Java Program ASAP I want only one program. Please pay attention to the screenshot for test case 2 and 3 and please make sure you match the result. Modify this program so it passes the test cases in Hypergrade becauses it says 5 out of 7 passed. import java.io.BufferedReader; import java.io.FileReader; import java.io.IOException; import java.util.ArrayList; import java.util.Arrays; import java.util.InputMismatchException; import java.util.Scanner; public class FileSorting { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); while (true) { System.out.println("Please enter the file name or type QUIT to exit:"); String fileName = scanner.nextLine(); if (fileName.equalsIgnoreCase("QUIT")) { break; } try { ArrayList lines = readFile(fileName); if (lines.isEmpty()) { System.out.println("File " + fileName + " is empty."); } else { performSorting(lines); } } catch (IOException e) { System.out.println("File " + fileName + " is not found."); if (!fileName.equalsIgnoreCase("QUIT")) { System.out.println("Please re-enter the file name or type QUIT to exit:"); } } } scanner.close(); } private static ArrayList readFile(String fileName) throws IOException { ArrayList lines = new ArrayList<>(); try (BufferedReader reader = new BufferedReader(new FileReader(fileName))) { String line; while ((line = reader.readLine()) != null) { lines.add(line); } } return lines; } private static void performSorting(ArrayList lines) { try { ArrayList> numbers = new ArrayList<>(); for (String line : lines) { String[] elements = line.split(","); ArrayList row = new ArrayList<>(); for (String element : elements) { row.add(Integer.parseInt(element)); } numbers.add(row); } for (ArrayList row : numbers) { Integer[] array = row.toArray(new Integer[0]); Arrays.sort(array); // Use Arrays.sort() for sorting System.out.println(Arrays.toString(array).replaceAll("[\\[\\],]", "")); } } catch (NumberFormatException | InputMismatchException e) { System.out.println("Invalid file format."); } } } input2x2.csv -67,-11 -27,-70 input1.csv 10 input10x10.csv 56,-19,-21,-51,45,96,-46 -27,29,-58,85,8,71,34 50,51,40,50,100,-82,-87 -47,-24,-27,-32,-25,46,88 -47,95,-41,-75,85,-16,43 -78,0,94,-77,-69,78,-25 -80,-31,-95,82,-86,-32,-22 68,-52,-4,-68,10,-14,-89 26,33,-59,-51,-48,-34,-52 -47,-24,80,16,80,-66,-42 input0.csv Test Case 1 Please enter the file name or type QUIT to exit:\n input1.csvENTER 10\n Test Case 2 Please enter the file name or type QUIT to exit:\n input2x2.csvENTER -67,-11\n -70,-27\n Test Case 3 Please enter the file name or type QUIT to exit:\n input10x10.csvENTER -51,-46,-21,-19,45,56,96\n -58,-27,8,29,34,71,85\n -87,-82,40,50,50,51,100\n -47,-32,-27,-25,-24,46,88\n -75,-47,-41,-16,43,85,95\n -78,-77,-69,-25,0,78,94\n -95,-86,-80,-32,-31,-22,82\n -89,-68,-52,-14,-4,10,68\n -59,-52,-51,-48,-34,26,33\n -66,-47,-42,-24,16,80,80\n Test Case 4 Please enter the file name or type QUIT to exit:\n input0.csvENTER File input0.csv is empty.\n Test Case 5 Please enter the file name or type QUIT to exit:\n input2.csvENTER File input2.csv is not found.\n Please re-enter the file name or type QUIT to exit:\n input1.csvENTER 10\n Test Case 6 Please enter the file name or type QUIT to exit:\n
Java
I want only one program. Please pay attention to the screenshot for test case 2 and 3 and please make sure you match the result. Modify this program so it passes the test cases in Hypergrade becauses it says 5 out of 7 passed.
