Review Loops Write a java program to ask three people three questions about name, number of courses, and number of credit hours. Also, print result in columns as below.
Review Loops Write a java program to ask three people three questions about name, number of courses, and number of credit hours. Also, print result in columns as below.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
![A loc-cs.org/~chu/DataStructures/H
19
Special Hint for Taking User's Input
import java.util.Scanner;
/**
* This is an example to show you that
* the method nextInt() only flushes the number to the variable
* and leave the "END OF LINE" symbol in the input buffer.
* If you have next input looking for "END OF LINE",
the system will not wait for user's input and
just take the "END OF LINE" symbol from input buffer.
* So, the solution is flush "END OF LINE" out before you
大
* expect an input from user.
* @author Valerie Chu
* @version August 24, 2020
*/
public class Example
{
public static void main(String[] args)
{
Scanner input = new Scanner (System.in);
String [] item = new String[2];
int (] num = new int[2];
for (int i=0; i<2; i++)
{
System.out.println("Which item are you shopping for?");
item[i] =
input.nextLine();
System.out.printf("How many \"%s\" do you need?\n", item[i]);
num[i] = input.nextInt();
input.nextLine();
//Flush the "END OF LINE" symbol out
System.out.printf("%15s%10s\n", "Item", "Quantity");
for (int i=0; i<2; i++)
{
System.out.printf("%15s%10d\n",item[i],num[i]);
You can copy the program below to test it.
import java.util.Scanner;
public class Example
{
public static void main(String[] args)
{
Scanner input = new Scanner(System.in);
String [] item =
int [] num = new int[2];
for(int i=0; i<2; i++)
{
System.out.println("Which item are you shopping for?");
item[i]
System.out.printf("How many \"%s\" do you need?\n", item[i]);
num[i] =
input.nextLine();
}
System.out.printf("%15s%10s\n", "Item", "Quantity");
for (int i=0; i<2; i++)
{
System.out.printf("%15s%1od\n",item[i], num[i]);
new String[2];
input.nextLine();
input.nextInt();
//Flush the "END OF LINE" symbol out
}
}
}](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fdc7af1f5-b041-422e-a7e0-fe3bd054e9c0%2Fa45d8c0e-b4bd-4246-b038-687959a17e47%2Fbash8v_processed.png&w=3840&q=75)
Transcribed Image Text:A loc-cs.org/~chu/DataStructures/H
19
Special Hint for Taking User's Input
import java.util.Scanner;
/**
* This is an example to show you that
* the method nextInt() only flushes the number to the variable
* and leave the "END OF LINE" symbol in the input buffer.
* If you have next input looking for "END OF LINE",
the system will not wait for user's input and
just take the "END OF LINE" symbol from input buffer.
* So, the solution is flush "END OF LINE" out before you
大
* expect an input from user.
* @author Valerie Chu
* @version August 24, 2020
*/
public class Example
{
public static void main(String[] args)
{
Scanner input = new Scanner (System.in);
String [] item = new String[2];
int (] num = new int[2];
for (int i=0; i<2; i++)
{
System.out.println("Which item are you shopping for?");
