QUESTION 2 You have been hired under a student working scheme program. Your first task is to write a C program to assist in one of the professors in the faculty to grade the final exam of his/her students. The exam consists of 20 multiple-choice questions. Each question has one of four possible answers: A, B, C, or D. The program will read in the students' answers and the correct answers from files and prints out the result onto the screen and also the file output. There are at least 15 students in class. Input The students' answers are stored in a data file named "StudentAnswers.dat" as shown in Figure 2.1. In this file, the first column represents the students' name (1 word), second column represent the students' ID and the third column until the last column represent the students' answer of question 1 until question 20. In this example (Figure 1.1), there are only five students. You have to complete this input file with data at least for 15 students and cover all situations. The correct answers for all the questions are stored in a text file named "CorrectAnswers.txt" as shown in Figure 2.2. Output The program needs to determine and print out the following items on the screen as shown in Figure 2.3: o Ask the user to enter student's ID o Display the student's ID and student's name o Compare the student's answers and the correct answers. Calculate and display the total number of questions missed by the student. o Display the list of the questions missed by the students, showing the correct answers and the incorrect answers by the student for each missed question. o Calculate and display the percentage of questions answered correctly. This can be calculated as: percentage = correctly answered questions total number of questions x 100 o Display the grade of the students based on the percentage as follows: ▪ 80 ≤ percentage ≤ 100-grade is A ▪ 70 ≤ percentage < 80-grade is B ▪ 60 ≤ percentage < 70 - grade is C percentage < 60 grade is F
QUESTION 2 You have been hired under a student working scheme program. Your first task is to write a C program to assist in one of the professors in the faculty to grade the final exam of his/her students. The exam consists of 20 multiple-choice questions. Each question has one of four possible answers: A, B, C, or D. The program will read in the students' answers and the correct answers from files and prints out the result onto the screen and also the file output. There are at least 15 students in class. Input The students' answers are stored in a data file named "StudentAnswers.dat" as shown in Figure 2.1. In this file, the first column represents the students' name (1 word), second column represent the students' ID and the third column until the last column represent the students' answer of question 1 until question 20. In this example (Figure 1.1), there are only five students. You have to complete this input file with data at least for 15 students and cover all situations. The correct answers for all the questions are stored in a text file named "CorrectAnswers.txt" as shown in Figure 2.2. Output The program needs to determine and print out the following items on the screen as shown in Figure 2.3: o Ask the user to enter student's ID o Display the student's ID and student's name o Compare the student's answers and the correct answers. Calculate and display the total number of questions missed by the student. o Display the list of the questions missed by the students, showing the correct answers and the incorrect answers by the student for each missed question. o Calculate and display the percentage of questions answered correctly. This can be calculated as: percentage = correctly answered questions total number of questions x 100 o Display the grade of the students based on the percentage as follows: ▪ 80 ≤ percentage ≤ 100-grade is A ▪ 70 ≤ percentage < 80-grade is B ▪ 60 ≤ percentage < 70 - grade is C percentage < 60 grade is F
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question

Transcribed Image Text:QUESTION 2
You have been hired under a student working scheme program. Your first task is to write a C
program to assist in one of the professors in the faculty to grade the final exam of his/her students.
The exam consists of 20 multiple-choice questions. Each question has one of four possible
answers: A, B, C, or D. The program will read in the students' answers and the correct answers
from files and prints out the result onto the screen and also the file output. There are at least 15
students in class.
Input
The students' answers are stored in a data file named "StudentAnswers.dat" as shown in Figure
2.1. In this file, the first column represents the students' name (1 word), second column represent
the students' ID and the third column until the last column represent the students' answer of
question 1 until question 20. In this example (Figure 1.1), there are only five students. You have
to complete this input file with data at least for 15 students and cover all situations. The correct
answers for all the questions are stored in a text file named "CorrectAnswers.txt" as shown in
Figure 2.2.
Output
The program needs to determine and print out the following items on the screen as shown in
Figure 2.3:
o Ask the user to enter student's ID
o
Display the student's ID and student's name
o
Compare the student's answers and the correct answers. Calculate and display the total
number of questions missed by the student.
o Display the list of the questions missed by the students, showing the correct answers and
the incorrect answers by the student for each missed question.
o Calculate and display the percentage of questions answered correctly. This can be
calculated as:
percentage =
·
correctly answered questions
total number of questions
x 100
o Display the grade of the students based on the percentage as follows:
▪ 80 ≤ percentage ≤ 100-grade is A
70 ≤percentage < 80-grade is B
▪ 60 ≤percentage < 70 - grade is C
percentage <60-grade is F

Transcribed Image Text:The program needs to print out the following item onto the file output as shown in Figure 2.4:
o Display all students' name, students' ID, students' percentage and students' grade
The number of student is based on the input data from file "StudentAnswers.dat".
Use array (one-dimension or two-dimension) to store the input data from file and the output
data.
The program should be written in several user-defined functions for example readFile()
function to read input data, compareAnswer () function to check the student's answers,
printMissQuestion () to display the missed questions and the correct answer,
printReport () to display output onto the output file etc. Each function must be
implemented with the concept of parameter passing. Use appropriate arguments for each
function. Do not use global variables.
Abdullah A19EE0180 A BADAC CDAB CDAD C D A B C D
LuDong
Syarifah
Sivarajah
Wendy
A19EE0160 ABD DAC BDDB CAAD C D A B C D
A A D C B C D D A B C CAACDAD CD
C B C B A C C D B B C C A B C D C C C D
A C C D A B C CAAC DA A ADA B C D
AC12CS678
AC12CS123
B19EE0167
2
8
A B C D A B C D A B C D A B C D A B C D
EXAM RESULT
Name
Figure 2.1: file "StudentAnswers.dat"
Enter the student ID: B19EE0167
10
14
15
Figure 2.2: file "CorrectAnswers.txt"
Wendy
Student ID : B19EE0167
Number of questions missed: 5
List of the questions missed:
Question
Correct Answer
B
D
B
B
с
Percentage: 75%, GRED B
Student Answer
T
A
A
A
Figure 2.3: example of output on the screen
LIST OF STUDENTS AND GRADES
NAME
Abdullah
ID
PERCENTAGE GRADE
A19EE0180 85
A19EE0160 70
LuDong
Syarifah AC12CS678
Sivarajah
Wendy
55
AC12CS123 65
B19EE0167 75
A
B
F
с
B
Figure 2.4: example of output in the output file.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 8 images

Recommended textbooks for you
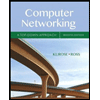
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
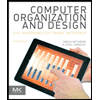
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
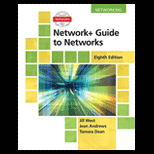
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
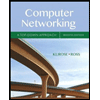
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
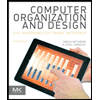
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
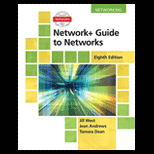
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
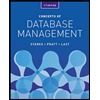
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
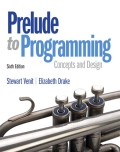
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
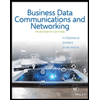
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY