Topic: A function with parameters passed by value and passed by reference Do not use anything beyond Chapter 6 materials. Write a C++ Write a single function rectangleAreaPerim (width, height, area, perimeter) that calculates both the area and the perimeter of a rectangle given its width and height. -Input parameters to the functions are the width and height of the rectangle. Function cannot modify the input parameters. -Output parameters of the function are the area, perimeter, and a boolean return (success or failure). -This function returns true if both width and height are not negative, and thus calculation is required. Otherwise the function returns false and skips all calculations. -The function rectangleAreaPerim(width, height, area, perimeter) should Not use any "cin" or "cout". Only the main() function will contain "cin" and "cout". -The main() function calls the function rectangleAreaPerim() in a loop until the user enters 0 0. These are "Sentinel" values that stop the loop (chapter 5). If only one input is 0, the loop keeps going. This is a basic simple code without loop to test your function: int main() { double width = 0, height = 0, area, perimeter; bool success = rectangleAreaPerim(width, height, area, perimeter); if (success) cout << "Area is " << area << ". Perimeter is " << perimeter << endl; else cout << "Invalid input" << endl; // Expected output is: // Area is 0. Perimeter is 0 } This does NOT mean you have to copy and paste the above code to your code for submission. It just gives you a guide of how to write the function prototype so that the test code above works correctly.. The result of the function is used in main() to display the correct output: true means the calculation result is stored in area and perimeter, and false means that the input parameters width, height are invalid. After using the simple test code above, write code in main() to accept multiple width, height inputs and print results by writing a Sentinel loop (chapter 5) that runs until the user enters 0 and 0 for the width and height. Write the function prototype before main() and write the full function definition after main(). Output sample This program calculates area and perimeter or rectangle. Enter width and height separated by spaces, or enter 0 0 to stop: 1.2 3.4 Rectangle area is 4.08 Rectangle perimeter is 9.2 Enter width and height separated by spaces, or enter 0 0 to stop: 0 3.4 Rectangle area is 0 Rectangle perimeter is 6.8 Enter width and height separated by spaces, or enter 0 0 to stop: -1.2 3.4 Invalid input Enter width and height separated by spaces, or enter 0 0 to stop: 1.2 -3.4 Invalid input Enter width and height separated by spaces, or enter 0 0 to stop: -1.2 -3.4 Invalid input Enter width and height separated by spaces, or enter 0 0 to stop: 0 0 Goodbye
Topic: A function with parameters passed by value and passed by reference
Do not use anything beyond Chapter 6 materials. Write a C++
Write a single function rectangleAreaPerim (width, height, area, perimeter) that calculates both the area and the perimeter of a rectangle given its width and height.
-Input parameters to the functions are the width and height of the rectangle. Function cannot modify the input parameters.
-Output parameters of the function are the area, perimeter, and a boolean return (success or failure).
-This function returns true if both width and height are not negative, and thus calculation is required. Otherwise the function returns false and skips all calculations.
-The function rectangleAreaPerim(width, height, area, perimeter) should Not use any "cin" or "cout". Only the main() function will contain "cin" and "cout".
-The main() function calls the function rectangleAreaPerim() in a loop until the user enters 0 0. These are "Sentinel" values that stop the loop (chapter 5). If only one input is 0, the loop keeps going.
This is a basic simple code without loop to test your function:
int main()
{
double width = 0, height = 0, area, perimeter;
bool success = rectangleAreaPerim(width, height, area, perimeter);
if (success)
cout << "Area is " << area << ". Perimeter is " << perimeter << endl;
else
cout << "Invalid input" << endl;
// Expected output is:
// Area is 0. Perimeter is 0
}
This does NOT mean you have to copy and paste the above code to your code for submission. It just gives you a guide of how to write the function prototype so that the test code above works correctly..
The result of the function is used in main() to display the correct output: true means the calculation result is stored in area and perimeter, and false means that the input parameters width, height are invalid.
After using the simple test code above, write code in main() to accept multiple width, height inputs and print results by writing a Sentinel loop (chapter 5) that runs until the user enters 0 and 0 for the width and height.
Write the function prototype before main() and write the full function definition after main().
Output sample
This program calculates area and perimeter or rectangle.
Enter width and height separated by spaces, or enter 0 0 to stop: 1.2 3.4
Rectangle area is 4.08
Rectangle perimeter is 9.2
Enter width and height separated by spaces, or enter 0 0 to stop: 0 3.4
Rectangle area is 0
Rectangle perimeter is 6.8
Enter width and height separated by spaces, or enter 0 0 to stop: -1.2 3.4
Invalid input
Enter width and height separated by spaces, or enter 0 0 to stop: 1.2 -3.4
Invalid input
Enter width and height separated by spaces, or enter 0 0 to stop: -1.2 -3.4
Invalid input
Enter width and height separated by spaces, or enter 0 0 to stop: 0 0
Goodbye

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

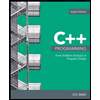
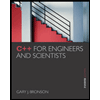
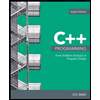
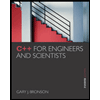