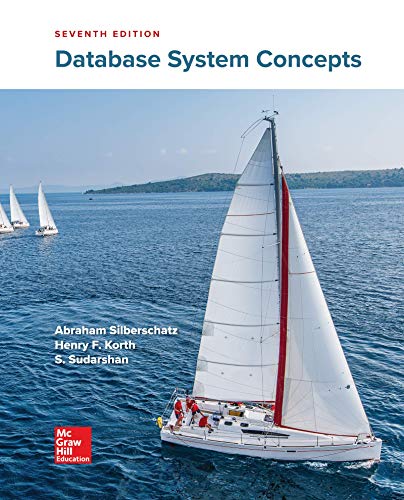
Write a client program for MyTime which loops around getting strings from
the user representing times and responding with a greeting such as “good
morning” as appropriate. The user should enter the string “quit” to exit the
program.
EXAMPLE run of client:
USER: 2:07 pm
PROGRAM: good afternoon
USER: 10:71 am
PROGRAM: that’s not a valid time, please re-enter
USER: 14:30am
PROGRAM: that’s not a valid time, please re-enter
USER: 10:21am
PROGRAM: good morning
USER: 7:00 pm
PROGRAM: good evening
the set method for MyTime should detect input
strings which do not represent valid times. An exception should be thrown in
such a case. Clients will have
to handle (or catch) the exceptions. Thus you will have to also write your own exception class which can be used by MyTime and the client class.
Here are my code:
public class MyTime {
private String time;
public String getTime() {
return time;
}
public void setTime() throws InvalidTime {
String time = askTime();
if (time.equalsIgnoreCase("quit")) {
System.exit(0);
} else {
try {
getHours(time);
getMinutes(time);
AmOrPM(time);
greetUser(time);
} catch (InvalidTime e) {
System.out.println("that's not a valid time, please re-enter");
setTime();
}
}
}
public static int getHours(String time) throws InvalidTime {
String[] separatedHourAndMin = time.split(":");
int hour = Integer.parseInt(separatedHourAndMin[0]);
if (hour >= 0 && hour <= 12) {
return hour;
} else {
throw new InvalidTime("that's not a valid time, please re-enter");
}
}
public static int getMinutes(String time) {
MyTime mt = new MyTime();
String[] separatedHourAndMin = time.split(":");
String[] separatedMinandAM_PM = separatedHourAndMin[1].split(" ");
int min = Integer.parseInt(separatedMinandAM_PM[0]);
if (min >= 0 && min <= 60) {
return min;
} else {
System.out.println("that's not a valid time, please re-enter");
try {
mt.setTime();
} catch (InvalidTime e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
return min;
}
public static boolean isAm(String time) throws InvalidTime {
String[] separatedHourAndMin = time.split(":");
String[] separatedMinandAM_PM = separatedHourAndMin[1].split(" ");
if (separatedMinandAM_PM[1].equalsIgnoreCase("am")) {
return true;
} else {
throw new InvalidTime("that's not a valid time, please re-enter");
}
}
public String AmOrPM(String time) throws InvalidTime {
String[] separatedHourAndMin = time.split(":");
String[] separatedMinandAM_PM = separatedHourAndMin[1].split(" ");
if (separatedMinandAM_PM[1].equalsIgnoreCase("am")) {
return "am";
} else {
if (separatedMinandAM_PM[1].equalsIgnoreCase("pm")) {
return "pm";
} else {
throw new InvalidTime("that's not a valid time, please re-enter");
}
}
}
public void greetUser(String timeAmOrPM) throws InvalidTime {
MyTime mt = new MyTime();
if (getHours(timeAmOrPM) >= 6 && getHours(timeAmOrPM) < 12 && AmOrPM(timeAmOrPM).equalsIgnoreCase("pm")) {
System.out.println("good evening");
mt.setTime();
;
} else {
if (getHours(timeAmOrPM) >= 0 && getHours(timeAmOrPM) < 3 && AmOrPM(timeAmOrPM).equalsIgnoreCase("am")) {
System.out.println("good night");
mt.setTime();
} else {
if (getHours(timeAmOrPM) >= 3 && getHours(timeAmOrPM) < 11
&& AmOrPM(timeAmOrPM).equalsIgnoreCase("am")) {
System.out.println("good morning");
mt.setTime();
;
} else {
if ((getHours(timeAmOrPM) >= 12 || getHours(timeAmOrPM) < 6)
&& AmOrPM(timeAmOrPM).equalsIgnoreCase("pm")) {
System.out.println("good afternoon");
mt.setTime();
} else {
System.out.println("that's not a valid time, please re-enter");
mt.setTime();
}
}
}
}
}
public static String askTime() {
Scanner sc = new Scanner(System.in);
System.out.println("Enter time: ");
return sc.nextLine();
}
}
public class InvalidTime extends Exception{
InvalidTime(String s){
super(s);
}
}

Step by stepSolved in 4 steps with 3 images

