Parameters array - Any JavaScript Array size - A positive integer Number indicating the desired "chunk" size. Return Value A new Array of Arrays. Each sub-Array has a length equal to size. Remaining elements are gathered into a final sub-Array. Do not mutate the input array Do not use Array.prototype.slice in your implementation. Do not use Array.prototype.shift in your implementation. Do not use Array.prototype.pop in your implementation. Do not use Array.prototype.splice in your implementation. Please fix this JavaScript function so that the when calling the function with the parameters chunk([false, true, false, true], 3); the output is [[false, true, false], [true]] (subarrays) and when the parameters are chunk([undefined, null, 0, false, NaN, ""], 3); the output is [[undefined, null, 0], [false, NaN, ""]] thank you very much! function chunk(ar, sz) { var res = []; var ind = 0; // itterating through the new length of resulting array for (var i = 0; i < ar.length / sz; i++) { var t = []; // iterating through the given size for (var j = 0; j < sz; j++) { // adding elements to new 1D array t[] t.push(ar[ind]); ind++; } // push this new array into res[] res.push(t); } // return the answer return res; }
Parameters
- array - Any JavaScript Array
- size - A positive integer Number indicating the desired "chunk" size.
Return Value
A new Array of Arrays. Each sub-Array has a length equal to size. Remaining elements are gathered into a final sub-Array.
Do not mutate the input array
Do not use Array.prototype.slice in your implementation.
Do not use Array.prototype.shift in your implementation.
Do not use Array.prototype.pop in your implementation.
Do not use Array.prototype.splice in your implementation.
Please fix this JavaScript function so that the when calling the function with the parameters chunk([false, true, false, true], 3); the output is [[false, true, false], [true]] (subarrays)
and when the parameters are chunk([undefined, null, 0, false, NaN, ""], 3); the output is [[undefined, null, 0], [false, NaN, ""]]
thank you very much!
function chunk(ar, sz) {
var res = [];
var ind = 0;
// itterating through the new length of resulting array
for (var i = 0; i < ar.length / sz; i++) {
var t = [];
// iterating through the given size
for (var j = 0; j < sz; j++) {
// adding elements to new 1D array t[]
t.push(ar[ind]);
ind++;
}
// push this new array into res[]
res.push(t);
}
// return the answer
return res;
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

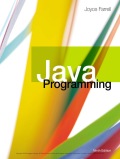
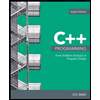
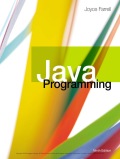
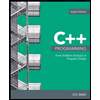