Question 1 Background
Question 1
Background
You are required to write program that will read a file, encrypt the content of the file, and then
create a new file that contains the encrypted content.
Your program will make use of the following classes:
Page – This class will be used to represent the content of the file that was read.
PageManager – This class will be used to read page content from and to a file.
APM11A1Encryptor – This class will be used to encrypt the content of a file using the Caesar-Cipher
method.
APM11A1 - Advanced
Programming
AdvancedAPM11A1Encryptor – This class will be used to encrypt the content of a file using the
Exclusive-OR method.
Caesar Cipher
Caesar was a Roman military and political leader during 100BC to 44BC. In order to make the
communication channels secure during war, he invented an encryption technique called the Caesar
Cipher. The cipher requires a sentence and a key to function properly. Each character in the sentence
was then shifted according to the value of the key. Computer programmers adapted the cipher so that
it can be used in programs. Each alphabet letter is assigned a value. This value is determined by the
position of the letter in the ASCII table. E.g. A=65, B=66, C=67, D=68 …. X=88, Y=89, Z=90. The key is
determined by the person who wants to encrypt the sentence. If you select a key=1, and you have a
sentence=”TPG11BT is fun”, it will encrypt to: UQH22CU!jt!gvo.
Exclusive-OR Encryption
The exclusive-OR method requires a key and a sentence to function properly. The ACII value of each
character in the sentence is exclusive-ORed with the key. E.g. If you select a key=1, and you have a
sentence=”TPG11BT is fun”, it will encrypt to: UQF00CU!hr!gto.
Below is an example of how to exclusive-OR the character in x with 1:
char x = ‘T’;
char y = x^1;
NB! Please note that you may not add any additional methods or members to the classes of
question 1.1 – 1.4.
1.1.Write a back–end class called Page and save it in a file called Page.cpp. This class must have the
following structure:
Private Members
Data
Type
Name Description
string cSentences[] This member will store the sentences on the page.
You may assume that a record will never have more
than 34 sentences.
int cSentenceCounter This member will store the number of sentences on
the page.
Public Methods
Return
Type
Method Signature Description
Page () A default constructor that will initialise
cSentenceCounter to zero.
void addSentence(string
sentence)
This method will place add a sentence to the Page.
You must make use of the cSentences[] array.
string getSentenceAt(int index) This method will retrieve a sentence.
int getNumberOfSentences() This method will return the number of sentences
there are on the Page.
1.2.Write a back-end class called PageManager and save it in a file called PageManager.cpp. This
class must have the following structure:
Public Methods
Return
Type
Method Signature Description
Page loadPageFromFile(string
fileName)
This method must read the content of the
specified file into a Page object and return
this Page.
void writePageToFile(string fileName,
Page page)
This method must write the content of a
Page object into the specified file.
1.3.Write a back-end class called APM11A1Encryptor and save it in a file called
APM11A1Encryptor.cpp. This class must have the following structure:
Protected Members
Data
Type
Name Description
int key This member will store the key that will be used during
the encryption process. (You may NOT change the name
of this member)
Public Methods
Return
Type
Method Signature Description
char encryptACharacter(char
character)
This method must encrypt the received character
by using the key class member and the Caesar
Cipher encryption technique.
Page encryptPage(Page page) This method will encrypt the content of the
received page parameter by making use of the
encryptACharacter() method.
void setKey(int key) This method will set the value of the key data
member. (You may NOT change the name of the
input parameter)
1.4.Write a back-end class called AdvancedAPM11A1Encryptor and save it in a file called
AdvancedAPM11A1Encryptor.cpp. This class must inherit the functionality of the
APM11A1Encryptor class and add functionality that will enable us to make use of the ExclusiveOR encryption procedure. This class must have the following structure:
Inherited Data Members
Data
Type
Name Description
int key This member will store the key that will be used during
the encryption process.
Public Methods
Return
Type
Method Signature Description
char encryptACharacter(char
character)
This method must encrypt the received character
by using the key class member and the ExclusiveOR encryption technique.
Inherited Methods
Method Signature
encryptPage(Page page), setKey(int key)
1.5.Write a main program and save it in a file called Run.cpp. In this program you must read a text file
that contains sentences into a Page object, encrypt the content using the APM11A1Encryptor
class and then write the Page to a new text file called encrypt1.txt by using the PageManager
class.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

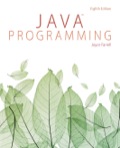
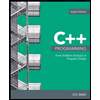
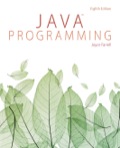
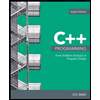