1- Write a Java class that models a Vehicle. There are three types of vehicles (Sedan, SUV, Truck). Every vehicle object should also contain an instance variable that can be used to store the time at which the vehicle has entered the car parking building (Hint: use the java.util.Date class to represent the time). In addition to the constructors, you should provide the following methods: public double getDuration (Date exit Time) this method will return the duration (in minutes) from the time the vehicle entered the parking until the given exit Time. public String getType() this method will return either Sedan or SUV or Truck based on the vehicle type.
1- Write a Java class that models a Vehicle. There are three types of vehicles (Sedan, SUV, Truck). Every vehicle object should also contain an instance variable that can be used to store the time at which the vehicle has entered the car parking building (Hint: use the java.util.Date class to represent the time). In addition to the constructors, you should provide the following methods: public double getDuration (Date exit Time) this method will return the duration (in minutes) from the time the vehicle entered the parking until the given exit Time. public String getType() this method will return either Sedan or SUV or Truck based on the vehicle type.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question

Transcribed Image Text:Sultan Qaboos University
Department of Computer Science
COMP2202: Fundamentals of Object Oriented Programming
Assignment # 2 (Due 5 November 2022 @23:59)
The purpose of this assignment is to practice with java classes and objects, and arrays.
Create a NetBeans/IntelliJ project named HW2_YourId to develop a java program as explained below.
Important: Apply good programming practices:
1- Provide comments in your code.
2- Provide a program design
3-
Use meaningful variables and constant names.
4- Include your name, university id and section number as a comment at the beginning of your code.
5- Submit to Moodle the compressed file of your entire project (HW1_YourId).
1. Introduction:
In crowded cities, it's very crucial to provide enough parking spaces for vehicles. These are usually multistory
buildings where each floor is divided into rows and columns. Drivers can park their cars in exchange for some
fees. A single floor can be modeled as a two-dimensional array of rows and columns. A building consists of
several floors. See Figure 1 for an illustration.
I
Figure 1: A Multistory car parking
2. To Do:
1- Write a Java class that models a Vehicle. There are three types of vehicles (Sedan, SUV, Truck). Every
vehicle object should also contain an instance variable that can be used to store the time at which the
vehicle has entered the car parking building (Hint: use the java.util.Date class to represent the time). In
addition to the constructors, you should provide the following methods:
public double getDuration(Date exitTime) this method will return the duration (in minutes) from
the time the vehicle entered the parking until the given exit Time.
public String getType() this method will return either Sedan or SUV or Truck based on the vehicle
type.
![public void setType(String type) this method will set the type of the vehicle to either Sedan or SUV
or Truck.
public void setEntryTime(Date entryTime) this method will set the time at which the vehicle has
entered the parking.
2- Write a CarParking class to model a multistory car parking building. This class should contain a 3-
dimensional array of type Vehicle. The third dimension of the array simply represents the number of
floors of the building while the first and second dimensions are the number of rows and columns in every
floor. By default, there are 3 floors, 7 rows and 7 columns in a building. However, the user should be able
to specify the maximum number of floors, rows and columns at the time of creating a CarParking object.
In addition to the constructors, this class should provide the following methods:
O
public boolean isOccupied (int floor, int row, int col) this method returns true if the location
specified by the floor, row and column is already occupied by a vehicle.
public void add (Vehicle v) this method will add the vehicle at the next empty slot. Slots are field
row by row (from left to right) starting from the ground floor.
public Vehicle remove (int floor, int row, int col, double rate) this method to removes the
vehicle at the specified location and returns the parking fee. The fee is calculated by multiplying
the rate by the duration (in minutes). Drivers will receive 5% discount per floor other than the
ground floor. If the location is not occupied, the method returns zero.
public double getTotalExpectedRevenue(double[] rates, Date exitTime) this method
calculates the total expected revenue if all cars exit the building at the given exitTime. The rates
array will contain an element for every vehicle type (Sedan, SUV, Truck).
public int getVehicleCount(String type, int floor) this method finds and returns the total number
of cars for a given type in a given floor.
O public double getRevenue (String type, int floor, double rate) this method finds and returns
the total revenue of a given car type in a given floor for a given rate.
public boolean canOrganizeByType() this method should return true if it is possible to
accommodate all cars of the same type in separate floors.
D
O
O
O
O
O
3- Write a Java program that uses the CarParking class and the Vehicle class to simulate a car parking
building. The program should start by constructing a CarParking object. The user should then be presented
with a menu to select one of the following actions:
Add a vehicle: This option constructs a vehicle object and adds it to the parking. The user should
be required to enter the vehicle type.
