Python Code please
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Python Code please
Point of Sale
Write a program that will manage the point of sale in a store.
-
Build the ItemToPurchase class with the following:
- Attributes
- item_name (string)
- item_price (int)
- item_quantity (int)
- Default constructor
- Initializes item's name = "none", item's price = 0, item's quantity = 0
- Method
- print_item_cost()
Bottled Water 10 @ $1 = $10 - Attributes
-
Extend the ItemToPurchase class to contain a new attribute.
- item_description (string) - Set to "none" in default constructor
Implement the following method for the ItemToPurchase class.
- print_item_description() - Prints item_description attribute for an ItemToPurchase object. Has an ItemToPurchase parameter.
Bottled Water: Deer Park, 12 oz. - Build the ShoppingCart class with the following data attributes and related methods. Note: Some can be method stubs (empty methods) initially, to be completed in later steps.
- Parameterized constructor which takes the customer name and date as parameters
- Attributes
- customer_name (string) - Initialized in default constructor to "none"
- current_date (string) - Initialized in default constructor to "January 1, 2016"
- cart_items (list)
- Methods
- add_item()
- Adds an item to cart_items list. Has a parameter of type ItemToPurchase. Does not return anything.
- remove_item()
- Removes item from cart_items list. Has a string (an item's name) parameter. Does not return anything.
- If item name cannot be found, output this message: Item not found in cart. Nothing removed.
- modify_item()
- Modifies an item's quantity. Has a parameter of type ItemToPurchase. Does not return anything.
- If item can be found (by name) in cart, modify item in cart.
- If item cannot be found (by name) in cart, output this message: Item not found in cart. Nothing modified.
- get_num_items_in_cart()
- Returns quantity of all items in cart. Has no parameters.
- get_cost_of_cart()
- Determines and returns the total cost of items in cart. Has no parameters.
- print_total()
- Outputs total of objects in cart.
- If cart is empty, output this message: SHOPPING CART IS EMPTY
- print_descriptions()
- Outputs each item's description.
- add_item()
- In the main section of the code, prompt the user for a customer's name and today's date. Output the name and date. Create an object of type ShoppingCart.
Example:Enter customer's name: John Doe Enter today's date: February 1, 2016 Customer name: John Doe Today's date: February 1, 2016 - Implement the print_menu() function to print the following menu of options to manipulate the shopping cart.
Example:MENU a - Add item to cart r - Remove item from cart c - Change item quantity i - Output items' descriptions o - Output shopping cart q - Quit - Implement the execute_menu() function that takes 2 parameters: a character representing the user's choice and a shopping cart. execute_menu() performs the menu options described below, according to the user's choice.
- In the main section of the code, call print_menu() and prompt for the user's choice of menu options. Each option is represented by a single character. If an invalid character is entered, continue to prompt for a valid choice. When a valid option is entered, execute the option by calling execute_menu(). Then, print the menu and prompt for a new option. Continue until the user enters 'q'.
Hint: Implement Quit before implementing other options.
Example:a - Add item to cart r - Remove item from cart c - Change item quantity i - Output items' descriptions o - Output shopping cart q - Quit Choose an option: - Implement Output shopping cart menu option in execute_menu().
Example:OUTPUT SHOPPING CART John Doe's Shopping Cart - February 1, 2016 Number of Items: 8 Nike Romaleos 2 @ $189 = $378 Chocolate Chips 5 @ $3 = $15 Powerbeats 2 Headphones 1 @ $128 = $128 Total: $521 - Implement Output item's description menu option in execute_menu().
Example:OUTPUT ITEMS' DESCRIPTIONS John Doe's Shopping Cart - February 1, 2016 Item Descriptions Nike Romaleos: Volt color, Weightlifting shoes Chocolate Chips: Semi-sweet Powerbeats 2 Headphones: Bluetooth headphones - Implement Add item to cart menu option in execute_menu().
Example:ADD ITEM TO CART Enter the item name: Nike Romaleos Enter the item description: Volt color, Weightlifting shoes Enter the item price: 189 Enter the item quantity: 2 - Implement remove item menu option in execute_menu().
Example:REMOVE ITEM FROM CART Enter name of item to remove: Chocolate Chips - Implement Change item quantity menu option in execute_menu().
Example:CHANGE ITEM QUANTITY Enter the item name: Nike Romaleos Enter the new quantity: 3
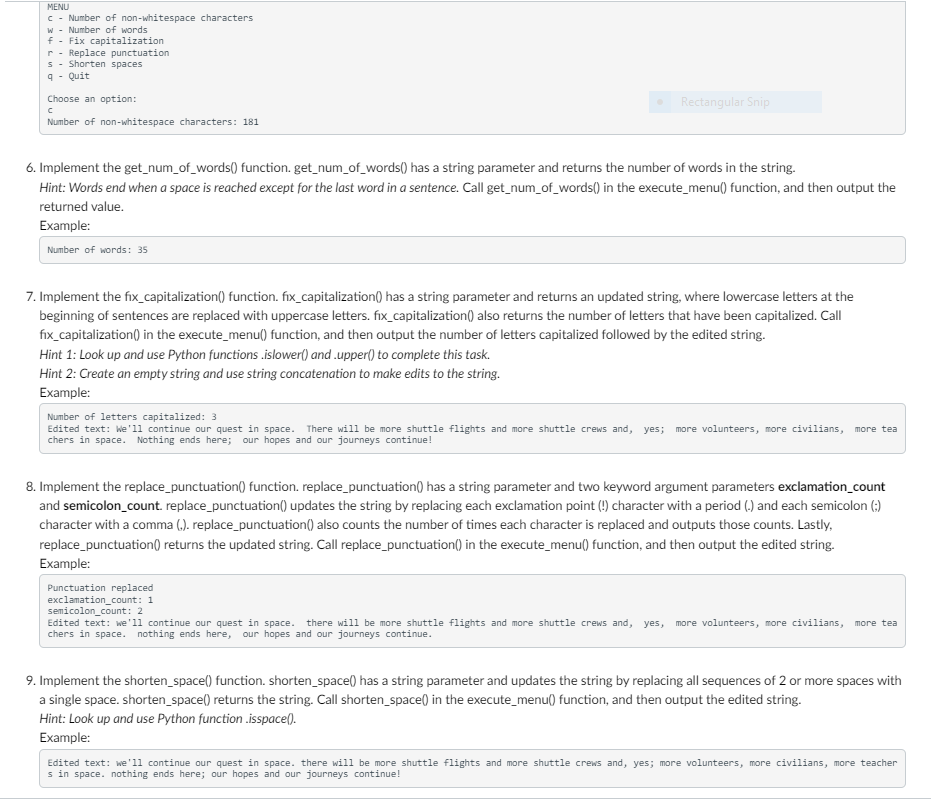
Transcribed Image Text:MENU
c - Number of non-whitespace characters
w Number of words
f
Fix capitalization
r - Replace punctuation
s
Shorten spaces
q - Quit
Choose an option:
C
Number of non-whitespace characters: 181
6. Implement the get_num_of_words() function. get_num_of_words() has a string parameter and returns the number of words in the string.
Hint: Words end when a space is reached except for the last word in a sentence. Call get_num_of_words() in the execute_menu() function, and then output the
returned value.
Example:
Number of words: 35
Rectangular Snip
7. Implement the fix_capitalization() function. fix_capitalization() has a string parameter and returns an updated string, where lowercase letters at the
beginning of sentences are replaced with uppercase letters. fix_capitalization() also returns the number of letters that have been capitalized. Call
fix_capitalization() in the execute_menu() function, and then output the number of letters capitalized followed by the edited string.
Hint 1: Look up and use Python functions.islower() and .upper() to complete this task.
Hint 2: Create an empty string and use string concatenation to make edits to the string.
