Remove any word that does not start with a capital letter. For instance, for the input text of "Hello, 2022 world!", we should get "Hello, 2022 !". Notes: As in the previous question, you can safely assume that the set of characters that will be used will be only among the following: alphabetical characters [A-Za-z] (upper and lower case English letters), numerics [0-9] (digits), the space character [ ], and the following 6 punctuations: [.,!?;:]. You can define a word as follows: starts with a letter [A-Za-z], then proceeds with alphanumeric characters [A-Za-z0-9] until hits a non alphanumeric character (so a space character or a punctuation). However, if for simplicity, you take each word to be allowed to start with numeric characters too, that is fine as well (your outputs will be considered as valid). So for instance, the words like 2way, 4door, etc or even numbers like 2022 are also valid words. STARTER CODE: .data buffer_for_input_string: .space 100 buffer_for_processed_string: .space 100 prompt_for_input: .asciiz "Please enter your string:\n" prompt_for_output: .asciiz "Your processed string is as follows:\n" .text main: # prompting the user with a message for a string input: li $v0, 4 la $a0, prompt_for_input syscall # reading the input string and putting it in the memory: # the starting address of the string is accessible as buffer_for_input_string # 100 is the hard-coded maximum length of the null-terminated string that is # going to be read from the input. So effectively, up to 99 ascii characters. li $v0, 8 la $a0, buffer_for_input_string li $a1, 100 syscall # >>>> MAKE YOUR CHANGES BELOW HERE: # Looping over characters of the string: la $t0, buffer_for_input_string la $t1, buffer_for_processed_string Loop: lb $t2, 0($t0) # potentially do some processing on the character loaded in t2 sb $t2, 0($t1) addi $t0, $t0, 1 addi $t1, $t1, 1 bne $t2, $zero, Loop # keep going until you reach the end of the string, # which is demarcated by the null character. # <<<< MAKE YOUR CHANGES ABOVE HERE # prompting the user with a message for the processed output: li $v0, 4 la $a0, prompt_for_output syscall # printing the processed output # note that v0 already holds 4, the syscall code for printing a string. la $a0, buffer_for_processed_string syscall # Finish the programme: li $v0, 10 # syscall code for exit syscall # exit
Your Task:
Remove any word that does not start with a capital letter. For instance, for the input text of "Hello, 2022 world!", we should get "Hello, 2022 !".
Notes:
- As in the previous question, you can safely assume that the set of characters that will be used will be only among the following: alphabetical characters [A-Za-z] (upper and lower case English letters), numerics [0-9] (digits), the space character [ ], and the following 6 punctuations: [.,!?;:].
- You can define a word as follows: starts with a letter [A-Za-z], then proceeds with alphanumeric characters [A-Za-z0-9] until hits a non alphanumeric character (so a space character or a punctuation). However, if for simplicity, you take each word to be allowed to start with numeric characters too, that is fine as well (your outputs will be considered as valid). So for instance, the words like 2way, 4door, etc or even numbers like 2022 are also valid words.
STARTER CODE:
.data buffer_for_input_string: .space 100 buffer_for_processed_string: .space 100 prompt_for_input: .asciiz "Please enter your string:\n" prompt_for_output: .asciiz "Your processed string is as follows:\n" .text main: # prompting the user with a message for a string input: li $v0, 4 la $a0, prompt_for_input syscall # reading the input string and putting it in the memory: # the starting address of the string is accessible as buffer_for_input_string # 100 is the hard-coded maximum length of the null-terminated string that is # going to be read from the input. So effectively, up to 99 ascii characters. li $v0, 8 la $a0, buffer_for_input_string li $a1, 100 syscall
# >>>> MAKE YOUR CHANGES BELOW HERE: # Looping over characters of the string: la $t0, buffer_for_input_string la $t1, buffer_for_processed_string Loop: lb $t2, 0($t0) # potentially do some processing on the character loaded in t2 sb $t2, 0($t1) addi $t0, $t0, 1 addi $t1, $t1, 1 bne $t2, $zero, Loop # keep going until you reach the end of the string, # which is demarcated by the null character.
# <<<< MAKE YOUR CHANGES ABOVE HERE
# prompting the user with a message for the processed output: li $v0, 4 la $a0, prompt_for_output syscall # printing the processed output # note that v0 already holds 4, the syscall code for printing a string. la $a0, buffer_for_processed_string syscall # Finish the programme: li $v0, 10 # syscall code for exit syscall # exit

Step by step
Solved in 2 steps

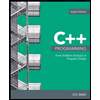
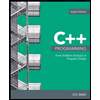