item[i] =
input.nextLine();
System.out.printf("How many \"%s\" do you need?\n", item[i]);
num[i] = input.nextInt();
input.nextLine();
//Flush the "END OF LINE" symbol out
System.out.printf("%15s%10s\n", "Item", "Quantity");
for (int i=0; i<2; i++)
{
System.out.printf("%15s%10d\n",item[i],num[i]);
You can copy the program below to test it.
import java.util.Scanner;
public class Example
{
public static void main(String[] args)
{
Scanner input = new Scanner(System.in);
String [] item =
int [] num = new int[2];
for(int i=0; i<2; i++)
{
System.out.println("Which item are you shopping for?");
item[i]
System.out.printf("How many \"%s\" do you need?\n", item[i]);
num[i] =
input.nextLine();
}
System.out.printf("%15s%10s\n", "Item", "Quantity");
for (int i=0; i<2; i++)
{
System.out.printf("%15s%1od\n",item[i], num[i]);
new String[2];
input.nextLine();
input.nextInt();
//Flush the "END OF LINE" symbol out
}
}
}
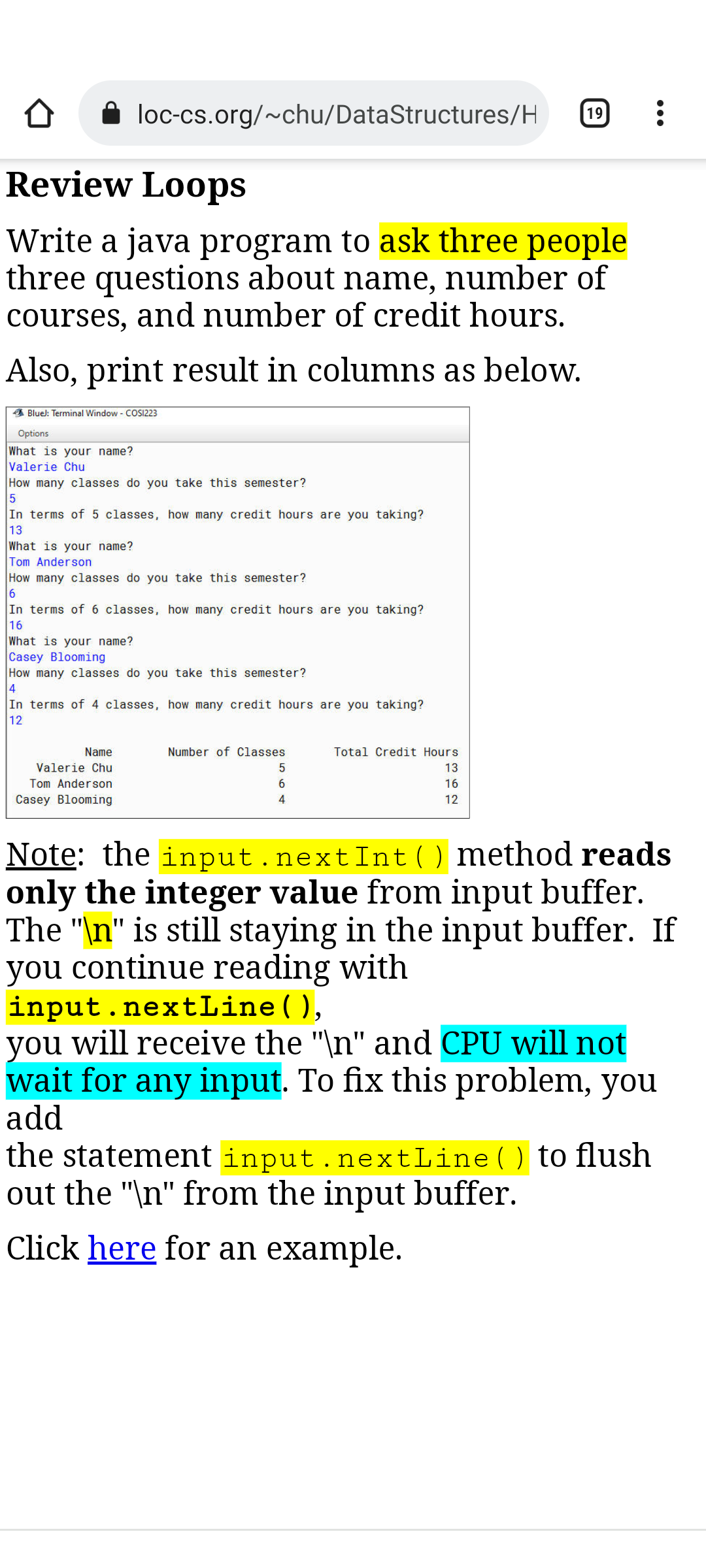
Transcribed Image Text:A loc-cs.org/~chu/DataStructures/H
19
Review Loops
Write a java program to ask three people
three questions about name, number of
courses, and number of credit hours.
Also, print result in columns as below.
A Bluel: Terminal Window - COSI223
Options
What is your name?
Valerie Chu
How many classes do you take this semester?
In terms of 5 classes, how many credit hours are you taking?
13
What is your name?
Tom Anderson
How many classes do you take this semester?
6.
In terms of 6 classes, how many credit hours are you taking?
16
What is your name?
Casey Blooming
How many classes do you take this semester?
4
In terms of 4 classes, how many credit hours are you taking?
12
Name
Number of Classes
Total Credit Hours
Valerie Chu
5
13
Tom Anderson
Casey Blooming
16
4
12
Note: the input.nextInt() method reads
only the integer value from input buffer.
The "\n" is still staying in the input buffer. If
you continue reading with
input.nextLine(),
you will receive the "\n" and CPU will not
wait for any input. To fix this problem, you
add
the statement input.nextLine() to flush
out the "\n" from the input buffer.
Click here for an example.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Recommended textbooks for you
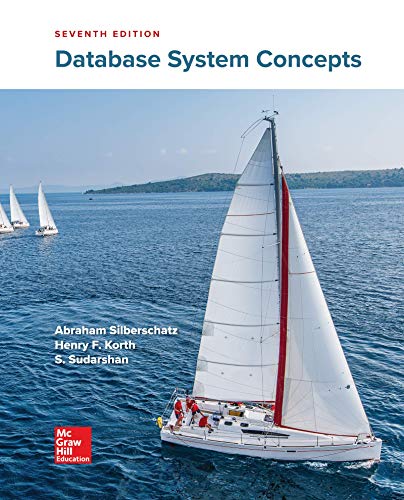
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
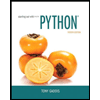
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
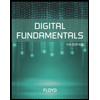
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
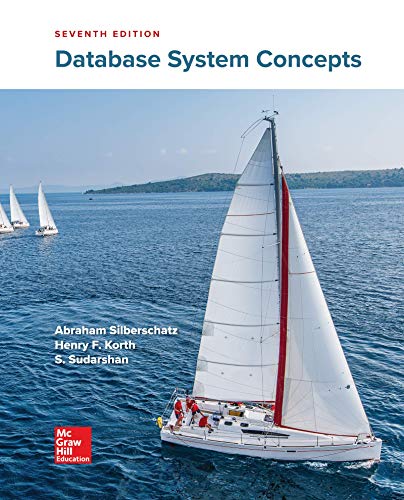
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
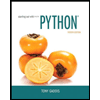
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
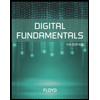
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
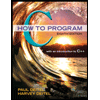
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
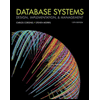
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
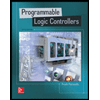
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education