1. Need to save all the inputted data in .txt file (example reservation.txt) 2. compute the total of adults in generate report 3. compute the total of children in generate report 4. compute the total payment in generate report 5. If DELETE RESERVATION is selected, the user needs to input the reservation number to remove the existing reservation. 6. If the GENERATE REPORT is clicked, display the report of all reservations: reservation = {} rnum = 0 while True: adults = {} Date = {} Time = {} name = {} Children = {} total = {} mylist = [] choice = input( '\nRESTAURANT RESERVATION SYSTEM\nSystem Menu\nA = View all Reservations\nB = Make Reservation\nC = Delete Reservation\nD = Generate Report\nE = Exit\nEnter choice --> ').lower() f1 = open("reservation.txt", "a") if choice in 'Aa': f2 = open("reservation.txt", "r") print("Records are:") print(f"#\t\tDate\tTime\t\tName\t\tTotal Adults\t\tTotal children\t\tSubtotal\t\t") print(f2.read()) f2.close() elif choice in 'Bb': try: rnum += 1 name = input("Enter Name: ") Date = input("Enter date(DD-MM-YYYY): ") Time = input("Enter time(HH:MM): ") adults = input("Number of adults: ") Children = input("Number os children: ") f1.write(str(int(rnum))) f1.write("\t") f1.write(Date) f1.write("\t") f1.write(Time) f1.write("\t\t") f1.write(name) f1.write("\t\t") f1.write(adults) f1.write("\t\t\t\t") f1.write(Children) f1.write("\t\t\t\t\t") total = int(adults) * 500 + int(Children) * 300 reservation[rnum] = [Date, Time, name, adults, Children, total] f1.write(str(total)) f1.write("\n") f1.close() print("\nBelow are your Reservation Details:") print( f"#\t{rnum}\nName\t\t{name}\nDate\t\t{Date}\nTime\t\t{Time}\nTotal Adults\t\t{adults}\nTotal children\t\t{Children}\nSubtotal\t\t{total}\n") print(f1.read()) except ValueError: print("there is an incorrect value!! try again") elif choice in 'Cc': try: rnumd = int(input("Enter Reservation number:")) reservation[rnum] = open("reservation.txt", "r+") reservation[rnum].truncate(rnumd) print("No Record found") except KeyError: print("Invalid reservation ID") print("Reservation deleted") elif choice in 'Dd': f2 = open("reservation.txt", "r+") values = adults.values() Atotal = sum(values) print("Records are:") print(f"#\t\tDate\t\tTime\t\tDate\t\tTotal Adults\t\tTotal children\t\t") print(f2.read()) print(f"Total Number of Adults: " + str(Atotal)) print("Total Number of Children: ") print("Grand Total : ") f2.close() elif (choice == "e"): f1.truncate(0) f1.close() print("Thank You") break
PLEASE READ!!!! I keep asking the same question and the one answering sending the same.
PLEASE USE FILE METHOD!!
PLEASE READ !!!! .txt file
1. Need to save all the inputted data in .txt file (example reservation.txt)
2. compute the total of adults in generate report
3. compute the total of children in generate report
4. compute the total payment in generate report
5. If DELETE RESERVATION is selected, the user needs to input the reservation number to remove the existing reservation.
6. If the GENERATE REPORT is clicked, display the report of all reservations:
reservation = {}
rnum = 0
while True:
adults = {}
Date = {}
Time = {}
name = {}
Children = {}
total = {}
mylist = []
choice = input(
'\nRESTAURANT RESERVATION SYSTEM\nSystem Menu\nA = View all Reservations\nB = Make Reservation\nC = Delete Reservation\nD = Generate Report\nE = Exit\nEnter choice --> ').lower()
f1 = open("reservation.txt", "a")
if choice in 'Aa':
f2 = open("reservation.txt", "r")
print("Records are:")
print(f"#\t\tDate\tTime\t\tName\t\tTotal Adults\t\tTotal children\t\tSubtotal\t\t")
print(f2.read())
f2.close()
elif choice in 'Bb':
try:
rnum += 1
name = input("Enter Name: ")
Date = input("Enter date(DD-MM-YYYY): ")
Time = input("Enter time(HH:MM): ")
adults = input("Number of adults: ")
Children = input("Number os children: ")
f1.write(str(int(rnum)))
f1.write("\t")
f1.write(Date)
f1.write("\t")
f1.write(Time)
f1.write("\t\t")
f1.write(name)
f1.write("\t\t")
f1.write(adults)
f1.write("\t\t\t\t")
f1.write(Children)
f1.write("\t\t\t\t\t")
total = int(adults) * 500 + int(Children) * 300
reservation[rnum] = [Date, Time, name, adults, Children, total]
f1.write(str(total))
f1.write("\n")
f1.close()
print("\nBelow are your Reservation Details:")
print(
f"#\t{rnum}\nName\t\t{name}\nDate\t\t{Date}\nTime\t\t{Time}\nTotal Adults\t\t{adults}\nTotal children\t\t{Children}\nSubtotal\t\t{total}\n")
print(f1.read())
except ValueError:
print("there is an incorrect value!! try again")
elif choice in 'Cc':
try:
rnumd = int(input("Enter Reservation number:"))
reservation[rnum] = open("reservation.txt", "r+")
reservation[rnum].truncate(rnumd)
print("No Record found")
except KeyError:
print("Invalid reservation ID")
print("Reservation deleted")
elif choice in 'Dd':
f2 = open("reservation.txt", "r+")
values = adults.values()
Atotal = sum(values)
print("Records are:")
print(f"#\t\tDate\t\tTime\t\tDate\t\tTotal Adults\t\tTotal children\t\t")
print(f2.read())
print(f"Total Number of Adults: " + str(Atotal))
print("Total Number of Children: ")
print("Grand Total : ")
f2.close()
elif (choice == "e"):
f1.truncate(0)
f1.close()
print("Thank You")
break

Step by step
Solved in 2 steps with 8 images

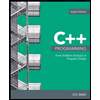
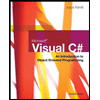
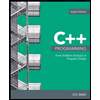
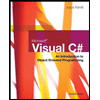