Queue We are given a class stack. 1 class [ ’ a ] stack = object 2 val mutable seznam =([]: ’ a list ) 3 method empty =[]= seznam 4 method pop =let r = List . hd seznam in seznam < - List . tl seznam ; r 5 method push elm = seznam < - elm :: seznam 6 method reverse = seznam < - List . rev seznam 7 end ;; The above stack type includes the methods needed for this exercise. Note that the method reverse reverses the elements stored on the stack and the method empty returns true if and only if the stack is empty. Implement a class queue with the following type 1 class [ ’ a ] queue : 2 object 3 method dequeue : ’a 4 method enqueue : ’a -> unit 5 end 1You may use a free service accessible on https://try.ocamlpro.com/. 1 The class queue uses two instances of a class stack. The first stack is used for storing the inserted new element. The second stack is used for retrieving the elements from the queue. If the second stack is empty and the first is not, then the first is reversed and stored as the second. The method enqueue inserts an element to the beginning of a queue, and the method dequeue returns an element from the end of the queue. 1 # let q1 = new queue ;; 2 # q1 # enqueue 1 ;; 3 - : unit = () 4 # q1 # enqueue 2 ;; 5 - : unit = () 6 # q1 # dequeue ;; 7 - : int = 1 8 # q1 # dequeue ;; 9 - : int = 2 10 # let q2 = new queue ;; 11 # q2 # enqueue " First " ;; 12 - : unit = () 13 # q2 # dequeue ;; 14 - : string = " First " 15 # q2 # enqueue " Second " ;; 16 - : unit = () 17 # q2 # enqueue " Third " ;; 18 - : unit = () 19 # q2 # dequeue ;; 20 - : string = " Second " 21 # q2 # dequeue ;; 22 - : string = " Third "
Queue
We are given a class stack.
1 class [ ’ a ] stack = object
2 val mutable seznam =([]: ’ a list )
3 method empty =[]= seznam
4 method pop =let r = List . hd seznam in seznam < - List . tl
seznam ; r
5 method push elm = seznam < - elm :: seznam
6 method reverse = seznam < - List . rev seznam
7 end ;;
The above stack type includes the methods needed for this exercise. Note
that the method reverse reverses the elements stored on the stack and the
method empty returns true if and only if the stack is empty.
Implement a class queue with the following type
1 class [ ’ a ] queue :
2 object
3 method dequeue : ’a
4 method enqueue : ’a -> unit
5 end
1You may use a free service accessible on https://try.ocamlpro.com/.
1
The class queue uses two instances of a class stack. The first stack is used
for storing the inserted new element. The second stack is used for retrieving
the elements from the queue. If the second stack is empty and the first is not,
then the first is reversed and stored as the second. The method enqueue inserts
an element to the beginning of a queue, and the method dequeue returns an
element from the end of the queue.
1 # let q1 = new queue ;;
2 # q1 # enqueue 1 ;;
3 - : unit = ()
4 # q1 # enqueue 2 ;;
5 - : unit = ()
6 # q1 # dequeue ;;
7 - : int = 1
8 # q1 # dequeue ;;
9 - : int = 2
10 # let q2 = new queue ;;
11 # q2 # enqueue " First " ;;
12 - : unit = ()
13 # q2 # dequeue ;;
14 - : string = " First "
15 # q2 # enqueue " Second " ;;
16 - : unit = ()
17 # q2 # enqueue " Third " ;;
18 - : unit = ()
19 # q2 # dequeue ;;
20 - : string = " Second "
21 # q2 # dequeue ;;
22 - : string = " Third "

Step by step
Solved in 4 steps with 2 images

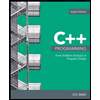
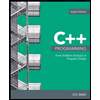