Revise a MarketPlace class in PYTHON that allows users to buy/sell stocks. It will match buyers with the lowest seller (<= buyer's price) and match sellers with the highest buyer (>= seller's price). Given this current class, the follow up is that input now has expiration dates included in the buy/sell order methods. Revise the class to include handling expiration, and removing from current heaps. Example: (109,Time1), (110,Time2), (110,Time3), (114,Time4) Hint: change method parameters to accept a currentTime and expirationTime and heaps to consist of a dictionary where the key is the buying/selling price and the value is the currentTime + expirationTime. So when you check for a match the map.key has to match price wise and map.val has to be larger than the current time otherwise the offer is expired.
Revise a MarketPlace class in PYTHON that allows users to buy/sell stocks. It will match buyers with the lowest seller (<= buyer's price) and match sellers with the highest buyer (>= seller's price).
Given this current class, the follow up is that input now has expiration dates included in the buy/sell order methods. Revise the class to include handling expiration, and removing from current heaps. Example: (109,Time1), (110,Time2), (110,Time3), (114,Time4)
Hint: change method parameters to accept a currentTime and expirationTime and heaps to consist of a dictionary where the key is the buying/selling price and the value is the currentTime + expirationTime. So when you check for a match the map.key has to match price wise and map.val has to be larger than the current time otherwise the offer is expired.
class Marketplace:
def __init__(self, buy_offers, sell_offers):
self.buy_offers = [-offer for offer in buy_offers]
heapq.heapify(self.buy_offers)
# Maintain min heap for sell offers so self.sell_offers[0] returns smallest sell offer
self.sell_offers = sell_offers
heapq.heapify(self.sell_offers)
def match_new_buy_offer(self, amount):
if len(self.sell_offers) == 0 or self.sell_offers[0] > amount:
print(f'No match for buy offer at ${amount}')
heapq.heappush(self.buy_offers, -amount)
matched_offer = heapq.heappop(self.sell_offers)
print(f'Matched buy offer at ${amount} to existing sell offer for ${matched_offer}')
def match_new_sell_offer(self, amount):
if len(self.buy_offers) == 0 or -self.buy_offers[0] < amount:
print(f'No match for sell offer at ${amount}')
heapq.heappush(self.sell_offers, amount)
matched_offer = -heapq.heappop(self.buy_offers)
print(f'Matched sell offer at ${amount} to existing buy offer for ${matched_offer}')
Example input:
marketplace = Marketplace(buy_offers=[90, 99, 99, 100, 100], sell_offers=[110, 110, 120]) marketplace.match_new_sell_offer(95) # matches to $100
marketplace.match_new_buy_offer(150) # matches to $110
marketplace.match_new_buy_offer(109) # no match, added to heap marketplace.match_new_sell_offer(100) # matches to $109
Follow up inputs:
marketplace.match_new_buy_offer(price,expiration_time)
marketplace.match_new_sell_offer(price,expiration_time)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

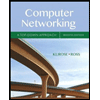
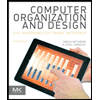
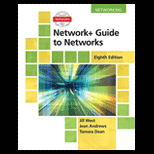
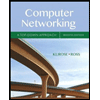
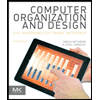
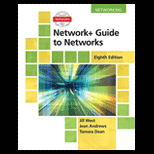
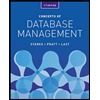
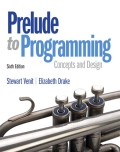
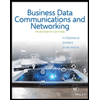