Requirements for the program Finish the print_formatted_tasks(tasks) function. You can assume all fields in all tasks will be valid (all tasks will have all the necessary fields, all "priority" values will be between 1-5, all "deadline" values will be valid date strings, etc. ), so you do not need to do additional validation.
A task in this lab is a dictionary object that is guaranteed to have key-value pairs that follow certain constraints:
-
Every task must have a "name" key, with the value being a string of the task's name
-
Every task must have a "description" field, with the value being a string of the task's description
-
Every task must have a "priority" field, with the value being a number 1-5, representing the task's priority
- 1 stands for "Lowest" (priority)
- 2 stands for "Low" (priority)
- 3 stands for "Medium" (priority)
- 4 stands for "High" (priority)
- 5 stands for "Highest" (priority)
-
Every task must have a "deadline" field, with the value being a task's deadline represented as a valid date-string in slashed-format (ex. "02/05/2022")
Note: we are using the US format for strings: <MM>/<DD>/<YEAR>. 01/02/2022 represents January 2nd, 2022.
-
Every task must have a "completed" field, with the value being a Boolean value representing whether a task is completed or not
Here is an example of what a task could look like:
{ 'name': 'get groceries', 'description': 'buy jam and peanut butter', 'deadline': '02/23/2022', 'priority': 2, 'completed': False }
Printing tasks
To print a task in a human-readable format, make sure that
- The name is written in all caps (i.e., capital letters),
- The priority is converted from an integer to its corresponding string status ("Low", "Lowest", "Medium", "High", "Highest")
- The deadline is converted to its written format (see Lab 7.19)
- The completed field is converted to a "Yes" or "No".
Also, we want to print not just a single task, but a list of tasks, so we will print the index of a task on the same line as its name. We will also print a newline after printing a task to separate them in a list. Here is what the print should look like when a list with just 1 element gets printed:
my_list = [{ 'name': 'get groceries', 'description': 'buy jam and peanut butter', 'priority': 2, 'deadline': '02/23/2022', 'completed': False }] print_formatted_tasks(my_list)
The function call should print:
0: GET GROCERIES Description: buy jam and peanut butter Priority: Low Deadline: February 23, 2022 Completed: No <newline>
Note that <newline> should not be printed but should have a new-line character \n in its place.
Here is what should be produced when the function is called with the following input:
my_list = [{ 'name': 'get groceries', 'description': 'buy jam and peanut butter', 'deadline': '02/23/2022', 'priority': 2, 'completed': False }, { 'name': 'get some sleep', 'description': '8 hours of sleep is necessary', 'deadline': '02/03/2022', 'priority': 3, 'completed': False }, { 'name': 'compar. lit essay', 'description': "finish comparative lit essay that's overdue", 'deadline': '02/15/2022', 'priority': 4, 'completed': True }] print_formatted_tasks(my_list)
The function call should print:
0: GET GROCERIES Description: buy jam and peanut butter Priority: Low Deadline: February 23, 2022 Completed: No 1: GET SOME SLEEP Description: 8 hours of sleep is necessary Priority: Medium Deadline: February 3, 2022 Completed: No 2: COMPAR. LIT ESSAY Description: finish comparative lit essay that's overdue Priority: High Deadline: February 15, 2022 Completed: Yes <newline>
Requirements for the program
- Finish the print_formatted_tasks(tasks) function. You can assume all fields in all tasks will be valid (all tasks will have all the necessary fields, all "priority" values will be between 1-5, all "deadline" values will be valid date strings, etc. ), so you do not need to do additional validation.
Simply print the list of tasks in a user-friendly format defined above.
- Make a couple of dictionaries to convert "completed" from a Boolean to a string, "priority" from an integer to a string.
- Go back to Lab 7.19 and grab the date string conversion function you wrote. Notice that the format for written dates is exactly the same.
def print_formatted_tasks(tasks_list):
# Finish the function definition
if __name__ == "__main__":
# for testing purposes, so you can observe the output
my_list = [{
'name': 'get groceries',
'description': 'buy some jam and peanut butter',
'deadline': '02/23/2022',
'priority': 2,
'completed': False
},
{
'name': 'get some sleep',
'description': '8 hours of sleep is necessary',
'deadline': '02/03/2022',
'priority': 3,
'completed': False
},
{
'name': 'compar. lit essay',
'description': "finish comparative lit essay that's overdue",
'deadline': '02/15/2022',
'priority': 4,
'completed': True
}]

Step by step
Solved in 2 steps

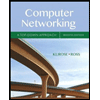
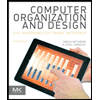
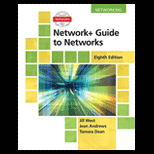
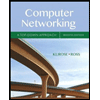
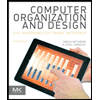
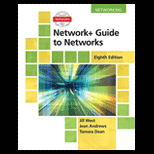
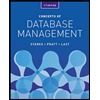
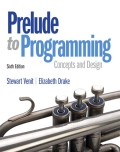
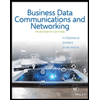