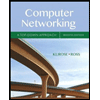
For the Queue use the java interface Queue with the ArrayDeque class
For the Stack use the java Deque interface with the ArrayDeque class
For the LinkedList, use the java LinkedList class
Also, when you are asked to create and use a Queue, you can use only those methods pertaining to the general operations of a queue (Enqueue: addLast, Dequeue: removeFirst, and peek()
Similarly, when you are asked to create and/or use a stack, you can use only those methods pertaining to the general operations of a Stack (push: addFirst, pop:removeFirst, and peek() )
Your code should not only do the job, but also it should be done as efficiently as possible: fast and uses no additional memory if at all possible
Questions
- Write a method “int GetSecondMax(int[] array)” . this method takes an array of integers and returns the second max value
Example: if the array contains: 7, 2, 9, 5, 4, 15, 6, 1}. It should return the value: 9

- Start.
- Read the inputs.
- Perform the operations.
- Print the output.
- Exit.
Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 1 images

- Each of the iterators must implement the java.util.Iterator interface by providing the two public methods hasNext () and next(). The Iterator interface also lists two additional methods, remove () and forEachRemaining (). These are marked as default, so the interface itself does not require implementation. Of these methods, this assignment only requires you to implement the remove () method of the linked list iterator. 1 ArrayList iterator This is an external iterator, meaning it is a public class named ICS211ArrayListIterator, and and the code is not part of the ArrayList class. Your ICS211ArrayListIterator class should have a constructor ICS211ArrayListIterator(java.util.ArrayList gives access to all the elements of the array list. This array must be saved as the value of a class variable of the iterator. data). The constructor must call one of the two toArray methods of ArrayList to obtain an array that Other than this single call to java.util.ArrayList.toArray in the constructor,…arrow_forwardCreate an implementation of each LinkedList, Queue Stack interface provided For each implementation create a tester to verify the implementation of thatdata structure performs as expected Your task is to: Implement the LinkedList interface ( fill out the implementation shell). Put your implementation through its paces by exercising each of the methods in the test harness Create a client ( a class with a main ) ‘StagBusClient’ which builds a bus route by performing the following operations on your linked list: Create (insert) 4 stations List the stations Check if a station is in the list (print result) Check for a station that exists, and one that doesn’t Remove a station List the stations Add a station before another station. List the stations Add a station after another station. Print the stations StagBusClient.java package app; import linkedList.LinkedList; import linkedList.LinkedListImpl; public class StagBusClient { public static void main(String[] args) { // create…arrow_forwardAdd the three classic operations of Queues in the MagazineList.java to make it become a queue. Add testing code in MagazineRack.java to test your code. (You do not need to modify Magazine.java) Magazine.java : public class Magazine {private String title;//-----------------------------------------------------------------// Sets up the new magazine with its title.//-----------------------------------------------------------------public Magazine(String newTitle){ title = newTitle;}//-----------------------------------------------------------------// Returns this magazine as a string.//-----------------------------------------------------------------public String toString(){return title;}} MagazineList.java : attached the image below MagazineRack.java: public class MagazineRack{//----------------------------------------------------------------// Creates a MagazineList object, adds several magazines to the// list, then prints…arrow_forward
- JAVA please Given main() in the ShoppingList class, define an insertAtEnd() method in the ItemNode class that adds an element to the end of a linked list. DO NOT print the dummy head node. Ex. if the input is: 4 Kale Lettuce Carrots Peanuts where 4 is the number of items to be inserted; Kale, Lettuce, Carrots, Peanuts are the names of the items to be added at the end of the list. The output is: Kale Lettuce Carrots Peanuts Code provided in the assignment ItemNode.java:arrow_forwardA stack-ended queue, sometimes known as a steque, is a data type that allows push, pop, and enqueue operations. Make an API for this ADT. Create a linked-list implementation.arrow_forwardComplete the code for the removeFirst method, which should remove and return the first element in the linked list. Throw aNoSuchElementException if the method is invoked on an empty list. import java.util.NoSuchElementException; public class LinkedList { private Node first; public LinkedList() { first = null; } public Object getFirst() { if (first == null) { throw new NoSuchElementException(); } return first.data; } public Object removeFirst() { // put your code here } class Node { public Object data; public Node next; } }arrow_forward
- Given main() and an IntNode class, complete the IntList class (a linked list of IntNodes) by writing the insertInAscendingOrder() method that inserts a new IntNode into the IntList in ascending order. Ex. If the input is: 8 3 6 2 5 9 4 1 7 -1 the output is: 1 2 3 4 5 6 7 8 9 import java.util.Scanner; public class SortedList {public static void main (String[] args) {Scanner scnr = new Scanner(System.in);IntList intList = new IntList();IntNode curNode;int num;num = scnr.nextInt();// Read in until -1while (num != -1) {// Insert into linked listcurNode = new IntNode(num);intList.insertInAscendingOrder(curNode);num = scnr.nextInt();}intList.printIntList();}}arrow_forwardjava Suppose queue is defined as LinkedQueue<Integer> queue = new LinkedQueue<>(); Show what is written by the following segment of code. SHOW YOUR WORK.arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
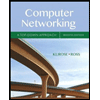
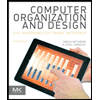
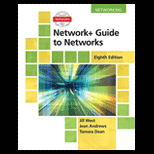
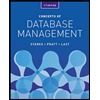
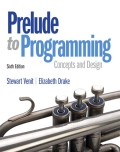
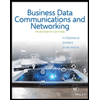