QUESTION PROVIDED BELOW KINDLY SEE. PROVIDE OUTPUT AS IT IS SHOWN IN QUESTION. AND FOLLOW TEMPLATES PROVIDED AT END OF QUESTION CHECK THIS BEFORE MAKING SOLUTION ( main.cpp , BikeService.cpp ) Write a C++ program to display the packages and the service cost. Strictly adhere to the Object-Oriented specifications given in the problem statement. All class names, member variable names, and function names should be the same as specified in the problem statement. Note: Use function overloading concept. Consider a class BikeService with the following private member variables. Datatype Variable string model string packageType Include the following methods in the class BikeService Method Description void bikeService(string packagetype) This method displays the available service based on the type of the package (either regular or additional). Note: If the package type is 1, display all the services corresponding to the regular package. If the package type is 2, display all the services corresponding to both the regular package and the additional package. float bikeService(int regularPackage) This method returns the service cost for the specified regular package. If the user desires to buy more than one package, then the service cost is the total of all selected package’s costs. float bikeService(int regularPackage,int addtionalPackage) This method returns the service cost for the specified regular package and additional package. If the user desires to buy more than one package, then the service cost is the total of all selected package’s costs. The service package and their cost are as follows: The service for regular packages are – Air Filter Cleaning – Rs. 1500 – Battery Check-Up and Distilled Water Top-Up – Rs. 1200 – Electrical Checking & Repair – Rs. 600 – Lights Checkup & Repair – Rs. 700 – Chain Adjustment & Cleaning – Rs. 400 – Handle Play Adjustment – Rs. 1000 – Tightening Of Bolts, Nuts,& Cylinder Head Checkup – Rs. 500 – Fuel Leakage Checking & Repair –Rs. 900 The service for additional packages are – Engine Oil – Rs. 500 – Water Wash – Rs. 400 – Battery Recharge – Rs. 300 In the main method get the inputs from the user and test the above class. Input and Output Format: Refer sample input and output for formatting specifications. All double values should be displayed correctly in two decimal places. [All text in bold corresponds to input and the rest corresponds to output] Sample Input and Output 1: Enter the model of the bike RoyalEnfield Select the service type 1.Regular 2.Additional Regular The service regular package 1.Air Filter Cleaning 2.Battery Check Up and Distilled Water Top-Up 3.Electrical Checking & Repair 4.Lights Checkup & Repair 5.Chain Adjustment & Cleaning 6.Handle Play Adjustment 7.Tightening Of Blots ,Nuts,& Cylinder Head Checkup 8.Fuel Leakage Checking & Repair Enter your package 1 Do you want to select any other package ? Yes Enter your package 2 Do you want to select any other package ? No The service cost is Rs.2700.00 Sample Input and Output 2: Enter the model of the bike Pulsar Select the service type 1.Regular 2.Additional Additional The service regular package 1.Air Filter Cleaning 2.Battery Check Up and Distilled Water Top-Up 3.Electrical Checking & Repair 4.Lights Checkup & Repair 5.Chain Adjustment & Cleaning 6.Handle Play Adjustment 7.Tightening Of Blots ,Nuts,& Cylinder Head Checkup 8.Fuel Leakage Checking & Repair The additional service package 1.Engine Oil 2.Water Wash 3.Battery Recharge Enter your regular package 1 Enter your additional package 3 Do you want to select any other package ? Yes Enter your regular package 3 Enter your additional package 2 Do you want to select any other package ? No The service cost is Rs.2800.00 ------------------------QUESTION ENDS--------------- --------------TEMPLATES BELOW--------------- main.cpp #include #include #include #include "BikeService.cpp" using namespace std; int main() { //Fill your code here } bikerservice.cpp #include using namespace std; class BikeService { private: string model; string packageType; public: void bikeService(string packagetype){ //Fill your code here } float bikeService(int regularPackage){ //Fill your code here } float bikeService(int regularPackage,int additionalPackage) { //Fill your code here } };
QUESTION PROVIDED BELOW KINDLY SEE. PROVIDE OUTPUT AS IT IS SHOWN IN QUESTION.
AND FOLLOW TEMPLATES PROVIDED AT END OF QUESTION CHECK THIS BEFORE MAKING SOLUTION ( main.cpp , BikeService.cpp )
Write a C++ program to display the packages and the service cost.
Strictly adhere to the Object-Oriented specifications given in the problem statement. All class names, member variable names, and function names should be the same as specified in the problem statement.
Note: Use function overloading concept.
Consider a class BikeService with the following private member variables.
