Separate the server code and client code into two different programs, server.py and client.py. Execute the server program first and then execute the client program. You should still get the same set of print messages as in the combined threaded code in proj.py In the program provided, the server just sends a message string to the client after it connects. In this step you have to modify the server in two cases. 1) Modify the server code so that when the client sends a string to the server, the server reverses the string before sending it back to the client. For example, if the client sends HELLO to the server, the client should receive OLLEH. Your client program should print the string sent by the client and the corresponding string received by the client from the server. 2) Modify the server code so that when the client sends a string to the server, the server change all letters to upper case letters and sending it back to the client. For example, if the client sends hello to the server, the client should receive HELLO. Your client program should print the string sent by the client and the corresponding string received by the client from the server. Now make your client program read each line from an input file in-proj.txt and send it to the server. Your server program should print the output of each cases to a file. A sample input file is provided. You are welcome to modify this file and try with your own sample inputs. If it helps, you may assume each line will be at most 200 characters long. The input file may contain multiple lines. Each input line may contain any character that can be typed on a standard US qwerty keyboard, including space. Python Code import threading import time import random import socket def server(): try: ss = socket.socket(socket.AF_INET, socket.SOCK_STREAM) print("[S]: Server socket created") except socket.error as err: print('socket open error: {}\n'.format(err)) exit() server_binding = ('', 50007) ss.bind(server_binding) ss.listen(1) host = socket.gethostname() print("[S]: Server host name is {}".format(host)) localhost_ip = (socket.gethostbyname(host)) print("[S]: Server IP address is {}".format(localhost_ip)) csockid, addr = ss.accept() print ("[S]: Got a connection request from a client at {}".format(addr)) # send a intro message to the client. msg = "Welcome!" csockid.send(msg.encode('utf-8')) # Close the server socket ss.close() exit() def client(): try: cs = socket.socket(socket.AF_INET, socket.SOCK_STREAM) print("[C]: Client socket created") except socket.error as err: print('socket open error: {} \n'.format(err)) exit() # Define the port on which you want to connect to the server port = 50007 localhost_addr = socket.gethostbyname(socket.gethostname()) # connect to the server on local machine server_binding = (localhost_addr, port) cs.connect(server_binding) # Receive data from the server data_from_server=cs.recv(100) print("[C]: Data received from server: {}".format(data_from_server.decode('utf-8'))) # close the client socket cs.close() exit() if __name__ == "__main__": t1 = threading.Thread(name='server', target=server) t1.start() t2 = threading.Thread(name='client', target=client) t2.start() print("Done.")
Separate the server code and client code into two different programs, server.py and client.py. Execute the server program first and then execute the client program. You should still get the same set of print messages as in the combined threaded code in proj.py
In the program provided, the server just sends a message string to the client after it connects. In this step you have to modify the server in two cases.
1) Modify the server code so that when the client sends a string to the server, the server reverses the string before sending it back to the client. For example, if the client sends HELLO to the server, the client should receive OLLEH. Your client program should print the string sent by the client and the corresponding string received by the client from the server.
2) Modify the server code so that when the client sends a string to the server, the server change all letters to upper case letters and sending it back to the client. For example, if the client sends hello to the server, the client should receive HELLO. Your client program should print the string sent by the client and the corresponding string received by the client from the server.
Now make your client program read each line from an input file in-proj.txt and send it to the server. Your server program should print the output of each cases to a file. A sample input file is provided. You are welcome to modify this file and try with your own sample inputs. If it helps, you may assume each line will be at most 200 characters long. The input file may contain multiple lines. Each input line may contain any character that can be typed on a standard US qwerty keyboard, including space.
Python Code
import threading
import time
import random
import socket
def server():
try:
ss = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
print("[S]: Server socket created")
except socket.error as err:
print('socket open error: {}\n'.format(err))
exit()
server_binding = ('', 50007)
ss.bind(server_binding)
ss.listen(1)
host = socket.gethostname()
print("[S]: Server host name is {}".format(host))
localhost_ip = (socket.gethostbyname(host))
print("[S]: Server IP address is {}".format(localhost_ip))
csockid, addr = ss.accept()
print ("[S]: Got a connection request from a client at {}".format(addr))
# send a intro message to the client.
msg = "Welcome!"
csockid.send(msg.encode('utf-8'))
# Close the server socket
ss.close()
exit()
def client():
try:
cs = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
print("[C]: Client socket created")
except socket.error as err:
print('socket open error: {} \n'.format(err))
exit()
# Define the port on which you want to connect to the server
port = 50007
localhost_addr = socket.gethostbyname(socket.gethostname())
# connect to the server on local machine
server_binding = (localhost_addr, port)
cs.connect(server_binding)
# Receive data from the server
data_from_server=cs.recv(100)
print("[C]: Data received from server: {}".format(data_from_server.decode('utf-8')))
# close the client socket
cs.close()
exit()
if __name__ == "__main__":
t1 = threading.Thread(name='server', target=server)
t1.start()
t2 = threading.Thread(name='client', target=client)
t2.start()
print("Done.")

Step by step
Solved in 4 steps with 4 images

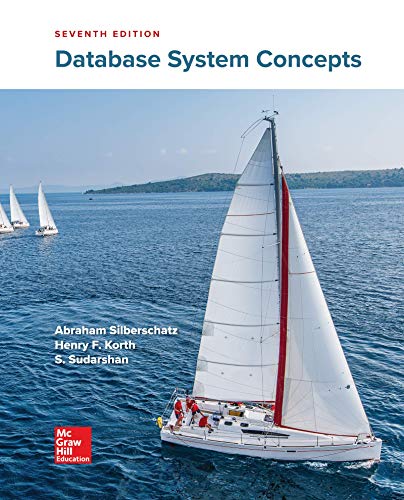
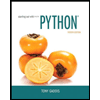
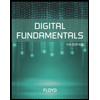
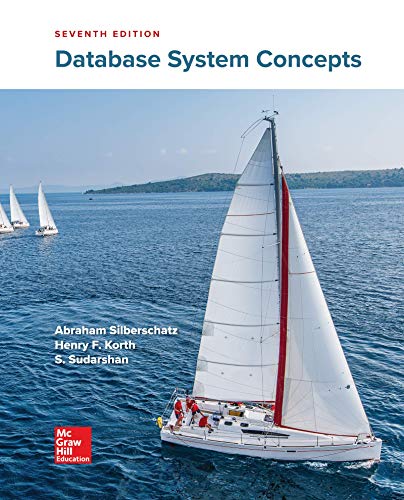
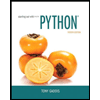
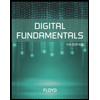
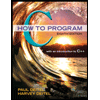
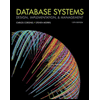
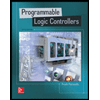