Python code: ############################## class Node: def __init__(self,value): self.left = None self.right = None self.val = value ############################### class BinarySearchTree: def __init__(self): self.root = None def print_tree(node): if node == None: return print_tree(node.left) print_tree(node.right) print(node.val) ################################################# # Task 1: get_nodes_in_range function ################################################# def get_nodes_in_range(node,min,max): # # your code goes here # pass # fix this if __name__ == '__main__': BST = BinarySearchTree() BST.root = Node(10) BST.root.left = Node(5) BST.root.right = Node(15) BST.root.left.left = Node(2) BST.root.left.right = Node(8) BST.root.right.left = Node(12) BST.root.right.right = Node(20) BST.root.right.right.right = Node(25) print(get_nodes_in_range(BST.root, 6, 20))
Python code:
##############################
class Node:
def __init__(self,value):
self.left = None
self.right = None
self.val = value
###############################
class BinarySearchTree:
def __init__(self):
self.root = None
def print_tree(node):
if node == None:
return
print_tree(node.left)
print_tree(node.right)
print(node.val)
#################################################
# Task 1: get_nodes_in_range function
#################################################
def get_nodes_in_range(node,min,max):
#
# your code goes here
#
pass # fix this
if __name__ == '__main__':
BST = BinarySearchTree()
BST.root = Node(10)
BST.root.left = Node(5)
BST.root.right = Node(15)
BST.root.left.left = Node(2)
BST.root.left.right = Node(8)
BST.root.right.left = Node(12)
BST.root.right.right = Node(20)
BST.root.right.right.right = Node(25)
print(get_nodes_in_range(BST.root, 6, 20))
![In this lab you are provided with a pre-populated Binary Search Tree and asked to implement the function get_nodes_in_range (node,
min, max) which returns a list (Python built-in list) of all the values in nodes in the tree which are within the range (inclusive of the min and
max).
As an example, for the following BST:
BST = BinarySearchTree ()
BST.root = Node (10)
BST.root.left = Node (5)
BST.root.right = Node (15)
BST.root.left.left = Node (2)
BST.root.left.right = Node (8)
the expected output for get_nodes_in_range (BST.root, 6, 20) is a list containing the following values:
[8, 15, 10]
NOTE: the order of the nodes in the list doesn't matter. The tests will check that the list returned by your function contains the same values](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F45d19586-ebc3-4f1a-8702-47e93c6193b1%2Fe9a8217d-36ef-4a7c-9855-d44be7067121%2Ftdb9enh_processed.png&w=3840&q=75)

Step by step
Solved in 3 steps with 1 images

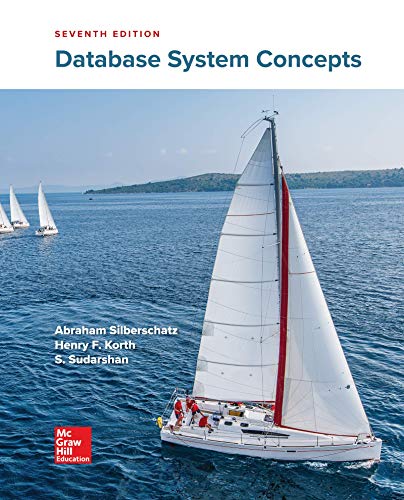
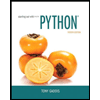
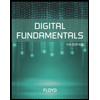
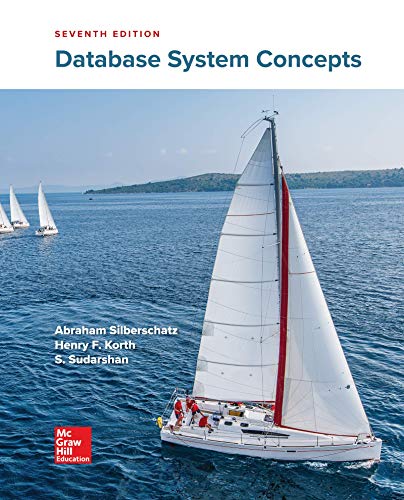
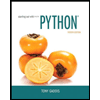
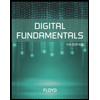
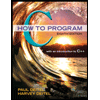
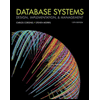
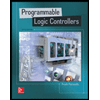