Datatype | Variable |
string | model |
string | packageType |
Include the following methods in the class BikeService
Method | Description |
void bikeService(string packagetype) | This method displays the available service based on the type of the package (either regular or additional). Note: If the package type is 1, display all the services corresponding to the regular package. If the package type is 2, display all the services corresponding to both the regular package and the additional package. |
float bikeService(int regularPackage) | This method returns the service cost for the specified regular package. If the user desires to buy more than one package, then the service cost is the total of all selected package’s costs. |
float bikeService(int regularPackage,int addtionalPackage) | This method returns the service cost for the specified regular package and additional package. If the user desires to buy more than one package, then the service cost is the total of all selected package’s costs. |
The service package and their cost are as follows:
The service for regular packages are
– Air Filter Cleaning – Rs. 1500
– Battery Check-Up and Distilled Water Top-Up – Rs. 1200
– Electrical Checking & Repair – Rs. 600
– Lights Checkup & Repair – Rs. 700
– Chain Adjustment & Cleaning – Rs. 400
– Handle Play Adjustment – Rs. 1000
– Tightening Of Bolts, Nuts,& Cylinder Head Checkup – Rs. 500
– Fuel Leakage Checking & Repair –Rs. 900
The service for additional packages are
– Engine Oil – Rs. 500
– Water Wash – Rs. 400
– Battery Recharge – Rs. 300
In the main method get the inputs from the user and test the above class.
Input and Output Format:
Refer sample input and output for formatting specifications.
All double values should be displayed correctly in two decimal places.
[All text in bold corresponds to input and the rest corresponds to output]
Sample Input and Output 1:
Enter the model of the bike
RoyalEnfield
Select the service type
1.Regular
2.Additional
Regular
The service regular package
1.Air Filter Cleaning
2.Battery Check Up and Distilled Water Top-Up
3.Electrical Checking & Repair
4.Lights Checkup & Repair
5.Chain Adjustment & Cleaning
6.Handle Play Adjustment
7.Tightening Of Blots ,Nuts,& Cylinder Head Checkup
8.Fuel Leakage Checking & Repair
Enter your package
1
Do you want to select any other package ?
Yes
Enter your package
2
Do you want to select any other package ?
No
The service cost is Rs.2700.00
Sample Input and Output 2:
Enter the model of the bike
Pulsar
Select the service type
1.Regular
2.Additional
Additional
The service regular package
1.Air Filter Cleaning
2.Battery Check Up and Distilled Water Top-Up
3.Electrical Checking & Repair
4.Lights Checkup & Repair
5.Chain Adjustment & Cleaning
6.Handle Play Adjustment
7.Tightening Of Blots ,Nuts,& Cylinder Head Checkup
8.Fuel Leakage Checking & Repair
The additional service package
1.Engine Oil
2.Water Wash
3.Battery Recharge
Enter your regular package
1
Enter your additional package
3
Do you want to select any other package ?
Yes
Enter your regular package
3
Enter your additional package
2
Do you want to select any other package ?
No
The service cost is Rs.2800.00
------------------------QUESTION ENDS---------------
--------------TEMPLATES BELOW---------------
main.cpp
#include<cstring> #include<iostream> #include<string> #include "BikeService.cpp" using namespace std; int main() { //Fill your code here } |
bikerservice.cpp
#include<iostream> class BikeService public: float bikeService(int regularPackage){ float bikeService(int regularPackage,int additionalPackage) |

Step by step
Solved in 5 steps with 6 images

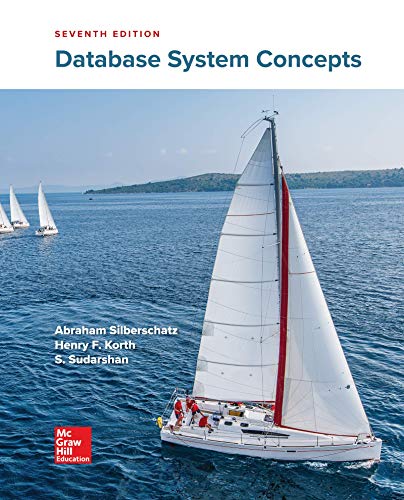
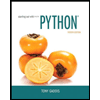
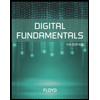
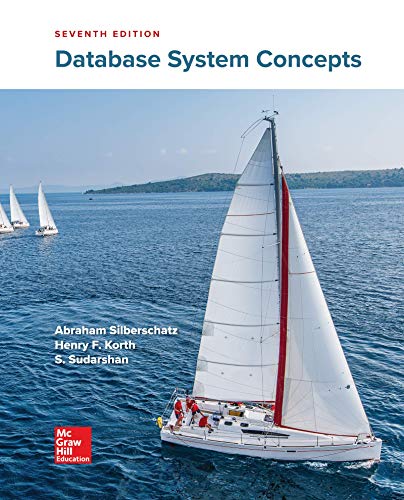
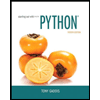
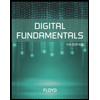
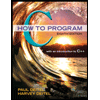
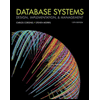
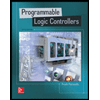