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.InputMismatchException;
import java.util.Scanner;
public class FileSorting {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.println("Please enter the file name or type QUIT to exit:");
String fileName = scanner.nextLine();
if (fileName.equalsIgnoreCase("QUIT")) {
break;
}
try {
ArrayList<String> lines = readFile(fileName);
if (lines.isEmpty()) {
System.out.println("File " + fileName + " is empty.");
} else {
performSorting(lines);
}
} catch (IOException e) {
System.out.println("File " + fileName + " is not found.");
if (!fileName.equalsIgnoreCase("QUIT")) {
System.out.println("Please re-enter the file name or type QUIT to exit:");
}
}
}
scanner.close();
}
private static ArrayList<String> readFile(String fileName) throws IOException {
ArrayList<String> lines = new ArrayList<>();
try (BufferedReader reader = new BufferedReader(new FileReader(fileName))) {
String line;
while ((line = reader.readLine()) != null) {
lines.add(line);
}
}
return lines;
}
private static void performSorting(ArrayList<String> lines) {
try {
ArrayList<ArrayList<Integer>> numbers = new ArrayList<>();
for (String line : lines) {
String[] elements = line.split(",");
ArrayList<Integer> row = new ArrayList<>();
for (String element : elements) {
row.add(Integer.parseInt(element));
}
numbers.add(row);
}
for (ArrayList<Integer> row : numbers) {
Integer[] array = row.toArray(new Integer[0]);
Arrays.sort(array); // Use Arrays.sort() for sorting
System.out.println(Arrays.toString(array).replaceAll("[\\[\\],]", ""));
}
} catch (NumberFormatException | InputMismatchException e) {
