Lab 20 Removing the first element in a list Start this lab with the code listed below. The LinkedList class defines the rudiments of the code needed to build a linked list of Nodes. Complete the code for the removeFirst method, which should remove and return the first element in the linked list. Throw a NoSuchElementException if the method is invoked on an empty list. Use the LinkedListRunner class’s main method to test your code. import java.util.NoSuchElementException; public class LinkedList { private Node first; public LinkedList() { first = null; } public Object getFirst() { if (first == null) { throw new NoSuchElementException(); } return first.data; } public void addFirst(Object element) { Node aNode = new Node(); aNode.data = element; aNode.next = first; first = aNode; } public Object removeFirst() { // put your code here } public String toString() { String temp = ""; Node current = first; while (current != null) { temp = temp + current.data.toString() + '\n'; current = current.next; } return temp; } class Node { public Object data; public Node next; } } ----------------------------------- public class LinkedListRunner { public static void main(String[] args) { LinkedList myList = new LinkedList(); myList.addFirst("aaa"); myList.addFirst("bbb"); myList.addFirst("ccc"); myList.addFirst("ddd"); System.out.println(myList); System.out.println("Removed element: " + myList.removeFirst()); System.out.println("Removed element: " + myList.removeFirst()); System.out.println(myList); } }
Lab 20 Removing the first element in a list
Start this lab with the code listed below. The LinkedList class defines the rudiments of the code needed to build a linked list of Nodes. Complete the code for the removeFirst method, which should remove and return the first element in the linked list. Throw a NoSuchElementException if the method is invoked on an empty list. Use the LinkedListRunner class’s main method to test your code.
import java.util.NoSuchElementException;
public class LinkedList
{
private Node first;
public LinkedList() { first = null; }
public Object getFirst()
{
if (first == null) { throw new NoSuchElementException(); }
return first.data;
}
public void addFirst(Object element)
{
Node aNode = new Node();
aNode.data = element;
aNode.next = first;
first = aNode;
}
public Object removeFirst()
{
// put your code here
}
public String toString()
{
String temp = "";
Node current = first;
while (current != null)
{
temp = temp + current.data.toString() + '\n';
current = current.next;
}
return temp;
}
class Node
{
public Object data;
public Node next;
}
}
-----------------------------------
public class LinkedListRunner
{
public static void main(String[] args)
{
LinkedList myList = new LinkedList();
myList.addFirst("aaa");
myList.addFirst("bbb");
myList.addFirst("ccc");
myList.addFirst("ddd");
System.out.println(myList);
System.out.println("Removed element: " + myList.removeFirst());
System.out.println("Removed element: " + myList.removeFirst());
System.out.println(myList);
}
}

Step by step
Solved in 3 steps with 3 images

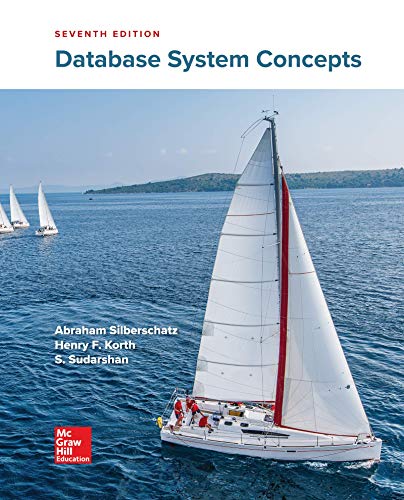
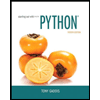
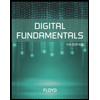
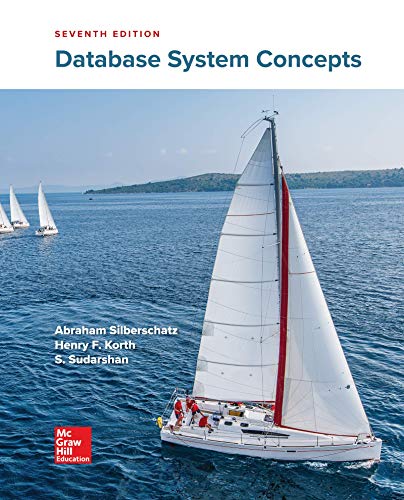
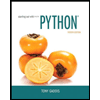
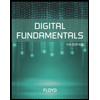
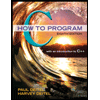
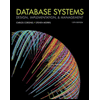
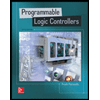