Remove a vehicle: This option removes a vehicle from the building and display its the parking
fees. The user should be prompted to enter the location and the rate.
Display statistics: this option will show a summary including:
●
i. Total expected revenue
ii. Number of sedans, SUVs and trucks per floor
iii. Revenue of sedans, SUVs and trucks per floor
iv. Number of empty slots per floor
Check arrangement: as a result of this selection the program should display one of the following
messages :
i. Cars can be arranged based on their type
ii. Not possible to rearrange cars based on their type.
Exit the program: This option will terminate the program
3. Hints:
1- Use the java.util.Date class to represent the time. This class contain several methods that allow you to
find for example the time difference in minutes between any two times.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F67d25ecd-3bd6-44ea-afb7-2e002c5a27b2%2Fcb5eeaef-376a-4134-a845-9c3121929a06%2Fomaufqj_processed.jpeg&w=3840&q=75)
Transcribed Image Text:public void setType(String type) this method will set the type of the vehicle to either Sedan or SUV
or Truck.
public void setEntryTime(Date entryTime) this method will set the time at which the vehicle has
entered the parking.
2- Write a CarParking class to model a multistory car parking building. This class should contain a 3-
dimensional array of type Vehicle. The third dimension of the array simply represents the number of
floors of the building while the first and second dimensions are the number of rows and columns in every
floor. By default, there are 3 floors, 7 rows and 7 columns in a building. However, the user should be able
to specify the maximum number of floors, rows and columns at the time of creating a CarParking object.
In addition to the constructors, this class should provide the following methods:
O
public boolean isOccupied (int floor, int row, int col) this method returns true if the location
specified by the floor, row and column is already occupied by a vehicle.
public void add (Vehicle v) this method will add the vehicle at the next empty slot. Slots are field
row by row (from left to right) starting from the ground floor.
public Vehicle remove (int floor, int row, int col, double rate) this method to removes the
vehicle at the specified location and returns the parking fee. The fee is calculated by multiplying
the rate by the duration (in minutes). Drivers will receive 5% discount per floor other than the
ground floor. If the location is not occupied, the method returns zero.
public double getTotalExpectedRevenue(double[] rates, Date exitTime) this method
calculates the total expected revenue if all cars exit the building at the given exitTime. The rates
array will contain an element for every vehicle type (Sedan, SUV, Truck).
public int getVehicleCount(String type, int floor) this method finds and returns the total number
of cars for a given type in a given floor.
O public double getRevenue (String type, int floor, double rate) this method finds and returns
the total revenue of a given car type in a given floor for a given rate.
public boolean canOrganizeByType() this method should return true if it is possible to
accommodate all cars of the same type in separate floors.
D
O
O
O
O
O
3- Write a Java program that uses the CarParking class and the Vehicle class to simulate a car parking
building. The program should start by constructing a CarParking object. The user should then be presented
with a menu to select one of the following actions:
Add a vehicle: This option constructs a vehicle object and adds it to the parking. The user should
be required to enter the vehicle type.
Remove a vehicle: This option removes a vehicle from the building and display its the parking
fees. The user should be prompted to enter the location and the rate.
Display statistics: this option will show a summary including:
●
i. Total expected revenue
ii. Number of sedans, SUVs and trucks per floor
iii. Revenue of sedans, SUVs and trucks per floor
iv. Number of empty slots per floor
Check arrangement: as a result of this selection the program should display one of the following
messages :
i. Cars can be arranged based on their type
ii. Not possible to rearrange cars based on their type.
Exit the program: This option will terminate the program
3. Hints:
1- Use the java.util.Date class to represent the time. This class contain several methods that allow you to
find for example the time difference in minutes between any two times.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 3 images

Recommended textbooks for you
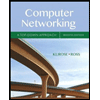
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
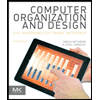
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
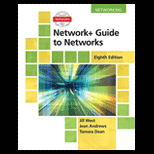
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
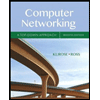
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
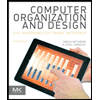
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
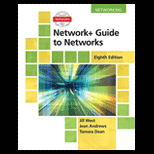
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
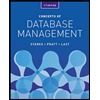
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
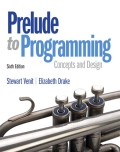
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
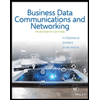
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY