U is upward, D is downward, L is left and R is right : Using the methods pickDir() and exractInt() as shown below, to write a simple automated game: how long does it take a robot to travel from a left line of a field to the right line? Assume field is 15 units apart (meters, kilometers, it doesn’t matter), and the robot starts on the left line. pickDir(): // return string with random direction and random distance (within maxDist) public static String pickDir (int maxDist) { Random r = new Random(); // random number generator // return direction + distance return ( "" + "LRUD".charAt( r.nextInt(4) ) + (r.nextInt(maxDist) + 1) ); } extractInt(): // returns integer from string, from pos p1 to p2: str = "D1" – extractInt(str,1,1); public static int extractInt (String str, int p1, int p2) { return ( Integer.parseInt( str.substring(p1, p2+1) ) ); } Write the main() method that keeps looping until the robot’s random left and right movements have the robot move past the right line, using the following rules: • starting travel distance (Trav) is zero (0)—the robot is starting on the left line • maximum distance, when picking a random direction, is 9 units • robot moves to the left (L) are negative integer distances (-) to the travel distance, and robot moves to the right (R) are positive integer distances (+) to the travel distance • robot moves up (U) and down (D) do not affect the left-right travel distance—but they are still displayed • keep looping while the robot’s travel distance <= 15 units (i.e., stop when travel distance > 15 units) - after the loop, display “robot has crossed the right line!” • in each loop iteration, the robot picks a new random direction (Rand), by calling pickDir() - hint: .charAt(), and method extractInt() (see previous question) for direction & distance • since the robot is travelling randomly, there is a possibility it may never cross the right line (or take a very, very, very long to cross), and it will run out of power, - add another condition to the loop, stopping if the number of iterations exceeds MAXLOOPS (set MAXLOOPS to 20, 50, or 100, depending on how much power the robot’s battery contains) - if the exceeding MAXLOOPS, display “battery power depleted, robot has stopped.” - In each iteration, display the current travel distance and current random direction. example run: Robot Travelling Trav: 0 Rand: L1 Trav: -1 Rand: R3 Trav: 2 Rand: L1 Trav: 1 Rand: D4 Trav: 1 Rand: R8 Trav: 9 Rand: U3 Trav: 9 Rand: L3 Trav: 6 Rand: U1 Trav: 6 Rand: R6 Trav: 12 Rand: D1 Trav: 12 Rand: L2 Trav: 10 Rand: R8 Trav: 18 Done—robot has crossed the right line!
U is upward, D is downward, L is left and R is right : Using the methods pickDir() and exractInt() as shown below, to write a simple automated game: how long does it take a robot to travel from a left line of a field to the right line?
Assume field is 15 units apart (meters, kilometers, it doesn’t matter), and the robot starts on the left line.
pickDir():
// return string with random direction and random distance (within maxDist)
public static String pickDir (int maxDist)
{
Random r = new Random(); // random number generator
// return direction + distance
return ( "" + "LRUD".charAt( r.nextInt(4) ) + (r.nextInt(maxDist) + 1) );
}
extractInt():
// returns integer from string, from pos p1 to p2: str = "D1" – extractInt(str,1,1);
public static int extractInt (String str, int p1, int p2)
{
return ( Integer.parseInt( str.substring(p1, p2+1) ) );
}
Write the main() method that keeps looping until the robot’s random left and right movements have the robot move past the right line, using the following rules:
• starting travel distance (Trav) is zero (0)—the robot is starting on the left line
• maximum distance, when picking a random direction, is 9 units
• robot moves to the left (L) are negative integer distances (-) to the travel distance, and robot moves to the right (R) are positive integer distances (+) to the travel distance
• robot moves up (U) and down (D) do not affect the left-right travel distance—but they are still displayed
• keep looping while the robot’s travel distance <= 15 units (i.e., stop when travel distance > 15 units)
- after the loop, display “robot has crossed the right line!”
• in each loop iteration, the robot picks a new random direction (Rand), by calling pickDir()
- hint: .charAt(), and method extractInt() (see previous question) for direction & distance
• since the robot is travelling randomly, there is a possibility it may never cross the right line (or take a very, very, very long to cross), and it will run out of power,
- add another condition to the loop, stopping if the number of iterations exceeds MAXLOOPS (set MAXLOOPS to 20, 50, or 100, depending on how much power the robot’s battery contains)
- if the exceeding MAXLOOPS, display “battery power depleted, robot has stopped.”
- In each iteration, display the current travel distance and current random direction.
example run:
Robot Travelling
Trav: 0
Rand: L1
Trav: -1
Rand: R3
Trav: 2
Rand: L1
Trav: 1
Rand: D4
Trav: 1
Rand: R8
Trav: 9
Rand: U3
Trav: 9
Rand: L3
Trav: 6
Rand: U1
Trav: 6
Rand: R6
Trav: 12
Rand: D1
Trav: 12
Rand: L2
Trav: 10
Rand: R8
Trav: 18
Done—robot has crossed the right line!

Step by step
Solved in 3 steps with 3 images

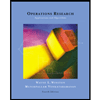
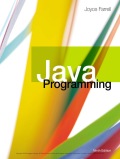
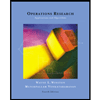
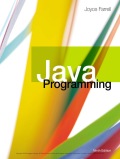