Recall that the mean T and the standard deviation ơ, are defined as N と;-ア)? ri Or i=1 i=1 and that the median î is defined as the value for which half of the scores are above it and half of the scores are below it. To compute the median, you first need to sort the array. If N is odd, then r is the element r; in the middle of the sorted array; if N is even, then F is the average of the two elements r; and ri+1 in the middle of the sorted array (if you find this confusing, just check Wikipedia, or come to office hours). Your program should be called qualityControl.cpp. You should copy-paste the following code and leave it untouched: #include #include using namespace std; float mean(float*, int); float stdev(float*, int); float median (float*, int); void top2scores (float*, int, float&, float&); void sort(float*, int); int main(void) { // Array for storing the test results cout << "How many test objects do you have? " int n; cin >> n; float* res = new float [n] ; // dynamically allocated memory for res[] // Read in the test results cout << "Enter the " くく n <く " test results: for (int i=0; i> res[i] ; // Print out cout << "Summary test scores: << endl ; %3D " << mean(res, n) << endl ; << stdev(res, n) << endl ; " < median(res, n) << endl ; cout << " mean cout << " stdev cout << median = float bestScore, secondBestScore; top2scores (res, n, bestScore, secondBestScore); cout << " top-2 = " << bestScore <« " " « secondBestScore « endl; return 0; Sample output is: How many test objects do you have? 6 Enter the 6 test results: 43 66 57 29 32 39 Summary test scores: = 44.3333 mean stdev 14.473 median = 41 top-2 = 66 57 Your task is to write the 5 functions needed to complete the program, namely: mean (), stdev(), median(), top2scores (), and sort (). Hints: 1. For sort (), use the provided bubbleSort() function (we will also cover this file in lecture). 2. The mean () function should be straightforward. 3. When writing the stdev() function, you can save yourself work by calling mean() from within stdev () to compute the mean. I know we haven't called functions from within functions, but that is totally acceptable in programming – and it saves you work. 4. To implement median() you will need to be able to determine if a number is even or odd. You can do this the hard way, or the easy way. The easy way makes use of the modulo operator, % (think about it). 5. You will also want to call sort() from within median(). 6. You should think about how to make use of sort () when writing the top2scores() function as well!
Recall that the mean T and the standard deviation ơ, are defined as N と;-ア)? ri Or i=1 i=1 and that the median î is defined as the value for which half of the scores are above it and half of the scores are below it. To compute the median, you first need to sort the array. If N is odd, then r is the element r; in the middle of the sorted array; if N is even, then F is the average of the two elements r; and ri+1 in the middle of the sorted array (if you find this confusing, just check Wikipedia, or come to office hours). Your program should be called qualityControl.cpp. You should copy-paste the following code and leave it untouched: #include #include using namespace std; float mean(float*, int); float stdev(float*, int); float median (float*, int); void top2scores (float*, int, float&, float&); void sort(float*, int); int main(void) { // Array for storing the test results cout << "How many test objects do you have? " int n; cin >> n; float* res = new float [n] ; // dynamically allocated memory for res[] // Read in the test results cout << "Enter the " くく n <く " test results: for (int i=0; i> res[i] ; // Print out cout << "Summary test scores: << endl ; %3D " << mean(res, n) << endl ; << stdev(res, n) << endl ; " < median(res, n) << endl ; cout << " mean cout << " stdev cout << median = float bestScore, secondBestScore; top2scores (res, n, bestScore, secondBestScore); cout << " top-2 = " << bestScore <« " " « secondBestScore « endl; return 0; Sample output is: How many test objects do you have? 6 Enter the 6 test results: 43 66 57 29 32 39 Summary test scores: = 44.3333 mean stdev 14.473 median = 41 top-2 = 66 57 Your task is to write the 5 functions needed to complete the program, namely: mean (), stdev(), median(), top2scores (), and sort (). Hints: 1. For sort (), use the provided bubbleSort() function (we will also cover this file in lecture). 2. The mean () function should be straightforward. 3. When writing the stdev() function, you can save yourself work by calling mean() from within stdev () to compute the mean. I know we haven't called functions from within functions, but that is totally acceptable in programming – and it saves you work. 4. To implement median() you will need to be able to determine if a number is even or odd. You can do this the hard way, or the easy way. The easy way makes use of the modulo operator, % (think about it). 5. You will also want to call sort() from within median(). 6. You should think about how to make use of sort () when writing the top2scores() function as well!