System.out.println("Invalid file format.");
}
}
}
input2x2.csv
-67,-11
-27,-70
input1.csv
10
input10x10.csv
56,-19,-21,-51,45,96,-46
-27,29,-58,85,8,71,34
50,51,40,50,100,-82,-87
-47,-24,-27,-32,-25,46,88
-47,95,-41,-75,85,-16,43
-78,0,94,-77,-69,78,-25
-80,-31,-95,82,-86,-32,-22
68,-52,-4,-68,10,-14,-89
26,33,-59,-51,-48,-34,-52
-47,-24,80,16,80,-66,-42
input0.csv
Test Case 1
input1.csvENTER
10\n
Test Case 2
input2x2.csvENTER
-67,-11\n
-70,-27\n
Test Case 3
input10x10.csvENTER
-51,-46,-21,-19,45,56,96\n
-58,-27,8,29,34,71,85\n
-87,-82,40,50,50,51,100\n
-47,-32,-27,-25,-24,46,88\n
-75,-47,-41,-16,43,85,95\n
-78,-77,-69,-25,0,78,94\n
-95,-86,-80,-32,-31,-22,82\n
-89,-68,-52,-14,-4,10,68\n
-59,-52,-51,-48,-34,26,33\n
-66,-47,-42,-24,16,80,80\n
Test Case 4
input0.csvENTER
File input0.csv is empty.\n
Test Case 5
input2.csvENTER
File input2.csv is not found.\n
Please re-enter the file name or type QUIT to exit:\n
input1.csvENTER
10\n
Test Case 6
quitENTER
Test Case 7
input2.csvENTER
File input2.csv is not found.\n
Please re-enter the file name or type QUIT to exit:\n
QUITENTER
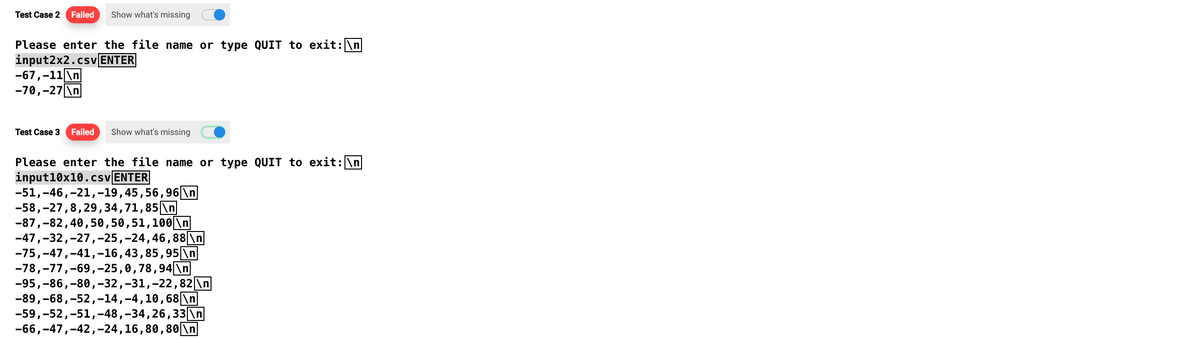
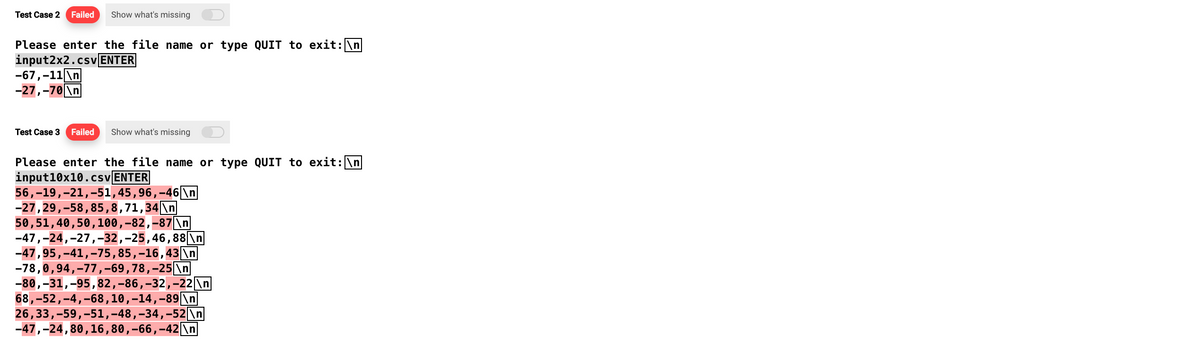

Step by step
Solved in 3 steps with 1 images

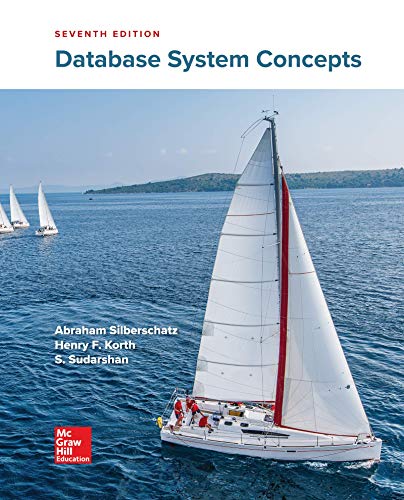
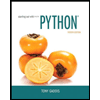
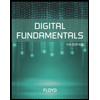
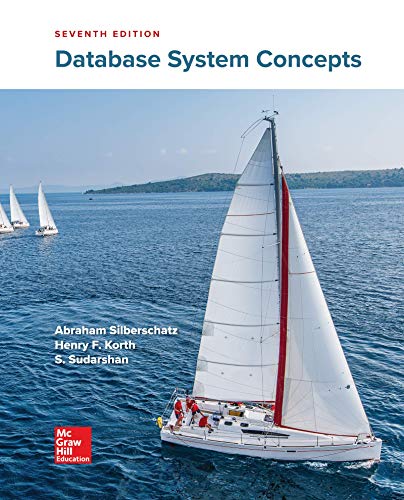
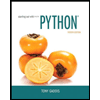
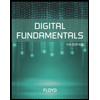
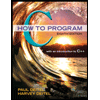
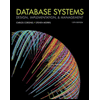
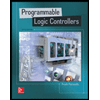