SHOW ALL YOUR WORK. REMEMBER THAT PROGRAM SEGMENTS ARE TO BE WRITTEN IN JAVA. Assume that the classes listed in the Java Quick Reference have been imported where appropriate. . Unless otherwise noted in the question, assume that parameters in method calls are not null and that methods are called only when their preconditions are satisfied. • In writing solutions for each question, you may use any of the accessible methods that are listed in classes defined in that question. Writing significant amounts of code that can be replaced by a call to one of these methods will not receive full credit. A manufacturer wants to keep track of the average of the ratings that have been submitted for an item using a running average. The algorithm for calculating a running average differs from the standard algorithm for calculating an average, as described in part (a). A partial declaration of the RunningAverage class is shown below. You will write two methods of the RunningAverage class. public class RunningAverage { /** The number of ratings included in the running average. */ private int count; /** The average of the ratings that have been entered. */ private double average; // There are no other instance variables. /** Creates a RunningAverage object. * Postcondition: count is initialized to è and average is initialized to 0.0. public RunningAverage() { / implementation not shown */ } /** Updates the running average to reflect the entry of a new rating, as described in part (a). public void updateAverage (double newal) { /* to be implemented in part (a) / ) /** Processes nun new ratings by considering them for inclusion in the running average and updating the running average as necessary. Returns an integer that represents the number of • invalid ratings, as described in part (b). • Precondition: num > e public int processNewRatings(int nun) { /* to be implemented in part (b) */ } /** Returns a single numeric rating. / public double getNewRating() { /* implementation not shown */ } } (a) Write the method updateAverage, which updates the RunningAverage object to include a new rating. To update a running average, add the new rating to a calculated total, which is the number of ratings times the current running average. Divide the new total by the incremented count to obtain the new running average. For example, if there are 4 ratings with a current running average of 3.5, the calculated total is 4 times 3.5. or 14.0. When a fifth rating with a value of 6.0 is included, the new total becomes 20.0. The new running average is 20.0 divided by 5, or 4.0. Complete method updateAverage. /** Updates the running average to reflect the entry of a new rating, as described in part (a). " public void updateAverage (double newal)
SHOW ALL YOUR WORK. REMEMBER THAT PROGRAM SEGMENTS ARE TO BE WRITTEN IN JAVA. Assume that the classes listed in the Java Quick Reference have been imported where appropriate. . Unless otherwise noted in the question, assume that parameters in method calls are not null and that methods are called only when their preconditions are satisfied. • In writing solutions for each question, you may use any of the accessible methods that are listed in classes defined in that question. Writing significant amounts of code that can be replaced by a call to one of these methods will not receive full credit. A manufacturer wants to keep track of the average of the ratings that have been submitted for an item using a running average. The algorithm for calculating a running average differs from the standard algorithm for calculating an average, as described in part (a). A partial declaration of the RunningAverage class is shown below. You will write two methods of the RunningAverage class. public class RunningAverage { /** The number of ratings included in the running average. */ private int count; /** The average of the ratings that have been entered. */ private double average; // There are no other instance variables. /** Creates a RunningAverage object. * Postcondition: count is initialized to è and average is initialized to 0.0. public RunningAverage() { / implementation not shown */ } /** Updates the running average to reflect the entry of a new rating, as described in part (a). public void updateAverage (double newal) { /* to be implemented in part (a) / ) /** Processes nun new ratings by considering them for inclusion in the running average and updating the running average as necessary. Returns an integer that represents the number of • invalid ratings, as described in part (b). • Precondition: num > e public int processNewRatings(int nun) { /* to be implemented in part (b) */ } /** Returns a single numeric rating. / public double getNewRating() { /* implementation not shown */ } } (a) Write the method updateAverage, which updates the RunningAverage object to include a new rating. To update a running average, add the new rating to a calculated total, which is the number of ratings times the current running average. Divide the new total by the incremented count to obtain the new running average. For example, if there are 4 ratings with a current running average of 3.5, the calculated total is 4 times 3.5. or 14.0. When a fifth rating with a value of 6.0 is included, the new total becomes 20.0. The new running average is 20.0 divided by 5, or 4.0. Complete method updateAverage. /** Updates the running average to reflect the entry of a new rating, as described in part (a). " public void updateAverage (double newal)
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
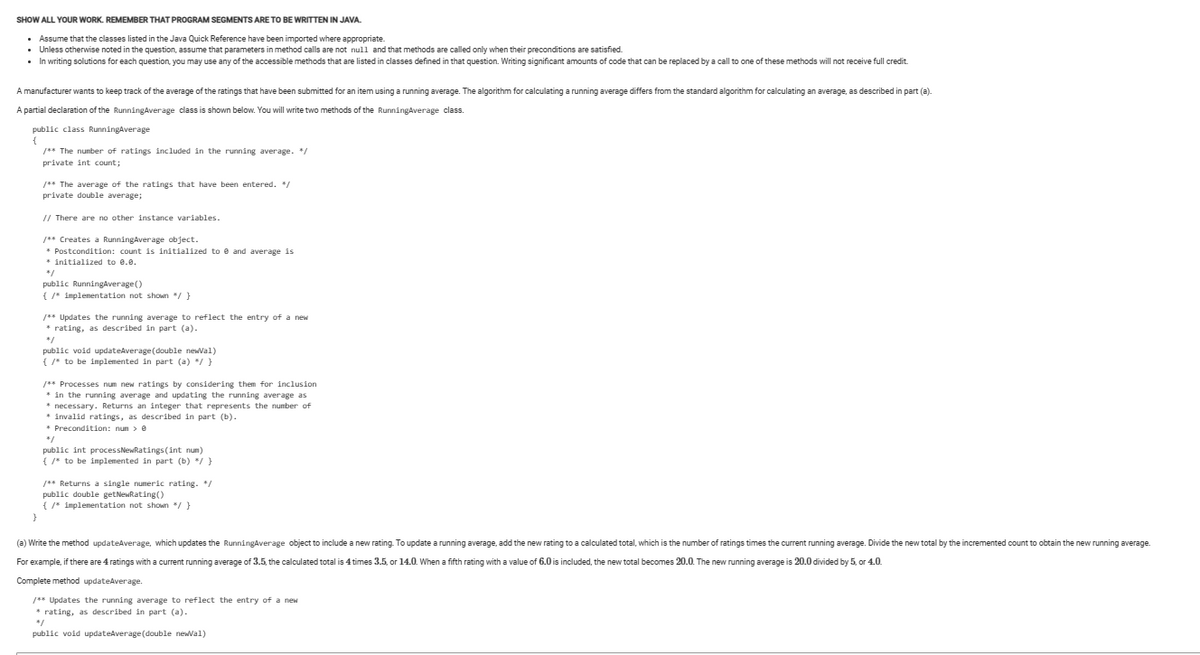
Transcribed Image Text:SHOW ALL YOUR WORK. REMEMBER THAT PROGRAM SEGMENTS ARE TO BE WRITTEN IN JAVA.
