Python Please Recap of two-dimensional arrays and their numpy implementation ** uploaded image ^ 1) In this problem, implement a TwoDArray class that is meant to mimic (some of) the functionality of a two-dimensional numpy array. DO NOT use numpy at any point. 1A) Give the class an __init__ method Make sure that the variable array is a valid input. This means you should a) Make sure that array is a list of lists. and b) Make sure that each of the inner lists has the same length. You do not need to check for anything else. If these conditions are not met you should raise the appropriate error(s). It is up for you to decide if you should raise a TypeError, KeyError, IndexError, ValueError, ZeroDivisionError, etc. When raising the error, you should also give an informative error message. With actual numpy arrays, all numbers are required to be the same datatype. You don't have to worry about that here. You can also assume that all numbers are ints or floats so you only have to worry about checking that the variable array is a list of lists.
Python Please
Recap of two-dimensional arrays and their numpy implementation
** uploaded image ^
1) In this problem, implement a TwoDArray class that is meant to mimic (some of) the functionality of a two-dimensional numpy array. DO NOT use numpy at any point.
1A) Give the class an __init__ method
Make sure that the variable array is a valid input. This means you should
a) Make sure that array is a list of lists. and b) Make sure that each of the inner lists has the same length. You do not need to check for anything else.
If these conditions are not met you should raise the appropriate error(s). It is up for you to decide if you should raise a TypeError, KeyError, IndexError, ValueError, ZeroDivisionError, etc. When raising the error, you should also give an informative error message.
With actual numpy arrays, all numbers are required to be the same datatype. You don't have to worry about that here. You can also assume that all numbers are ints or floats so you only have to worry about checking that the variable array is a list of lists.
1A)
def __init__(self, array):
self.array = array
*** What would be the rest of the code?
![2 import numpy as np
3
4 my_2d_array
np.array([[1,2],[3,4],[5,6]])
5 print("This is my_2d_array:")
6 print(my_2d_array)
7 print("This array has shape
str(my_2d_array.shape[0]) +
+ str(my_2d_array.shape) +
as there are
%3D
rows and"
+ str(my_2d_array.shape[1]) +
columns.")
+ str(1) +
8 print("The entry of the array with row index "
" has value "
and column index
+ str(1) +
+ str(my_2d_array[1,1]))](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fa7c87654-3157-4119-9345-56717eaa1677%2Fce4a0baa-a936-4018-aa3c-d7975c37fc8c%2Fgektidf_processed.png&w=3840&q=75)

Step by step
Solved in 3 steps with 1 images

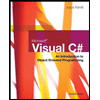
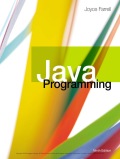
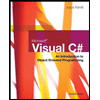
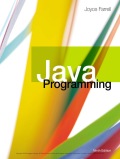