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter8: Arrays And Strings
Section: Chapter Questions
Problem 15PE
Related questions
Question
![Recall that the mean T and the standard deviation ơ, are defined as
N
と;-ア)?
ri
Or
i=1
i=1
and that the median î is defined as the value for which half of the scores are above it and half
of the scores are below it. To compute the median, you first need to sort the array. If N is odd,
then r is the element r; in the middle of the sorted array; if N is even, then F is the average of the
two elements r; and ri+1 in the middle of the sorted array (if you find this confusing, just check
Wikipedia, or come to office hours).
Your program should be called qualityControl.cpp. You should copy-paste the following code
and leave it untouched:
#include <iostream>
#include <cmath>
using namespace std;
float mean(float*, int);
float stdev(float*, int);
float median (float*, int);
void top2scores (float*, int, float&, float&);
void sort(float*, int);
int main(void) {
// Array for storing the test results
cout << "How many test objects do you have? "
int n;
cin >> n;
float* res
= new float [n] ;
// dynamically allocated memory for res[]
// Read in the test results
cout << "Enter the "
くく n <く "
test results:
for (int i=0; i<n; i++)
cin >> res[i] ;
// Print out](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F647a8de4-7140-4dc0-b12a-1ffdfefde701%2F56a3ae13-5ce3-4dd8-bf3b-8de857b0c976%2Fdoij71n.png&w=3840&q=75)
Transcribed Image Text:Recall that the mean T and the standard deviation ơ, are defined as
N
と;-ア)?
ri
Or
i=1
i=1
and that the median î is defined as the value for which half of the scores are above it and half
of the scores are below it. To compute the median, you first need to sort the array. If N is odd,
then r is the element r; in the middle of the sorted array; if N is even, then F is the average of the
two elements r; and ri+1 in the middle of the sorted array (if you find this confusing, just check
Wikipedia, or come to office hours).
Your program should be called qualityControl.cpp. You should copy-paste the following code
and leave it untouched:
#include <iostream>
#include <cmath>
using namespace std;
float mean(float*, int);
float stdev(float*, int);
float median (float*, int);
void top2scores (float*, int, float&, float&);
void sort(float*, int);
int main(void) {
// Array for storing the test results
cout << "How many test objects do you have? "
int n;
cin >> n;
float* res
= new float [n] ;
// dynamically allocated memory for res[]
// Read in the test results
cout << "Enter the "
くく n <く "
test results:
for (int i=0; i<n; i++)
cin >> res[i] ;
// Print out

Transcribed Image Text:cout << "Summary test scores:
<< endl ;
%3D
" << mean(res, n) << endl ;
<< stdev(res, n) << endl ;
" < median(res, n) << endl ;
cout << "
mean
cout << "
stdev
cout <<
median =
float bestScore, secondBestScore;
top2scores (res, n, bestScore, secondBestScore);
cout << " top-2 = " << bestScore <« " " « secondBestScore « endl;
return 0;
Sample output is:
How many test objects do you have? 6
Enter the 6 test results: 43 66 57 29 32 39
Summary test scores:
= 44.3333
mean
stdev
14.473
median
= 41
top-2
= 66 57
Your task is to write the 5 functions needed to complete the program, namely:
mean (), stdev(), median(), top2scores (), and sort ().
Hints:
1. For sort (), use the provided bubbleSort() function (we will also cover this file in lecture).
2. The mean () function should be straightforward.
3. When writing the stdev() function, you can save yourself work by calling mean() from within
stdev () to compute the mean. I know we haven't called functions from within functions, but that
is totally acceptable in programming – and it saves you work.
4. To implement median() you will need to be able to determine if a number is even or odd.
You can do this the hard way, or the easy way. The easy way makes use of the modulo operator, %
(think about it).
5. You will also want to call sort() from within median().
6. You should think about how to make use of sort () when writing the top2scores() function as
well!
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 5 images

Recommended textbooks for you
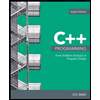
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
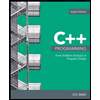
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning