Q8: Matrix Operations def create_matrix(lst): """Create matrix will take matrix represented as a list of list. And output a function which will index the input matrix Args: lst (List of List): A list of list representation of matrix. There will be more than one element of list type in lst. Returns: [function]: An indexing function. >>> matrix_index1 = create_matrix([[1, 2], [2, 3]]) # a 2 x 2 matrix. >>> matrix_index2 = create_matrix([[1, 2, 3], [2, 3, 1]]) # a 2 x 3 matrix. >>> matrix_index3 = create_matrix([[1, 2, 3], []]) # an invalid matrix """ ### Modify your code here ### Modify your code here def matrix_index(i=None, j=None): """Indexing function which will retrive (i, j) entry of the input matrix representation Args: i (int, optional): row index. Defaults to None. (0-index) j (int, optional): column index. Defaults to None. (0-index) Return: List of List: a list of list representation of the indexed. Return None if matrix or index is invalid >>> matrix_index1() [[1, 2], [2, 3]] >>> matrix_index1(i=1) # row 1 (2nd row since 0 indexed) [[2, 3]] >>> matrix_index1(j=1) # col 1 (2nd row since 0 indexed) [[2], [3]] >>> matrix_index1(i=1, j=1) [[3]] >>> print(matrix_index1(i=1, j=10)) None # invalid index >>> print(matrix_index3()) None # invalid matrix """ ### Modify your code here return matrix_index ### Modify your code here
Types of Linked List
A sequence of data elements connected through links is called a linked list (LL). The elements of a linked list are nodes containing data and a reference to the next node in the list. In a linked list, the elements are stored in a non-contiguous manner and the linear order in maintained by means of a pointer associated with each node in the list which is used to point to the subsequent node in the list.
Linked List
When a set of items is organized sequentially, it is termed as list. Linked list is a list whose order is given by links from one item to the next. It contains a link to the structure containing the next item so we can say that it is a completely different way to represent a list. In linked list, each structure of the list is known as node and it consists of two fields (one for containing the item and other one is for containing the next item address).
![### Q8: Matrix Operations
def create_matrix(1st):
"""Create matrix will take matrix represented as a list of list.
And output a function which will index the input matrix
Args:
1st (List of List): A list of list representation of matrix.
There will be more than one element of list type in 1st.
Returns:
[function]: An indexing function.
>> matrix_index1 = create_matrix([[1, 2], [2, 3]]) # a 2 x 2 matrix.
>>> matrix_index2
>>> matrix_index3 = create_matrix([[1, 2, 3], []]) # an invalid matrix
create_matrix([[1, 2, 3], [2, 3, 1]]) # a 2 x 3 matrix.
### Modify your code here
### Modify your code here
def matrix_index(i=None, j=None):
"""Indexing function which will retrive (i, j) entry of the input
matrix representation
Args:
i (int, optional): row index. Defaults to None. (0-index)
j (int, optional): column index. Defaults to None. (0-index)
Return:
List of List: a list of list representation of the indexed.
Return None if matrix or index is invalid
>>> matrix_index1()
[[1, 2], [2, 3]]
>>> matrix_index1(i=1) # row 1 (2nd row since 0 indexed)
[[2, 3]]
>>> matrix_index1(j=1) # col 1 (2nd row since e indexed)
[[2], [3]]
>>> matrix_index1(i=1, j=1)
[[3]]
>>> print(matrix_index1(i=1, j=1®))
None # invalid index
>>> print(matrix_index3())
None # invalid matrix
### Modify your code here
return matrix_index
### Modify your code here](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F11f6515a-e2b7-4581-b9b4-488784357aba%2F4058911d-ceab-47b9-99ea-c7548b82e39c%2Fp5aaxs_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

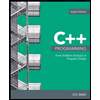
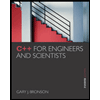
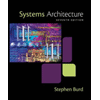
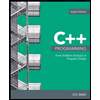
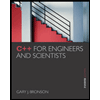
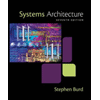