Question - Create a class named Pizza with the following data fields: description - of type String price - of type double The description stores the type of pizza (such as sausage and onion). Include a constructor that requires arguments for both fields and a method named display to display the data. For example, if the description is 'sausage and onion' and the price is '14.99', the display method should output: sausage and onion pizza Price: $14.99 Create a subclass named DeliveryPizza that inherits from Pizza but adds the following data fields: deliveryFee - of type double address - of type String The description, price, and delivery address are required as arguments to the constructor. The delivery fee is $3 if the pizza ordered costs more than $15; otherwise it is $5. Code that is not accepted - class DeliveryPizza extends Pizza { private double deliveryFee; private String address; public DeliveryPizza(String desc, double price, double deliveryFee, String address){ super(desc, price); this.deliveryFee = deliveryFee; this.address = address; } public void displayto(){ super.displayto(); if(price>=15) { System.out.println("Delivery Fee: $3"+"\nAddress: "+address); } else System.out.println("Delivery Fee: $5"+"\nAddress: "+address); } } public class DemoPizzas { public static void main(String args[]) { // Write demo program here DeliveryPizza pizza1 = new DeliveryPizza("sausage and oninon", 14.99, 3, "78/383 Milenium Street, WI"); DeliveryPizza pizza2 = new DeliveryPizza("oninon", 17, 15, "8/3 Milenium Street, mI"); pizza1.displayto(); pizza2.displayto(); } } class Pizza { // Define the Pizza class here public String description; public double price; public Pizza(String desc, double price) { this.description = desc; this.price = price; } public void displayto() { System.out.printf("%s pizza Price: $%.2f\n", description, price); } } One of the Errors - Build Status Build Failed Build OutputNtTest4ada87be.java:10: error: cannot find symbol pizza.display(); ^ symbol: method display() location: variable pizza of type Pizza NtTest4ada87be.java:23: error: cannot find symbol pizza2.display(); ^ symbol: method display() location: variable pizza2 of type Pizza 2 errors Test Contents@Test public void unitTest() { Tester.replaceOutputStream(); Pizza pizza = new Pizza("Canadian bacon and pineapple", 20.99); pizza.display(); String output = Tester.getOutput(); String expected = "Canadian bacon and pineapple pizza Price: $20.99"; assertEquals(expected, output); } @Test public void unitTest2() { Tester.replaceOutputStream(); Pizza pizza2 = new Pizza("cheese", 10.01); pizza2.display(); String output = Tester.getOutput(); String expected = "cheese pizza Price: $10.01"; assertEquals(expected, output); } public static class Tester { private static java.io.ByteArrayOutputStream outputBytes; private static java.io.PrintStream old; public static void replaceOutputStream() { Tester.outputBytes = new java.io.ByteArrayOutputStream(); Tester.old = System.out; java.io.PrintStream ps = new java.io.PrintStream(Tester.outputBytes); System.setOut(ps); } public static String getOutput() { String output = Tester.outputBytes.toString(); output = output.trim(); System.out.flush(); System.setOut(Tester.old); return output; } }
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Question -
Create a class named Pizza with the following data fields:
- description - of type String
- price - of type double
The description stores the type of pizza (such as sausage and onion). Include a constructor that requires arguments for both fields and a method named display to display the data. For example, if the description is 'sausage and onion' and the price is '14.99', the display method should output:
sausage and onion pizza Price: $14.99
Create a subclass named DeliveryPizza that inherits from Pizza but adds the following data fields:
- deliveryFee - of type double
- address - of type String
The description, price, and delivery address are required as arguments to the constructor. The delivery fee is $3 if the pizza ordered costs more than $15; otherwise it is $5.
Code that is not accepted -

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

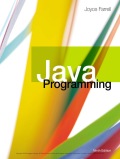
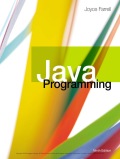