Example:
Number of letters capitalized: 3
Edited text: We'll continue our quest in space. There will be more shuttle flights and more shuttle crews and, yes; more volunteers, more civilians, more tea
chers in space. Nothing ends here; our hopes and our journeys continue!
8. Implement the replace_punctuation() function. replace_punctuation() has a string parameter and two keyword argument parameters exclamation_count
and semicolon_count. replace_punctuation() updates the string by replacing each exclamation point (!) character with a period (.) and each semicolon (;)
character with a comma (.). replace_punctuation () also counts the number of times each character is replaced and outputs those counts. Lastly,
replace_punctuation() returns the updated string. Call replace_punctuation() in the execute_menu() function, and then output the edited string.
Example:
Punctuation replaced
exclamation_count: 1
semicolon_count: 2
Edited text: we'll continue our quest in space. there will be more shuttle flights and more shuttle crews and, yes, more volunteers, more civilians, more tea
chers in space. nothing ends here, our hopes and our journeys continue.
9. Implement the shorten_space() function. shorten_space() has a string parameter and updates the string by replacing all sequences of 2 or more spaces with
a single space. shorten_space() returns the string. Call shorten_space() in the execute_menu() function, and then output the edited string.
Hint: Look up and use Python function.isspace().
Example:
Edited text: we'll continue our quest in space. there will be more shuttle flights and more shuttle crews and, yes; more volunteers, more civilians, more teacher
s in space. nothing ends here; our hopes and our journeys continue!
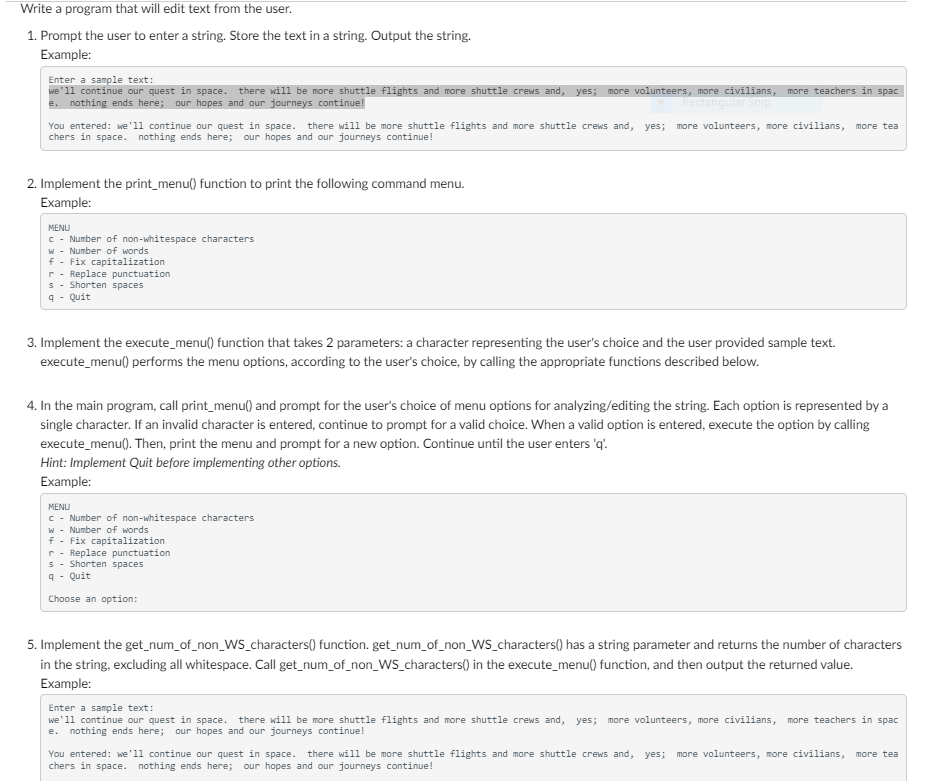
Transcribed Image Text:Write a program that will edit text from the user.
1. Prompt the user to enter a string. Store the text in a string. Output the string.
Example:
Enter a sample text:
we'll continue our quest in space. there will be more shuttle flights and more shuttle crews and, yes; more volunteers, more civilians,
e. nothing ends here; our hopes and our journeys continue!
Rectangular Snip
You entered: we'll continue our quest in space. there will be more shuttle flights and more shuttle crews and, yes; more volunteers, more civilians, more tea
chers in space. nothing ends here; our hopes and our journeys continue!
2. Implement the print_menu() function to print the following command menu.
Example:
MENU
c - Number of non-whitespace characters
w Number of words
f Fix capitalization
r-Replace punctuation
s Shorten spaces
q- Quit
3. Implement the execute_menu() function that takes 2 parameters: a character representing the user's choice and the user provided sample text.
execute_menu() performs the menu options, according to the user's choice, by calling the appropriate functions described below.
more teachers in spac
4. In the main program, call print_menu() and prompt for the user's choice of menu options for analyzing/editing the string. Each option is represented by a
single character. If an invalid character is entered, continue to prompt for a valid choice. When a valid option is entered, execute the option by calling
execute_menu(). Then, print the menu and prompt for a new option. Continue until the user enters 'q'.
Hint: Implement Quit before implementing other options.
Example:
MENU
c - Number of non-whitespace characters
w Number of words
f
Fix capitalization
r Replace punctuation
s
Shorten spaces
q - Quit
Choose an option:
5. Implement the get_num_of_non_WS_characters() function. get_num_of_non_WS_characters() has a string parameter and returns the number of characters
in the string, excluding all whitespace. Call get_num_of_non_WS_characters() in the execute_menu() function, and then output the returned value.
Example:
Enter a sample text:
we'll continue our quest in space. there will be more shuttle flights and more shuttle crews and, yes; more volunteers, more civilians, more teachers in spac
e. nothing ends here; our hopes and our journeys continue!
You entered: we'll continue our quest in space. there will be more shuttle flights and more shuttle crews and, yes; more volunteers, more civilians, more tea
chers in space. nothing ends here; our hopes and our journeys continue!
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
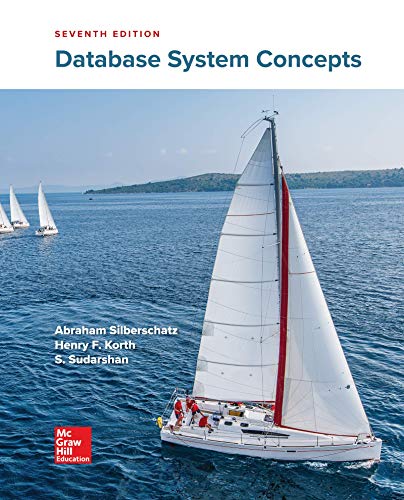
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
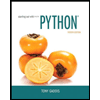
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
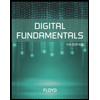
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
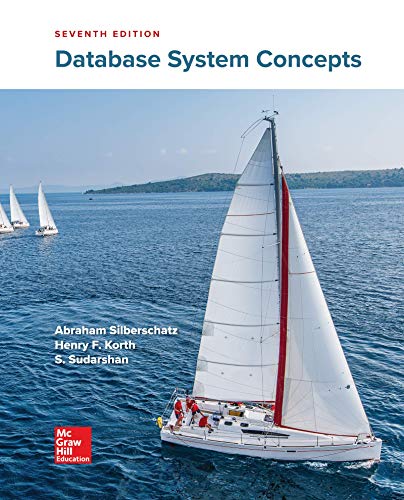
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
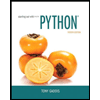
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
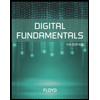
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
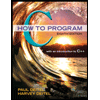
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
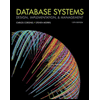
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
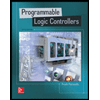
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education