Recall that the distance formula is: sqrt( (x2−x1)^2+(y2−y1)^2 ) The input consists of a single line containing six space-separated integer values: x, y, x1, y1, x2, and y2. You are guaranteed that x1
You have a fence post located at the point (x,y) where a goat is tethered by a rope. You also have a house, which is a rectangle with diagonally opposite corners at the points bottom-left: (x1,y1) and top-right: (x2,y2). You want to pick a length of rope that guarantees the goat cannot reach the house.
Determine the minimum distance from the fence post to the house, so that you can make sure to use a shorter rope.
Recall that the distance formula is: sqrt( (x2−x1)^2+(y2−y1)^2 )
The input consists of a single line containing six space-separated integer values: x, y, x1, y1, x2, and y2.
You are guaranteed that x1<x2 and y1<y2, and that (x,y) is strictly outside the axis-aligned rectangle with corners at (x1,y1) and (x2,y2).
Return the minimum distance from the goat’s post to the house as a floating-point value from main().
Learning Objectives
- Be able to create a program with a lesser template.
- Be able to calculate the min/max of integers.
- Be able to write a mathematical calculation (distance formula).
Template
def get_input():
# TODO: Get six inputs from the user
# TODO: Return all six inputs as (x, y) float pairs
# (x, y) is a tuple, which can be created using parentheses ()
datalist = input().split(" ")
post = (float(datalist[0]), float(datalist[1]))
# TODO: Finish getting input (bottom left and top right)
bl = (..., ...)
tr = (..., ...)
# Return the post, bottom-left, and top-right as tuples
return post, bl, tr
def rope(post, bl, tr):
# post is the (x, y) coordinates of the goat's post
# bl is the Bottom-Left coordinate (l, b)
# tr is the Top-Right coordinate (r, t)
# TODO: Calculate the shortest amount for the goat's rope
shortest_rope_length = 0
# Return the amount back to main.
return shortest_rope_length
if __name__ == "__main__": post, bl, tr = get_input() rope_length = rope(post, bl, tr) print("{:.3f}".format(rope_length))
Assignment
Step 1
Write a function called get_input().
def get_input():
- All input is on one line. However, there are 6 integers on that one line.
- Return three tuples. The first will be (x, y), the second (x1,y1), and the third (x2, y2).
- All input needs to be converted to floats.
Step 2
Write a function called rope().
def rope(post, bl, tr):
- The parameter post is a tuple (x, y) that contains the coordinates for the fence post.
- The parameter bl is the bottom-left coordinate of the house in (x1,y1) format.
- The parameter tr is the top-right coordinate of the house in (x2,y2) format.
- Recall that all coordinates are floating point values.
- Calculate the the minimum rope length needed so that the goat tied to post (x, y) cannot reach the house.
- Return the minimum length of rope as a float.
Step 3
Test your code.
if __name__ == "__main__":
post, bl, tr = get_input()
rope_length = rope(post, bl, tr)
print("{:.3f}".format(rope_length))
Make sure you test with more input values than just those given in the sample.
Sample
Given the input:
7 3 0 0 5 4
Your code should produce.
2.000
Hints and Tips
- The house can be thought of as a rectangle. You are given the bottom-left and upper-right coordinates of the rectangle, so you know the perimeter of the house.
- The goat's post is given an x,y coordinate.
- Think of this problem as a min-max problem.
- If the goat is on the left side of the house, then the post's x coordinate will be < the house's left coordinate.
- If the goat is on the right side of the house, then the post's x coordinate will be > the house's right coordinate.
- Think about if the goat is above the house (post's y > house's y).
- Your job is to find the distance between the house and the post.
- The post is a single point (x, y), but the house is a rectangle, so it has points left >= x >= right and top >= y >= bottom.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

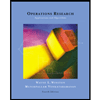
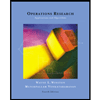