice;
22
23
do
24
{
25
showChoices ();
26
cin >> choice;
cout <« endl;
27
28
switch (choice)
{
29
30
31
case 1:
32
cout <« "Enter feet and inches: ";
33
cin >> feet > inches;
34
cout <« endl;
35
feetAndInches TOMetersAndCent(feet, inches,
36
meters, centimeters);
cout <« feet « "
<« inches <«
<« meters <«
feet (foot),
" inch(es)
meter(s),
« centimeters « " centimeter(s)." <« endl;
37
38
39
40
41
break;
42
43
case 2:
44
cout <« "Enter meters and centimeters: ";
cin >> meters >> centimeters;
45
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
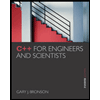
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
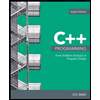
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
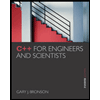
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
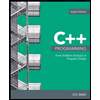
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning