put(self, content, evictionPolicy): It adds nodes at the beginning of the list and evicts items as necessary to free up space. If the content is larger than the maximum size, it doesn't evict anything. Otherwise, if there is currently not enough space for the content, evict items according to the eviction policy. If the content id exists in the list prior to the insertion, new content is not added to the list, but the existing content is moved to the beginning of the list. contains(self, cid): It finds a ContentItem from the list by id, moving the ContentItem to the front of the list if found. This is the special method for the in operator to allow the syntax cid in object. update(self, cid, content): It updates a ContentItem with a given id in the list. If a match is found, it is moved to the beginning of the list and the old ContentItem is entirely replaced with the new ContentItem. It is not assumed the size of the new content is the same as the content in the list, thus, must check that there is enough remaingSpace to perform the update. The update is not completed if the change results on exceeding the maxSize of the list, but the match is moved at the beginning of the list. lruEvict(self) / mruEvict(self): Removes the last (least recently used) or the first (most recently used) item of the list clear(self) Removes all items from the list. class CacheList: def __init__(self, size): self.head = None self.maxSize = size self.remainingSpace = size self.numItems = 0 def __str__(self): listString = "" current = self.head while current is not None: listString += "[" + str(current.value) + "]\n" current = current.next return 'REMAINING SPACE:{}\nITEMS:{}\nLIST:\n{}'.format(self.remainingSpace, self.numItems, listString) __repr__=__str__ def __len__(self): return self.numItems def put(self, content, evictionPolicy): # YOUR CODE STARTS HERE pass def __contains__(self, cid): # YOUR CODE STARTS HERE pass def update(self, cid, content): # YOUR CODE STARTS HERE pass def mruEvict(self): # YOUR CODE STARTS HERE pass def lruEvict(self): # YOUR CODE STARTS HERE pass def clear(self): # YOUR CODE STARTS HERE pass Expected output: >>> content1 = ContentItem(1000, 10, "Content-Type: 0", "0xA") >>> content2 = ContentItem(1004, 50, "Content-Type: 1", "110010") >>> content3 = ContentItem(1005, 180, "Content-Type: 2", "'CMPSC132'") >>> content4 = ContentItem(1006, 18, "another header", "111110") >>> content5 = ContentItem(1008, 2, "items", "11x1110") >>> lst=CacheList(200) >>> lst REMAINING SPACE:200 ITEMS:0 LIST: >>> lst.put(content1, 'mru') 'INSERTED: CONTENT ID: 1000 SIZE: 10 HEADER: Content-Type: 0 CONTENT: 0xA' >>> lst.put(content2, 'lru') 'INSERTED: CONTENT ID: 1004 SIZE: 50 HEADER: Content-Type: 1 CONTENT: 110010' >>> lst.put(content4, 'mru') 'INSERTED: CONTENT ID: 1006 SIZE: 18 HEADER: another header CONTENT: 111110' >>> lst.put(content5, 'mru') 'INSERTED: CONTENT ID: 1008 SIZE: 2 HEADER: items CONTENT: 11x1110' >>> lst.put(content3, 'lru') "INSERTED: CONTENT ID: 1005 SIZE: 180 HEADER: Content-Type: 2 CONTENT: 'CMPSC132'" >>> lst.put(content1, 'mru') 'INSERTED: CONTENT ID: 1000 SIZE: 10 HEADER: Content-Type: 0 CONTENT: 0xA' >>> 1006 in lst True >>> contentExtra = ContentItem(1034, 2, "items", "other content") >>> lst.update(1008, contentExtra) 'UPDATED: CONTENT ID: 1034 SIZE: 2 HEADER: items CONTENT: other content' >>> lst REMAINING SPACE:170 ITEMS:3 LIST: [CONTENT ID: 1034 SIZE: 2 HEADER: items CONTENT: other content] [CONTENT ID: 1006 SIZE: 18 HEADER: another header CONTENT: 111110] [CONTENT ID: 1000 SIZE: 10 HEADER: Content-Type: 0 CONTENT: 0xA] >>> lst.clear() 'Cleared cache!' >>> lst REMAINING SPACE:200 ITEMS:0 LIST:
Please do NOT ADD an extra method or def() such as find() or others, I have to get an expected output by ONLY modifying the bold parts.
Please please do not add an extra method def() PLEASE!!
put(self, content, evictionPolicy): It adds nodes at the beginning of the list and evicts items as necessary to free up space. If the content is larger than the maximum size, it doesn't evict anything. Otherwise, if there is currently not enough space for the content, evict items according to the eviction policy. If the content id exists in the list prior to the insertion, new content is not added to the list, but the existing content is moved to the beginning of the list.
contains(self, cid): It finds a ContentItem from the list by id, moving the ContentItem to the front of the list if found. This is the special method for the in operator to allow the syntax cid in object.
update(self, cid, content): It updates a ContentItem with a given id in the list. If a match is found, it is moved to the beginning of the list and the old ContentItem is entirely replaced with the new ContentItem. It is not assumed the size of the new content is the same as the content in the list, thus, must check that there is enough remaingSpace to perform the update. The update is not completed if the change results on exceeding the maxSize of the list, but the match is moved at the beginning of the list.
lruEvict(self) / mruEvict(self): Removes the last (least recently used) or the first (most recently used) item of the list
clear(self)
Removes all items from the list.
class CacheList:
def __init__(self, size):
self.head = None
self.maxSize = size
self.remainingSpace = size
self.numItems = 0
def __str__(self):
listString = ""
current = self.head
while current is not None:
listString += "[" + str(current.value) + "]\n"
current = current.next
return 'REMAINING SPACE:{}\nITEMS:{}\nLIST:\n{}'.format(self.remainingSpace, self.numItems, listString)
__repr__=__str__
def __len__(self):
return self.numItems
def put(self, content, evictionPolicy):
# YOUR CODE STARTS HERE
pass
def __contains__(self, cid):
# YOUR CODE STARTS HERE
pass
def update(self, cid, content):
# YOUR CODE STARTS HERE
pass
def mruEvict(self):
# YOUR CODE STARTS HERE
pass
def lruEvict(self):
# YOUR CODE STARTS HERE
pass
def clear(self):
# YOUR CODE STARTS HERE
pass
Expected output:
>>> content1 = ContentItem(1000, 10, "Content-Type: 0", "0xA")
>>> content2 = ContentItem(1004, 50, "Content-Type: 1", "110010")
>>> content3 = ContentItem(1005, 180, "Content-Type: 2", "<html><p>'CMPSC132'</p></html>")
>>> content4 = ContentItem(1006, 18, "another header", "111110")
>>> content5 = ContentItem(1008, 2, "items", "11x1110")
>>> lst=CacheList(200)
>>> lst
REMAINING SPACE:200
ITEMS:0
LIST:
<BLANKLINE>
>>> lst.put(content1, 'mru')
'INSERTED: CONTENT ID: 1000 SIZE: 10 HEADER: Content-Type: 0 CONTENT: 0xA'
>>> lst.put(content2, 'lru')
'INSERTED: CONTENT ID: 1004 SIZE: 50 HEADER: Content-Type: 1 CONTENT: 110010'
>>> lst.put(content4, 'mru')
'INSERTED: CONTENT ID: 1006 SIZE: 18 HEADER: another header CONTENT: 111110'
>>> lst.put(content5, 'mru')
'INSERTED: CONTENT ID: 1008 SIZE: 2 HEADER: items CONTENT: 11x1110'
>>> lst.put(content3, 'lru')
"INSERTED: CONTENT ID: 1005 SIZE: 180 HEADER: Content-Type: 2 CONTENT: <html><p>'CMPSC132'</p></html>"
>>> lst.put(content1, 'mru')
'INSERTED: CONTENT ID: 1000 SIZE: 10 HEADER: Content-Type: 0 CONTENT: 0xA'
>>> 1006 in lst
True
>>> contentExtra = ContentItem(1034, 2, "items", "other content")
>>> lst.update(1008, contentExtra)
'UPDATED: CONTENT ID: 1034 SIZE: 2 HEADER: items CONTENT: other content'
>>> lst
REMAINING SPACE:170
ITEMS:3
LIST:
[CONTENT ID: 1034 SIZE: 2 HEADER: items CONTENT: other content]
[CONTENT ID: 1006 SIZE: 18 HEADER: another header CONTENT: 111110]
[CONTENT ID: 1000 SIZE: 10 HEADER: Content-Type: 0 CONTENT: 0xA]
<BLANKLINE>
>>> lst.clear()
'Cleared cache!'
>>> lst
REMAINING SPACE:200
ITEMS:0
LIST:
<BLANKLINE>

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

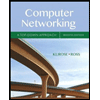
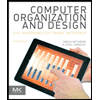
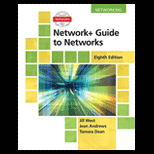
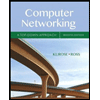
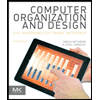
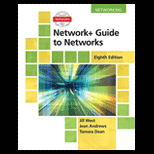
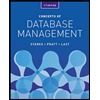
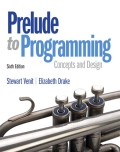
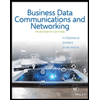