Requirement 4: DESCENDING ORDER (NOT ASCENDING ORDER) 4. Produce a list of all vaccines and their distributed quantities . The system should allow the employees to list all distributed vaccines and their accumulated quantities read from the dist. txt file. Note: The vaccines and their distributed quantities need to be sorted in descending order (with highest quantity listed first followed by second highest and so on) using Bubble sort before displaying on the screen.
Add distribution function in code
- Requirement 4: DESCENDING ORDER (NOT ASCENDING ORDER) 4. Produce a list of all vaccines and their distributed quantities . The system should allow the employees to list all distributed vaccines and their accumulated quantities read from the dist. txt file. Note: The vaccines and their distributed quantities need to be sorted in descending order (with highest quantity listed first followed by second highest and so on) using Bubble sort before displaying on the screen.
MY CODE:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// Function Declarations
void create_inventory();
void update_vacc_qty();
int search_vaccine();
void display_vaccine();
// Main Function starts here
int main()
{
create_inventory();
display_vaccine();
search_vaccine();
//update_vacc_qty();
return 0;
}
//Function to Create Vaccine.txt as per the given table
void create_inventory()
{
int option = 1;
// variables to collect data as per table given
char vaccName[15];
char vaccCode[2];
char country[15];
int qty;
float populaion;
//File definition
FILE *infile;
infile = fopen("Vaccine.txt","w"); // file opening for writing
if(infile == NULL) // Checking for the file creation
{
printf("Vaccine.txt file not found\n");
}
//Accepting data from user from keyboard till user enters 0 to close
while(option != 0)
{
printf("Enter Vaccine Name : ");
scanf("%s",vaccName);
printf("Enter Vaccine Code : ");
scanf("%s",vaccCode);
printf("Enter Counry : ");
scanf("%s",country);
printf("Enter Dosage Required : ");
scanf("%d",&qty);
printf("Enter Population Covered : ");
scanf("%f",&populaion);
//writing to the file using fprintf command
fprintf(infile,"%s %s %s %d %3.2f\n",
vaccName,vaccCode,country,qty,populaion);
printf("\nEnter 1 to continue and 0 to exit : ");
scanf("%d",&option);
if(option == 0)
fclose(infile); // closing the file when user wants to exit
}
}
//Function to display the file contents in a formatted way
void display_vaccine()
{
// variables to collect data as per table given
char vaccName[15];
char vaccCode[2];
char country[15];
int qty;
float populaion;
FILE *infile;
infile = fopen("Vaccine.txt","r"); // file opening for reading
if(infile == NULL) //checking for file exists or not
{
printf("Vaccine.txt file not found\n");
}
//printing the header line
printf("%15s\t%2s\t%15s\t%6s\t%10s\n","Vaccine Name","Vaccine Code","Country","Dosage","Population");
// Reading the file
while(fscanf(infile,"%s %s %s %d %f\n",vaccName,vaccCode,country,&qty,&populaion) !=EOF)
{
//printing the read data in a formatted way
printf("%15s\t%13s\t%15s\t%d\t%3.2f\n",vaccName,vaccCode,country,qty,populaion);
}
fclose(infile); // closing the file
}
void update_vacc_qty()
{
// to be coded
}
int search_vaccine()
{
// variables to collect data as per table given
char vaccName[15];
char vaccCode[2];
char country[15];
int qty;
float populaion;
FILE *infile;
char vcode[2];
char temp[2];
int value;
infile = fopen("Vaccine.txt","r"); // file opening for reading
//getting the vaccine code from user through keyboard to search
printf("Enter Vaccine Code to Search : ");
scanf("%s",vcode);
if(infile == NULL) // checking for file existence
{
printf("Vaccine.txt file not found\n");
}
strcpy(temp,vcode);
//Reading the file
while(fscanf(infile,"%s %s %s %d %f\n",vaccName,vaccCode,country,&qty,&populaion) !=EOF)
{
//checking user entered vaccine code and available in the file is same
if(vaccCode[0] == temp[0] && vaccCode[1] == temp[1])
{
// Printing the matched record
printf("%15s\t%2s\t%15s\t%6s\t%10s\n","Vaccine Name"," Vaccine Code","Country","Dosage","Population");
printf("%15s\t%13s\t%15s\t%d\t%3.2f\n",vaccName,vaccCode,country,qty,populaion);
}
}
fclose(infile);// closing the file
}//end of Program

Step by step
Solved in 3 steps with 1 images

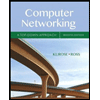
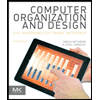
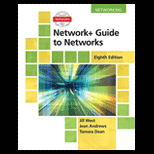
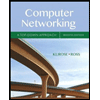
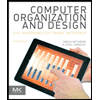
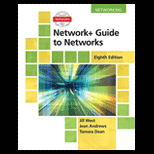
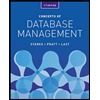
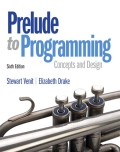
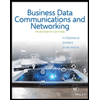