- Write a program called p3.py. Your program should have the following functionality: 1. Randomly selects a word from a pre-set list: kangaroo, capybara, wombat, koala, wallaby, quokka, platypus, dingo, kookaburra 2. Prompts the user to guess a letter 3. Validates the input 4. If the letter is not in the word, print a message reflecting this 5. Prints the word where all guessed letters are revealed and all unguessed letters are replaced by underscores hint : consider storing the guessed letters in a list 6. The program should continuously prompt the user to guess letters until they enter ‘quit’ or all the letters in the word have been guessed 7. Don’t forget to include the main guard Example of output: The program selects the word quokka, but the user does not know Guess a letter: a _____a Guess a letter: e there is no ‘e’ _____a Guess a letter: k ___kka Guess a letter: u _u_kka Guess a letter: o _uokka Guess a letter: q quokka You win! Good job!arrow_forwardIn Java Suppose you are given a text file that contains the names of people. Every name inthe file consists of a first name and a last name. Unfortunately, the programmer thatcreated the file of names had a strange sense of humor and did not guarantee thateach name was on a single line of the file. Write a program that reads this file ofnames and writes them to the console, one name per line.For example, if the input file (Names.txt) contains:Bob Jones FredCharles EdMarstonJeffWilliamsThe output should be:Bob JonesFred CharlesEd MarstonJeff Williams Sample Output:Enter the name of the input file:Names.txtBob JonesFred CharlesEd MarstonJeff WilliamsFile processing completed.arrow_forwardWrite a Java program correctly that generates a report of proof of vaccination against COVID-19 for the peoplewho received their dose of vaccination. Write a program which:1. Display a welcome message.2. Then prompts the user to enter the following:• First name, with first letter uppercase, which is then stores in a variable of type String.• Last name, with first letter uppercase which is then stores in a variable of type String.• Day of birth, set as a number between 1 and 31, which is then stores in a variable of typeint.• Month of birth, set as a number between 1 and 12, which is then stores in a variable of typeint.• Year of birth, set as a number between 1920 and 2006, which is then stores in a variable oftype int.• Day of vaccination, set as a number between 1 and 31, which is then stores in a variable oftype int.• Month of vaccination. set as a number between 1 and 12, which is then stores in a variableof type int.• Year of vaccination, set as a number between 2020 and 2021,…arrow_forward
- A private hospital would like to implement a Java application to manage patient-specialist appointment. The hospital has five specialists, in different areas of medicine (e.g. cardiac, renal, paediatric). These specialists have regular slots for appointments every week. For example, the cardiac specialist may have six slots available on Monday, Wednesday and Friday; for simplicity, we assume each slot starts on the hour (e.g. 9am, 10am etc).The system should be used by patients to schedule an appointment with a specialist. Each patient will first enter their unique hospital id number, and then the specialist they want to meet and desired date. The system will list the available slots for that specialist. The patient may then select one of the availableslots, select a different date, or a different specialist. The patient should also be shown a list of all booked appointments, which they have yet to attend. When the patient arrives for their appointment, they need to enter their…arrow_forward. You have a shopping list of some items. You want to substitute one of the items with some other items. Write a script that does that. For example: Your old shopping list is: ['apple', 'pear', 'orange', 'grape'] Enter 3 new items: banana peach kiwi Enter the item to be replaced: orange New list: ['apple', 'pear', ['banana', 'peach', 'kiwi'], 'grape']arrow_forwardWrite a Java program that opens a file and counts the whitespace-separated words in that file. You may obtain the name of the input file either from the command line or via prompt and user input. Be sure to clearly document the method chosen. You may test your program using any number of text files; however, you must be sure to test your program using the Sample Text File provided at the end (excerpt.txt). Sample Text File There was nothing so VERY remarkable in that; nor did Alice think it so VERY much out of the way to hear the Rabbit say to itself, `Oh dear! Oh dear! I shall be late!' (when she thought it over afterwards, it occurred to her that she ought to have wondered at this, but at the time it all seemed quite natural); but when the Rabbit actually TOOK A WATCH OUT OF ITS WAISTCOAT- POCKET, and looked at it, and then hurried on, Alice started to her feet, for it flashed across her mind that she had never before seen a rabbit with either a waistcoat-pocket, or a watch to…arrow_forward
- Write a unit conversion program that asks the users from which unit they want to convert(gal, oz, lb, ft, mi) and to which unit they want to convert (l, g, kg, m, km). Rejectincompatible conversions (such as gal → km). Ask for the value to be converted, thendisplay the result:Convert from? galConvert to? mlValue? 2.52.5 gal = 9462.5 mlarrow_forwardWrite a program that reads a string (password) and a number. The maximum number of attempts (3). The program with stop asking data if the word ‘quit’ is provided in the password value. If user provide correct credential the message access granted will be displayed otherwise the message access denied with be generated.arrow_forwardIn Java for zybooks ,Lab 3.27, Write a program that creates a login name for a user, given the user's first name, last name, and a four-digit integer as input. Output the login name, which is made up of the first six letters of the first name, followed by the first letter of the last name, an underscore (_), and then the last digit of the number (use the % operator). If the first name has less than six letters, then use all letters of the first name.arrow_forward
- Write a program that creates a login name for a user, given the user's first name, last name, and a four-digit integer as input. Output the login name, which is made up of the first five letters of the last name, followed by the first letter of the first name, and then the last two digits of the number (use the % operator). If the last name has less than five letters, then use all letters of the last name. Hint: Use the to_string() function to convert numerical data to a string.arrow_forwardWrite a program that prompts a user to enter a year between 1 and 2499 and prints its century in the format such as 1st, 2nd, 3rd, 4th, etc. please use a switch statement. for what we have done in class so far we have only used #include <iostream> <fstream> <cmath> <string> <iomanip>, so if possible if this can be done using that so I can understand for my next assignment that would be great thanks! Test Run 1:Enter a year between 1 and 2499 -- 19231923 is in the 20th century Test Run 2:Enter a year between 1 and 2499 -- 22022202 is in the 23rd century Test Run 3:Enter a year between 1 and 2499 -- 2630Wrong year!arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
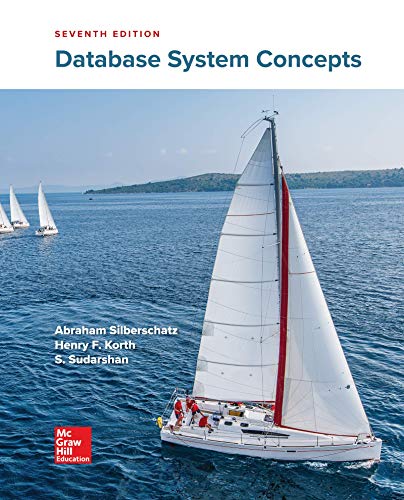
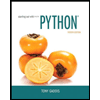
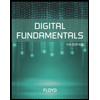
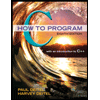
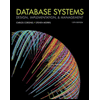
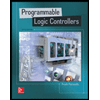