• Assume that the classes listed in the Java Quick Reference have been imported where appropriate.
• Unless otherwise noted in the question, assume that parameters in method calls are not null and that methods are called only when their preconditions are satisfied.
• In writing solutions for each question, you may use any of the accessible methods that are listed in classes defined in that question. Writing significant amounts of code that can be replaced by a call to one of these methods will not receive full credit.
A manufacturer wants to keep track of the average of the ratings that have been submitted for an item using a running average. The algorithm for calculating a running average differs from the standard algorithm for calculating an average, as described in part (a).
A partial declaration of the RunningAverage class is shown below. You will write two methods of the RunningAverage class.
public class RunningAverage
{
/** The number of ratings included in the running average. */
private int count;
/** The average of the ratings that have been entered. */
private double average;
// There are no other instance variables.
/** Creates a RunningAverage object.
* Postcondition: count is initialized to and average is
* initialized to 0.0.
*/
public RunningAverage()
{ /* implementation not shown */ }
/** Updates the running average to reflect the entry of a new
* rating, as described in part (a).
public void updateAverage (double newVal)
{ /* to be implemented in part (a) */ }
/** Processes num new ratings by considering them for inclusion
* in the running average and updating the running average as
*necessary. Returns an integer that represents the number of
* invalid ratings, as described in part (b).
* Precondition: num> @
public int processNewRatings (int num)
{ /* to be implemented in part (b) */ }
/** Returns a single numeric rating. */
public double getNewRating()
{ /* implementation not shown */ }
}
(a) Write the method updateAverage, which updates the RunningAverage object to include a new rating. To update a running average, add the new rating to a calculated total, which is the number of ratings times the current running average. Divide the new total by the incremented count to obtain the new running average.
For example, if there are 4 ratings with a current running average of 3.5, the calculated total is 4 times 3.5, or 14.0. When a fifth rating with a value of 6.0 is included, the new total becomes 20.0. The new running average is 20.0 divided by 5, or 4.0.
Complete method updateAverage.
/** Updates the running average to reflect the entry of a new
* rating, as described in part (a).
public void updateAverage (double newWal)

Transcribed Image Text:(b) Write the process NewRatings method, which considers num new ratings for inclusion in the running average. A helper method, getNewRating, which returns a single rating, has been provided for you
The running average must only be updated with ratings that are greater than or equal to zero. Ratings that are less than 0 are considered invalid and are not included in the running average.
The processNewRatings method returns the number of invalid ratings. See the table below for three examples of how calls to processNewRatings should work.
Complete method processNewRatings. Assume that updateAverage works as specified, regardless of what you wrote in part (a). You must use getNewRating and updateAverage appropriately to receive full credit.
/** Processes num new ratings by considering them for inclusion
* in the running average and updating the running average as
*necessary. Returns an integer that represents the number of
* invalid ratings, as described in part (b).
* Precondition: num > @
*/
public int processNewRatings(int num)
+-
Statement
processNewRatings (2)
processNewRatings (1)
processNewRatings (4)
Ratings
Generated
2.5, 4.5
-2.0
0.0 -2.2,
3.5, -1.5
processNewRatings
Return Value
0
1
2
Comments
Both new ratings are included in the running average.
No new ratings are included in the running average.
Two new ratings (0.0 and 3.5) are included in the running average.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

Recommended textbooks for you
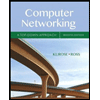
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
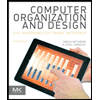
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
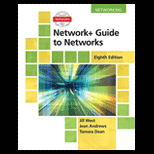
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
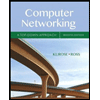
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
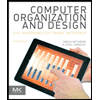
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
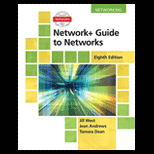
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
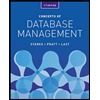
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
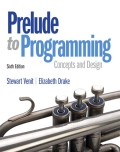
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
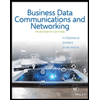
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY