Write a class called Alien. The Alien class must have one field of type String called name, one of type String called planet and one field of type boolean called humanoid. Write one constructor that takes three parameters to initialise all of these fields. The parameters must be passed in the order name, planet and humanoid status. IntelliJ hint (this won't be in the real test): put your cursor after the last field; right-click, choose Generate, choose Constructor, select the fields that are set via parameters and click Ok. Check that the constructor is correct. Write a getter method for each field. IntelliJ hint (this won't be in the real test): put your cursor after the closing curly bracket of the constructor; right click, choose Generate, choose Getter, select all of the fields; click Ok. Check that the you have three getters. Do not write setters methods. Write a method called getDetails that will return the values of the fields in one of the following two formats. For example, either: Cyberman from Mondas (humanoid) or: Cyberman from Mondas Note that "Cyberman" is just one possible value of the name field and "Mondas" is just one possible value of the planet field. If the humanoid field has the value true then the planet details must be followed by the text "(humanoid)", as in the first example above. If the humanoid field has the value false then there will be no additional text, as in the second example above. Write a class called AlienList to store a ArrayList of Alien objects. ArrayList is a class in the java.util package that acts like a list. AlienList must have a method called addAlien that takes an Alien object as a parameter and adds it to the ArrayList. AlienList must have a method called printHumanoids. This method must print out the details of all the Alien objects in the ArrayList whose humanoid field has the value true. Details of each Alien must be printed on a separate line. The print out must use exactly the format shown in part 1 of the question. Write a method in AlienList called getNonHumanoids.This method must find all Alien objects in the AlienList object’s ArrayList whose humanoid field has the value false. It must do the following things with all the matching objects: The method must remove all the matching Alien objects from the ArrayList of AlienList. It must store all matching Alien objects in a new The new ArrayList must be created as a local variable in the method, which is then returned from the method. If there are no matching Alien objects then an empty ArrayList must be returned.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Write a class called Alien. The Alien class must have one field of type String called name, one of type String called planet and one field of type boolean called humanoid. Write one constructor that takes three parameters to initialise all of these fields. The parameters must be passed in the order name, planet and humanoid status. IntelliJ hint (this won't be in the real test): put your cursor after the last field; right-click, choose Generate, choose Constructor, select the fields that are set via parameters and click Ok. Check that the constructor is correct. Write a getter method for each field. IntelliJ hint (this won't be in the real test): put your cursor after the closing curly bracket of the constructor; right click, choose Generate, choose Getter, select all of the fields; click Ok. Check that the you have three getters. Do not write setters methods. Write a method called getDetails that will return the values of the fields in one of the following two formats.
For example, either:
Cyberman from Mondas (humanoid)
or:
Cyberman from Mondas
Note that "Cyberman" is just one possible value of the name field and "Mondas" is just one possible value of the planet field. If the humanoid field has the value true then the planet details must be followed by the text "(humanoid)", as in the first example above. If the humanoid field has the value false then there will be no additional text, as in the second example above. Write a class called AlienList to store a ArrayList of Alien objects. ArrayList is a class in the java.util package that acts like a list. AlienList must have a method called addAlien that takes an Alien object as a parameter and adds it to the ArrayList. AlienList must have a method called printHumanoids. This method must print out the details of all the Alien objects in the ArrayList whose humanoid field has the value true. Details of each Alien must be printed on a separate line. The print out must use exactly the format shown in part 1 of the question. Write a method in AlienList called getNonHumanoids.This method must find all Alien objects in the AlienList object’s ArrayList whose humanoid field has the value false. It must do the following things with all the matching objects: The method must remove all the matching Alien objects from the ArrayList of AlienList.
It must store all matching Alien objects in a new The new ArrayList must be created as a local variable in the method, which is then returned from the method. If there are no matching Alien objects then an empty ArrayList must be returned.

Step by step
Solved in 3 steps with 1 images

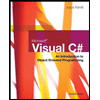
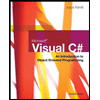