Question The system keeps track of available amount of flour to make cakes. It starts with inputting the quantity of 5kg flour from the user. If the quantity is invalid, it loops until the quantity is valid. Once the initial quantity is given, the user can perform the following activities. Each activity is done in a separate method: ● Make cake this method reads the quantity of cake to make. Each cake requires 400 grams of flour. It returns the quantity to update the main if input quantity is valid. It prompts error messages if quantity is invalid. ● Add flour - this method reads the quantity of 5Kg flour to add. It returns the value to update the main if input is valid. It prompts error message if input is invalid. • Check stock - this method displays the o total quantity of 5kg flour bought o the quantity of cakes made O the total gram of flour bought o the total gram of flour used o the total gram of flour remains. User can perform the activities repeatedly until a quit option is chosen. You are required to fulfill the following requirements: Write the program under only 1 class without using any object and object variables All methods are static methods. • • Provide input validation and prompt appropriate error messages for invalid input Use selection structure for selection options Use loop structure to handle looping of activities Handle each activity with a different method . Use only local variables to pass and return data needed to and from main. Below shows an example of the input and output of the program: Input the number of 5kg flour to start: -5 Invalid input, quantity must be positive Input the number of 5kg flour to start: 0 Invalid input, quantity must be positive Input the number of 5kg flour to start: 4 4 of 5kg flour added. Menu: 1. Make Cake 2. Add flour 3. Check record 1. Quit Invalid quantity Menu: 1. Make Cake 2. Add flour 3. Check record 4. Quit Enter selection (1-4): 1 Enter quantity of cake to make: 20 20 cakes made. flour left is 12000 gram Menu: 1. Make Cake 2. Add flour 3. Check record 4. Quit Enter selection (1-4): 1 Enter quantity of cake to make: 50 Insufficient flour. Available flour is 12000 gram Menu: 1. Make Cake 2. Add flour 3. Check record 4. Quit Enter selection (1-4): 2 How many 5kg flour to add? 5 5 of 5kg flour added. Menu: 1. Make Cake 2. Add flour 3. Check record 4. Quit Enter selection (1-4): 3 Total quantity of 5 kg flour bought: 9 Total quantity of cake made:20 Total flour bought: 45000gram Total flour used: 8000gram Total flour left: 37000gram Menu: 1. Make Cake 2. Add flour
Question The system keeps track of available amount of flour to make cakes. It starts with inputting the quantity of 5kg flour from the user. If the quantity is invalid, it loops until the quantity is valid. Once the initial quantity is given, the user can perform the following activities. Each activity is done in a separate method: ● Make cake this method reads the quantity of cake to make. Each cake requires 400 grams of flour. It returns the quantity to update the main if input quantity is valid. It prompts error messages if quantity is invalid. ● Add flour - this method reads the quantity of 5Kg flour to add. It returns the value to update the main if input is valid. It prompts error message if input is invalid. • Check stock - this method displays the o total quantity of 5kg flour bought o the quantity of cakes made O the total gram of flour bought o the total gram of flour used o the total gram of flour remains. User can perform the activities repeatedly until a quit option is chosen. You are required to fulfill the following requirements: Write the program under only 1 class without using any object and object variables All methods are static methods. • • Provide input validation and prompt appropriate error messages for invalid input Use selection structure for selection options Use loop structure to handle looping of activities Handle each activity with a different method . Use only local variables to pass and return data needed to and from main. Below shows an example of the input and output of the program: Input the number of 5kg flour to start: -5 Invalid input, quantity must be positive Input the number of 5kg flour to start: 0 Invalid input, quantity must be positive Input the number of 5kg flour to start: 4 4 of 5kg flour added. Menu: 1. Make Cake 2. Add flour 3. Check record 1. Quit Invalid quantity Menu: 1. Make Cake 2. Add flour 3. Check record 4. Quit Enter selection (1-4): 1 Enter quantity of cake to make: 20 20 cakes made. flour left is 12000 gram Menu: 1. Make Cake 2. Add flour 3. Check record 4. Quit Enter selection (1-4): 1 Enter quantity of cake to make: 50 Insufficient flour. Available flour is 12000 gram Menu: 1. Make Cake 2. Add flour 3. Check record 4. Quit Enter selection (1-4): 2 How many 5kg flour to add? 5 5 of 5kg flour added. Menu: 1. Make Cake 2. Add flour 3. Check record 4. Quit Enter selection (1-4): 3 Total quantity of 5 kg flour bought: 9 Total quantity of cake made:20 Total flour bought: 45000gram Total flour used: 8000gram Total flour left: 37000gram Menu: 1. Make Cake 2. Add flour
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
A basic java self-learner exercise, pls do use more // comment line to explain to me miss/sir, especially the method part I'm so confused that while it has more than 1 in it. Especially the criteria part need to be followed in the code .
Thanks miss/sir, pls do use more //comment line

Transcribed Image Text:Question
The system keeps track of available amount of flour to make cakes. It starts with inputting
the quantity of 5kg flour from the user. If the quantity is invalid, it loops until the quantity is
valid.
Once the initial quantity is given, the user can perform the following activities. Each activity
is done in a separate method:
●
Make cake - this method reads the quantity of cake to make. Each cake requires 400
grams of flour. It returns the quantity to update the main if input quantity is valid. It
prompts error messages if quantity is invalid.
Add flour - this method reads the quantity of 5Kg flour to add. It returns the value to
update the main if input is valid. It prompts error message if input is invalid.
Check stock - this method displays the
o
total quantity of 5kg flour bought
O
the quantity of cakes made
O
the total gram of flour bought
O
the total gram of flour used
O
the total gram of flour remains
User can perform the activities repeatedly until a quit option is chosen.
You are required to fulfill the following requirements:
Write the program under only 1 class without using any object and object variables
All methods are static methods.
Provide input validation and prompt appropriate error messages for invalid input
●
Use selection structure for selection options
Use loop structure to handle looping of activities
●
Handle each activity with a different method
●
Use only local variables to pass and return data needed to and from main.
Below shows an example of the input and output of the program:
Input the number of 5kg flour to start: -5
Invalid input, quantity must be positive
Input the number of 5kg flour to start: 0
Invalid input, quantity must be positive
Input the number of 5kg flour to start: 4
4 of 5kg flour added.
Menu:
1. Make Cake
2. Add flour
3. Check record
4. Quit
Enter selection (1-4): 1
Entor quantity of cake to make A
Invalid quantity
Menu:
1. Make Cake
2. Add flour
3. Check record
4. Quit
Enter selection (1-4): 1
Enter quantity of cake to make: 20
20 cakes made. flour left is 12000 gram
Menu:
1. Make Cake
2. Add flour
3. Check record
4. Quit
Enter selection (1-4): 1
Enter quantity of cake to make: 50
Insufficient flour.
Available flour is 12000 gram
Menu:
1. Make Cake
2. Add flour
3. Check record
4. Quit
Enter selection (1-4): 2
How many 5kg flour to add? 5
5 of 5kg flour added.
Menu:
1. Make Cake
2. Add flour
3. Check record
4. Quit
Enter selection (1-4): 3
Total quantity of 5 kg flour bought: 9
Total quantity of cake made:20
Total flour bought: 45000gram
Total flour used: 8000gram
Total flour left: 37000gram
Menu:
1. Make Cake
2. Add flour
3. Check record
4. Quit

Transcribed Image Text:self learn exercise
your code should include the following criteria
Assessment Criteria
Input/output
Variables, data types
Input/output
Validation
calculation
Selection structure
Loop structure
Make cake
Add flour
Check stock
Flow Control
Methods
Total
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
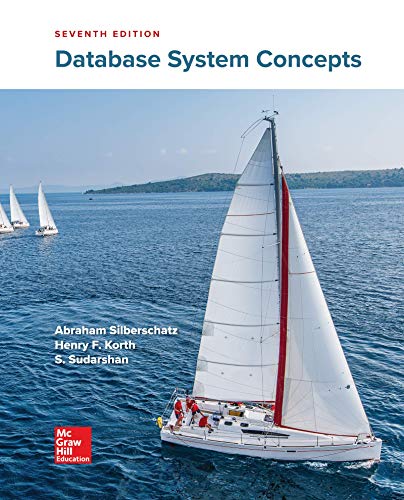
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
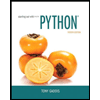
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
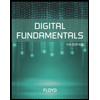
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
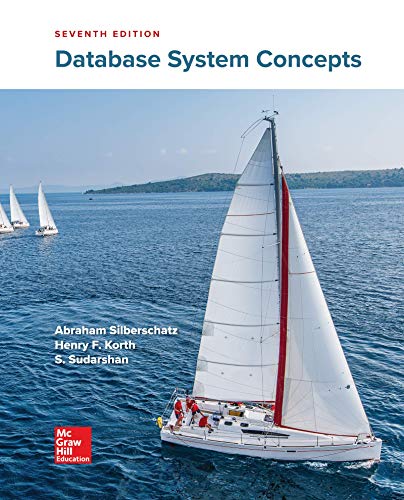
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
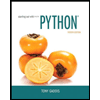
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
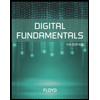
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
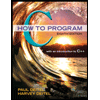
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
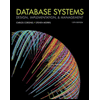
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
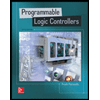
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education